TMCStepper
TMCStepper copied to clipboard
TMC2209 configuration on start
Hi teemuatlut, you did a great job with this library!
I am having a little problem that I can't solve with your documentation (certainly because I am not much of a programmer)
I want to swap old A4988 drivers with TMC2209s and because the board that the drivers go in can't do it I want to cofigure them via UART externally. I have them connected pretty much like this but on the second (Y) driver the MS2 pin is pulled high.
And I want to set current for both of the drivers and also configure the Stallguard to be used as a homing feature.
I tried adjusting your example code but it doesn't seem to be working.
Can you please give me a hand on this? Also I am using arduino nano.
(Code)
/**
* Author Teemu Mäntykallio
*
* Plot TMC2130 or TMC2660 motor load using the stallGuard value.
* You can finetune the reading by changing the STALL_VALUE.
* This will let you control at which load the value will read 0
* and the stall flag will be triggered. This will also set pin DIAG1 high.
* A higher STALL_VALUE will make the reading less sensitive and
* a lower STALL_VALUE will make it more sensitive.
*/
#include <TMCStepper.h>
#define STALL_VALUEX 10 // [0..255]
#define STALL_VALUEY 10 // [0..255]
#define SERIAL_PORT Serial // TMC2208/TMC2224 HardwareSerial port
#define R_SENSE 0.11f // Match to your driver
// SilentStepStick series use 0.11
// UltiMachine Einsy and Archim2 boards use 0.2
// Panucatt BSD2660 uses 0.1
// Watterott TMC5160 uses 0.075
// Select your stepper driver type
TMC2209Stepper driverX(&SERIAL_PORT, R_SENSE, 0b00);
TMC2209Stepper driverY(&SERIAL_PORT, R_SENSE, 0b10);
//TMC2209Stepper driver(SW_RX, SW_TX, R_SENSE, DRIVER_ADDRESS);
void setup() {
driverX.beginSerial(115200);
driverY.beginSerial(115200);
driverX.begin();
driverX.toff(4);
driverX.blank_time(24);
driverX.rms_current(500); // mA
driverX.microsteps(16);
driverX.TCOOLTHRS(0xFFFFF); // 20bit max
driverX.semin(5);
driverX.semax(2);
driverX.sedn(0b01);
driverX.SGTHRS(STALL_VALUEX);
driverY.begin();
driverY.toff(4);
driverY.blank_time(24);
driverY.rms_current(700); // mA
driverY.microsteps(16);
driverY.TCOOLTHRS(0xFFFFF); // 20bit max
driverY.semin(5);
driverY.semax(2);
driverY.sedn(0b01);
driverY.SGTHRS(STALL_VALUEY);
}
void loop() {
}
(END of code)
Thanks a lot!
Tomas
Your first priority should be establishing working communication. You can start with something simpler like this.
#include <TMCStepper.h>
// SW Serial so we can debug the responses
TMC2209Stepper driverX(2, 3, 0.11, 0b00);
TMC2209Stepper driverY(2, 3, 0.11, 0b10);
void setup() {
Serial.begin(115200);
driverX.begin();
driverY.begin();
}
void loop() {
auto versionX = driverX.version();
auto versionY = driverY.version();
if (versionX != 0x21) {
Serial.println("Driver X communication issue");
}
if (versionY != 0x21) {
Serial.println("Driver Y communication issue");
}
delay(2000);
}
You are absolutely right of course. I uploaded your code and the communication seems fine - reports no errors. Do you have any suggestion what to do next?
If your communication works fine, then you can monitor the stallguard values and determine the proper thresholds.
Thanks for your work on this library! I've encountered similar issues as described here but the solution hasn't worked for me.
