react-ace
react-ace copied to clipboard
Question: Documentation on AutoComplete
I can't find any details on AutoComplete, the difference between Basic and Live, how to add new AutoComplete rules, etc. I'm not clear if this is part of brace or something done externally. Can anyone point me in the right direction?
@Eric24 they are part of ace.js https://github.com/ajaxorg/ace/wiki/How-to-enable-Autocomplete-in-the-Ace-editor
by including the language_tools
like in this example https://github.com/ajaxorg/ace/wiki/How-to-enable-Autocomplete-in-the-Ace-editor you can enable them. As far as adding custom completers there is probably a way to do it by accessing the editor. When the editor has autocomplete turned on it has completers
available which you can push something like the rhymecompleter in this example http://plnkr.co/edit/6MVntVmXYUbjR0DI82Cr?p=preview from ace.js
I needed an html code editor with basic autocomplete, live autocomplete and snippet suggestion and I have managed to achieve my goal in this way:
Packages used
"react-ace": "^5.8.0",
"react": "^16.2.0",
Imports
import AceEditor from 'react-ace';
import 'brace/mode/html';
import 'brace/theme/xcode';
import 'brace/snippets/html';
import 'brace/ext/language_tools';
Render
<AceEditor
mode="html"
theme="xcode"
name="content"
onChange={this.onContentChange}
showPrintMargin={true}
showGutter={true}
highlightActiveLine={true}
value={form.content}
editorProps={{$blockScrolling: Infinity}}
setOptions={{
enableBasicAutocompletion: true,
enableLiveAutocompletion: true,
enableSnippets: true,
showLineNumbers: true,
tabSize: 2
}}
/>
Output
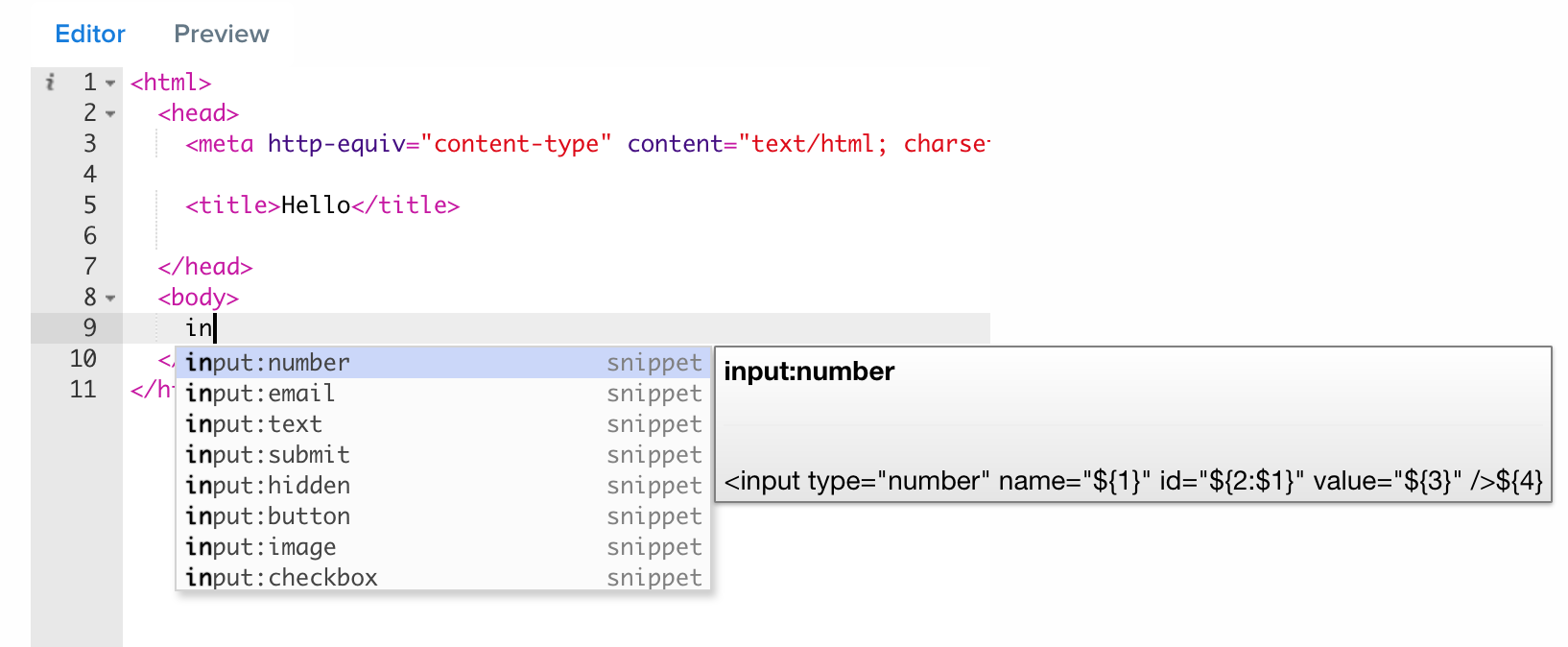
I believe, for other languages, by following this way, a similar output can be achieved.
Hope, it helps.
@anindya-dhruba How to write a custom mode? Your example shows clearly about adding mode which is in the brace, but what if I want to add my own?
