SwiftUICharts
SwiftUICharts copied to clipboard
Chart Values Under 0 were shown as Transparent Color on 2.10.1
//
// ContentView.swift
// Bar Chart Test
//
// Created by zhaoxin on 2022/4/28.
//
import SwiftUI
import SwiftUICharts
struct ContentView: View {
@State var data:BarChartData?
var body: some View {
ScrollView {
VStack {
if let data = data {
BarChart(chartData: data)
.touchOverlay(chartData: data, specifier: "%0.f KGs")
.xAxisGrid(chartData: data)
.yAxisGrid(chartData: data)
.xAxisLabels(chartData: data)
.yAxisLabels(chartData: data, specifier: "%0.f KGs")
.floatingInfoBox(chartData: data)
.headerBox(chartData: data)
.padding()
HStack {
Button {
data.dataSets.dataPoints.removeFirst()
data.dataSets.dataPoints.append(getRandomPoint())
} label: {
Text("Append Item")
}
Button {
changeMaxBarNumber()
} label: {
Text("Change Max Bar Number")
}
}
} else {
ProgressView().onAppear {
let points = (1...10).map { _ in getEmptyPoint()}
let barStyle = BarStyle(barWidth: 0.5, colourFrom: .dataPoints)
data = BarChartData(dataSets: BarDataSet(dataPoints: points),
barStyle: barStyle)
}
}
}
}
.padding()
.frame(minWidth: 800, minHeight: 350)
}
private func getRandomPoint() -> BarChartDataPoint {
let i = Int.random(in: -100...100)
return BarChartDataPoint(value: Double(i), xAxisLabel: String(i),
colour: ColourStyle(colour: i > 0 ? .green : .red))
}
private func getEmptyPoint() -> BarChartDataPoint {
return BarChartDataPoint(value: 0, xAxisLabel: nil, colour: ColourStyle(colour: .green))
}
private func changeMaxBarNumber() {
let maxBarNumber = Int.random(in: 10...20)
var points = data!.dataSets.dataPoints.filter { $0.value != 0 }
let count = points.count
guard let data = data else {
fatalError()
}
if count < maxBarNumber {
let emptyPoints = (1...(maxBarNumber - count)).map { _ in getEmptyPoint() }
points.insert(contentsOf: emptyPoints, at: 0)
data.dataSets = BarDataSet(dataPoints: points)
} else {
data.dataSets = BarDataSet(dataPoints: Array(points[(count - maxBarNumber)...]))
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
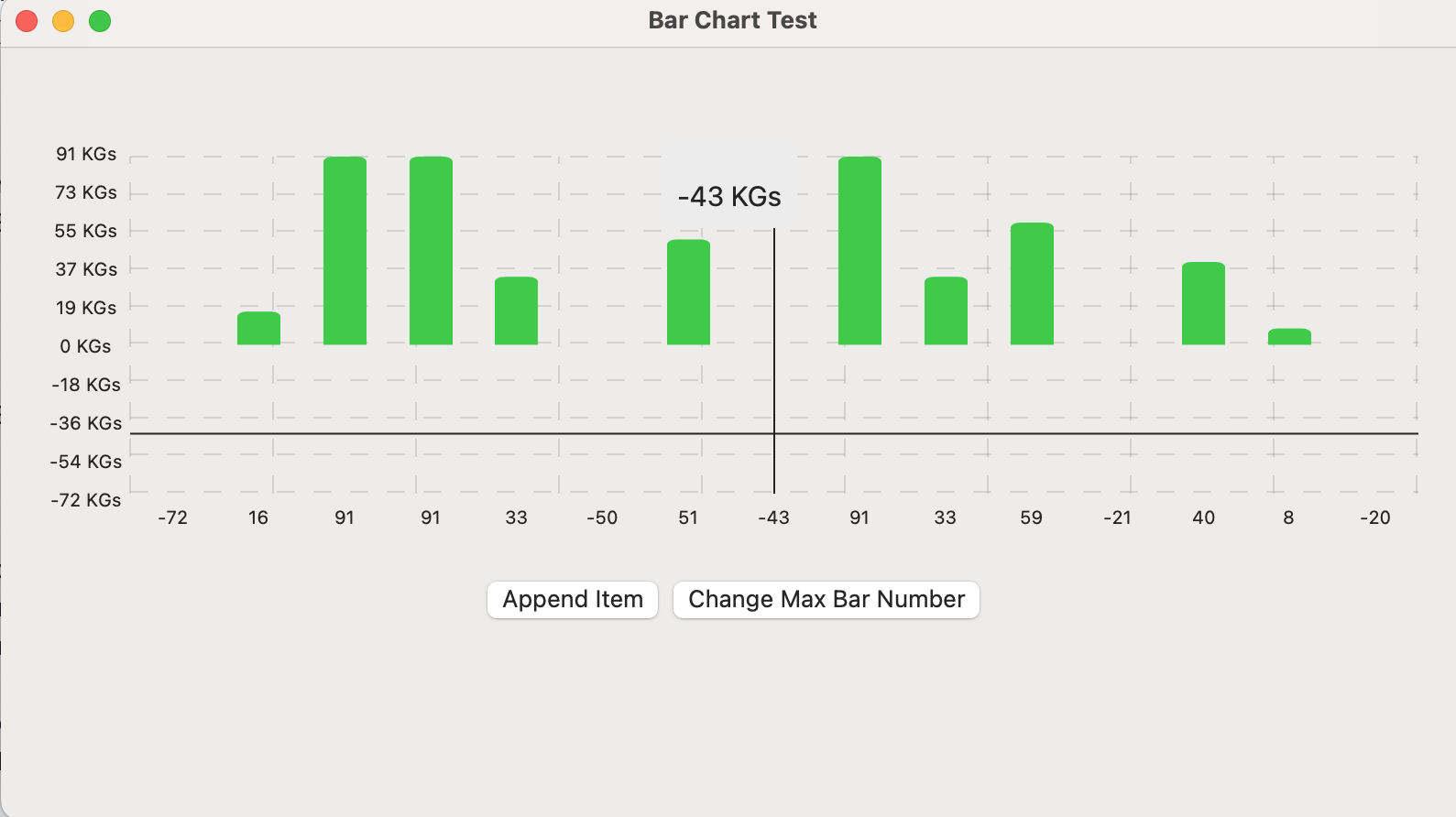
The issue is new, as 2.10.0 worked fine.
Ah, thanks for pointing out 2.10.0 works fine! I thought it was related to my reported issue: https://github.com/willdale/SwiftUICharts/issues/217
Fixed the version to 2.10.0 and solved my issue for now at least 💪
Ah, thanks for pointing out 2.10.0 works fine! I thought it was related to my reported issue: #217
Fixed the version to 2.10.0 and solved my issue for now at least 💪
Thank you for your reply and thank again for this useful framework.
For the purple warning, in my opinion, it means something that has not been officially supported. For example, if you enable one sheet inside another sheet. A purple waring showed says multiple sheets was not supported. But the app works as expected. The first sheet closes and the second sheet shows.
Thank you for your reply and thank again for this useful framework.
Don't give me the credits, I didn't create this framework! I just happened to have the same issue as you experienced