I'm using an Adafruit HUZZAH32 for my microcontroller and want use the StallGuard feature. Here are the pinouts for the HUZZAH:
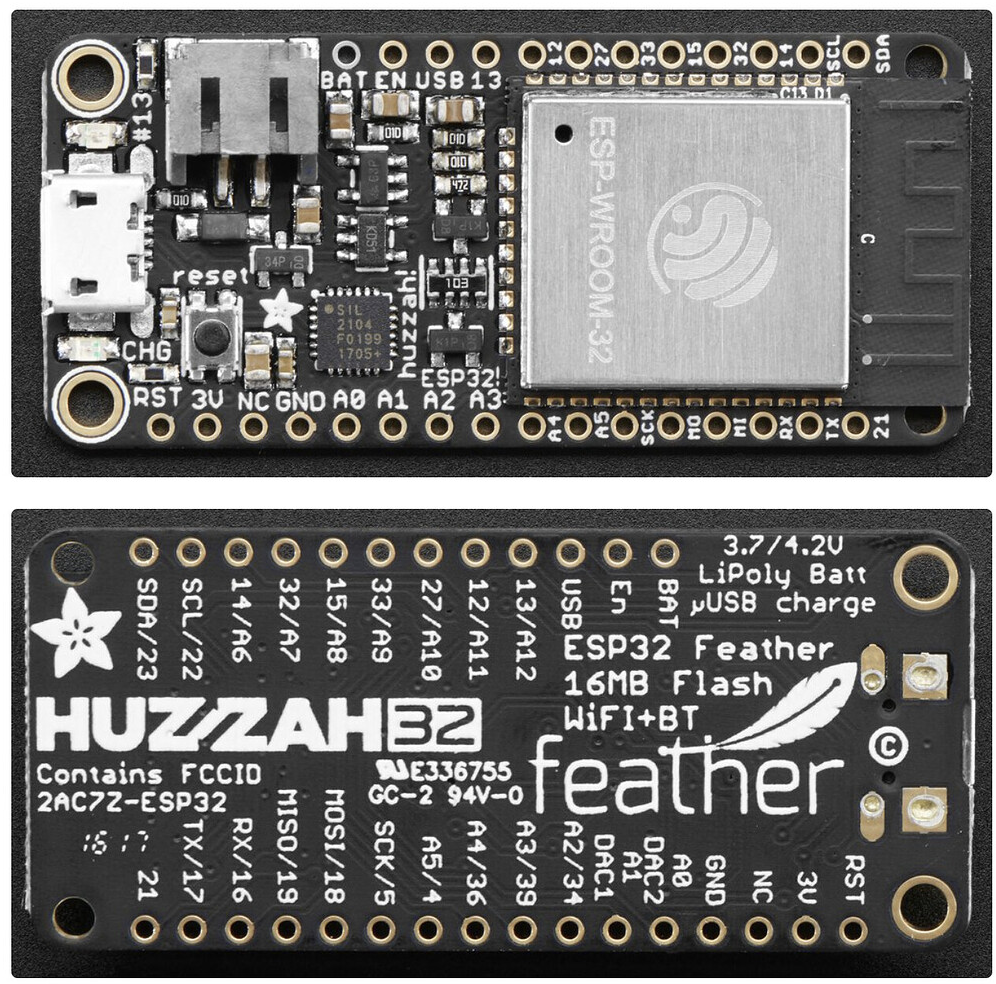
And for the TMC2209:
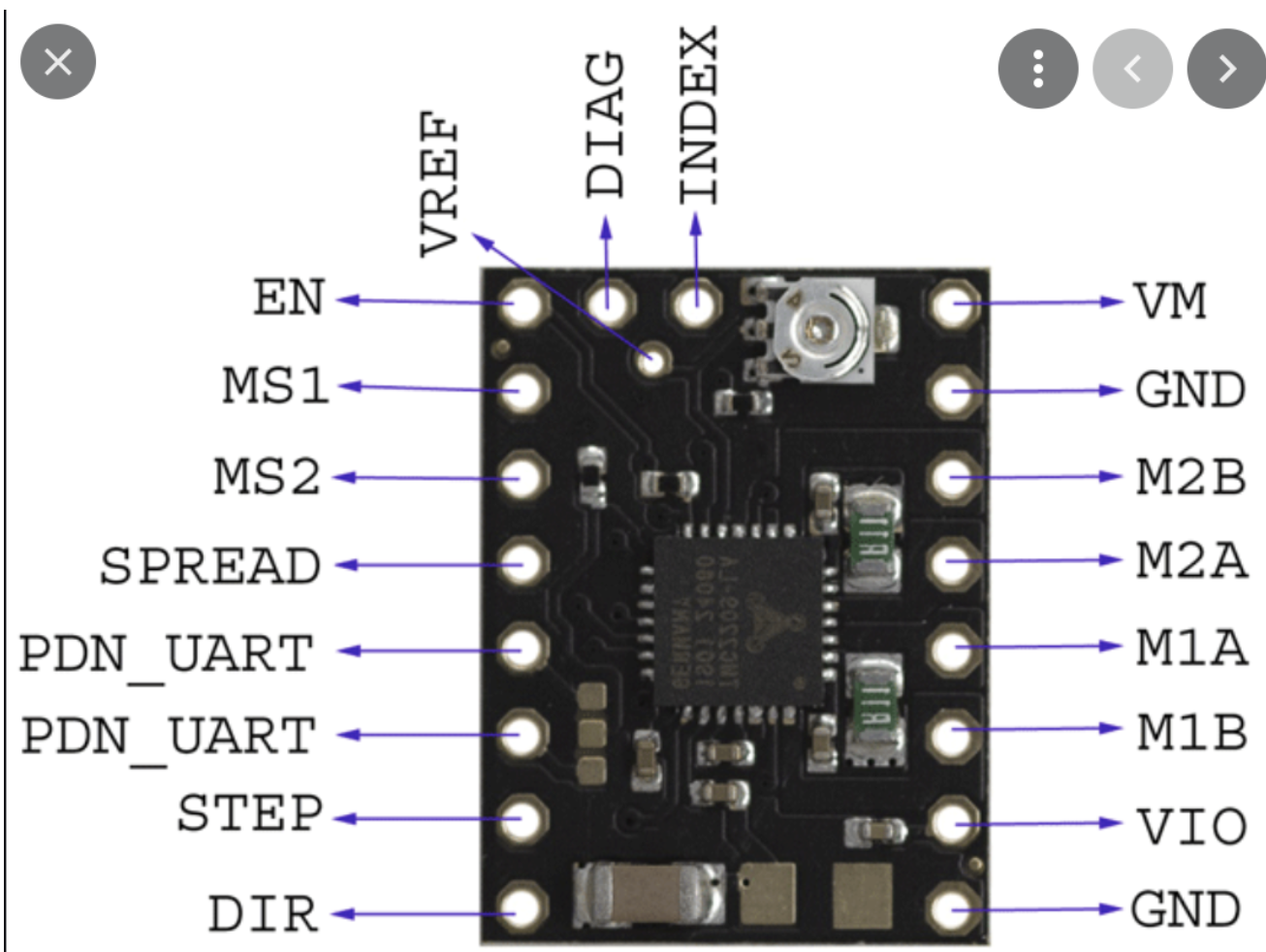
And here is how my circuit is actually wired up:
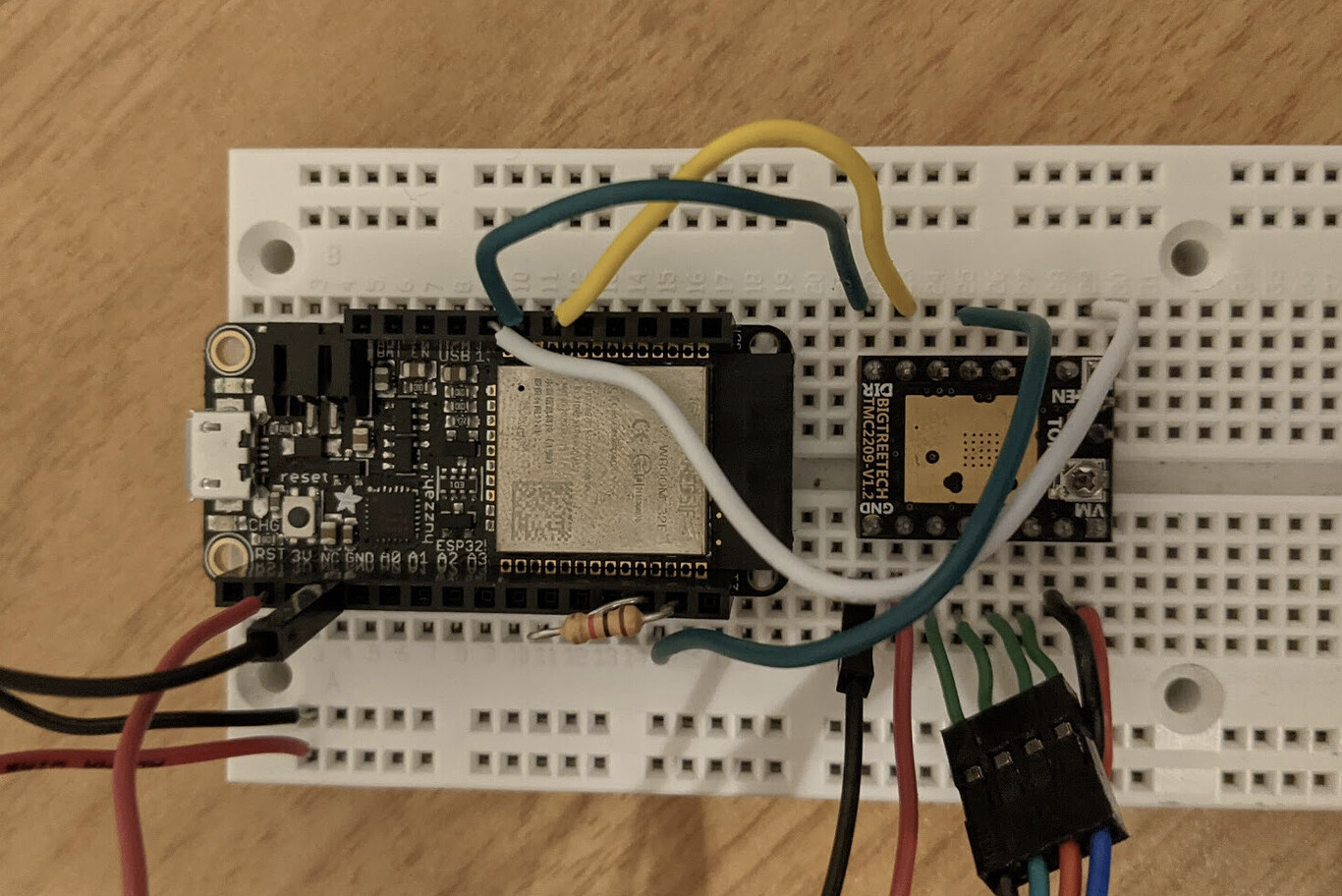
I'm trying to use UART as shown above.
Finally here is the code I'm running:
#include <TMCStepper.h>
#define EN_PIN 12 //enable (CFG6)
#define DIR_PIN 27 //direction
#define STEP_PIN 33 //step
//#define SW_RX 0 // TMC2208/TMC2224 SoftwareSerial receive pin
//#define SW_TX 1 // TMC2208/TMC2224 SoftwareSerial transmit pin
#define SERIAL_PORT Serial1 // TMC2208/TMC2224 HardwareSerial port
#define DRIVER_ADDRESS 0b00 // TMC2209 Driver address according to MS1 and MS2
#define R_SENSE 0.11f
TMC2209Stepper driver(&SERIAL_PORT, R_SENSE, DRIVER_ADDRESS);
//TMC2209Stepper driver(SW_RX, SW_TX, R_SENSE, DRIVER_ADDRESS);
void setup()
{
//set pin modes
pinMode(EN_PIN, OUTPUT);
digitalWrite(EN_PIN, HIGH); //deactivate driver (LOW active)
pinMode(DIR_PIN, OUTPUT);
digitalWrite(DIR_PIN, LOW); //LOW or HIGH
pinMode(STEP_PIN, OUTPUT);
digitalWrite(STEP_PIN, LOW);
digitalWrite(EN_PIN, LOW); //activate driver
Serial.begin(115200);
while (!Serial) {
; // wait for serial port to connect. Needed for Native USB only
}
SERIAL_PORT.begin(115200);
}
void loop()
{
// auto versionX = driver.version();
// if (versionX != 0x21) {
// Serial.println("Driver X communication issue");
// }
Serial.println(driver.SG_RESULT(), DEC);
//make steps
digitalWrite(DIR_PIN, HIGH); // Turn left
for (int i = 0; i < 200; i++) {
digitalWrite(STEP_PIN, HIGH);
delay(1);
digitalWrite(STEP_PIN, LOW);
delay(1);
}
delay(100);
digitalWrite(DIR_PIN, LOW); // Turn left
for (int i = 0; i < 600; i++) {
digitalWrite(STEP_PIN, HIGH);
delay(1);
digitalWrite(STEP_PIN, LOW);
delay(1);
}
delay(100);
}
I can't see where I deviate from the solution described above and am starting to feel pretty stuck on this one. When I do the version check I get the error message, and SG_RESULT just prints 0.
same problem, same error with the driver not even connect
Something to try that worked for me: I swapped the pin which is directly connected to the uart pin, the data sheet for my step stick showed it connected to Tx but it worked when I connected it to Rx with the 1k resistor to Tx. Weirdly I could set microsteps and current but couldn't read the SG_RESULT before the pin swap.
I still have the problem :/