@oakland, I am not the author of this repo, but I guess, you need to write one yourself and import that instead of the built-in one. I don't know how to write custom mode. The author @securingsincity might help you in this case.
Thanks.
I still cannot find any docs about what is live and basic?
@Obiwarn as far as I understand, "basic" is the completion mode that has to be manually triggered by pressing ctrl+space, and "live" is the one that displays suggestions as you type.
Hi there! I'm trying to turn on auto complete and add my own, custom variables but I'm running into issues. I'm trying to combine code from these two sources: http://plnkr.co/edit/PNvznZCXwr8nJAHwhxA2?p=preview
https://github.com/securingsincity/react-ace/blob/master/docs/FAQ.md#how-do-i-add-language-snippets
Here's basically what I've got so far:
Imports:
import AceEditor from 'react-ace';
import 'brace/mode/java';
import 'brace/theme/textmate';
import CustomMode from "./customMode.js"; //This is because I'm making my own mode, I think you can ignore it
import "brace/ext/language_tools";
Render:
//The text editor:
renderWidget(args) {
Object.assign(args, {
ref: "aceEditor",
mode: "java",
fontSize: 16,
theme: "textmate",
showPrintMargin: false,
showGutter: true,
readOnly: false,
wrapEnabled: true,
editorProps: {$blockScrolling: true},
setOptions: {
enableBasicAutocompletion: true,
enableLiveAutocompletion: true,
enableSnippets: true,
showLineNumbers: true,
tabSize: 2,
}
});
return <AceEditor {...args}/>;
}
Other:
componentDidMount() {
const customMode = new CustomMode(); //Has to do with custom mode
this.refs.aceEditor.editor.getSession().setMode(customMode); //Has to do with custom mode
language_tools.addCompleter(staticWordCompleter);
}
I'm getting a type error: language_tools is not defined
Any help would be appreciated!
Hi there! I'm trying to turn on auto complete and add my own, custom variables but I'm running into issues. I'm trying to combine code from these two sources: http://plnkr.co/edit/PNvznZCXwr8nJAHwhxA2?p=preview
https://github.com/securingsincity/react-ace/blob/master/docs/FAQ.md#how-do-i-add-language-snippets
Here's basically what I've got so far:
Imports:
import AceEditor from 'react-ace'; import 'brace/mode/java'; import 'brace/theme/textmate'; import CustomMode from "./customMode.js"; //This is because I'm making my own mode, I think you can ignore it import "brace/ext/language_tools";
Render:
//The text editor: renderWidget(args) { Object.assign(args, { ref: "aceEditor", mode: "java", fontSize: 16, theme: "textmate", showPrintMargin: false, showGutter: true, readOnly: false, wrapEnabled: true, editorProps: {$blockScrolling: true}, setOptions: { enableBasicAutocompletion: true, enableLiveAutocompletion: true, enableSnippets: true, showLineNumbers: true, tabSize: 2, } }); return <AceEditor {...args}/>; }
Other:
componentDidMount() { const customMode = new CustomMode(); //Has to do with custom mode this.refs.aceEditor.editor.getSession().setMode(customMode); //Has to do with custom mode language_tools.addCompleter(staticWordCompleter); }
I'm getting a type error:
language_tools is not defined
Any help would be appreciated! Give up the old iron, it does not support custom!
@anindya-dhruba worked like a charm <3
forgot language tools, thanks man
Note from the react-ace Readme:
NOTE FOR VERSION 8! : We have stopped support for Brace and now use Ace-builds. Please read the documentation on how to migrate. Examples are being updated.
下面的方法可以添加自定义的代码补全。
import { addCompleter } from 'ace-builds/src-noconflict/ext-language_tools';
componentDidMount() {
addCompleter({
getCompletions: function(editor, session, pos, prefix, callback) {
callback(null, [
{
name: 'test',
value: 'test',
caption: 'test',
meta: 'local',
score: 1000,
},
]);
},
});
}
You can also do something like:
const customCompleter = (editor, session, pos, prefix, cb) => {
cb(null, [...])
}
<AceEditor
...
setOptions={{
...,
enableBasicAutocompletion: [customCompleter],
enableLiveAutocompletion: true
}}
/>
It worked for me in my CRA app(with Typescript).
import ace, {Ace} from 'ace-builds';
import React from 'react';
import AceEditor from 'react-ace';
import 'ace-builds/src-noconflict/mode-sql';
import 'ace-builds/src-noconflict/theme-github';
import 'ace-builds/src-noconflict/ext-language_tools';
const Editor: React.FC = () => {
const name = 'unique name';
React.useEffect(() => {
const langTools = ace.require('ace/ext/language_tools');
// data stub:
const sqlTables = [
{ name: 'users', description: 'Users in the system' },
{ name: 'userGroups', description: 'User groups to which users belong' },
{ name: 'customers', description: 'Customer entries' },
{ name: 'companies', description: 'Legal entities of customers' },
{ name: 'loginLog', description: 'Log entries for user log-ins' },
{ name: 'products', description: 'Products offered in the system' },
{ name: 'productCategories', description: 'Different product categories' },
];
const sqlTablesCompleter = {
getCompletions: (
editor: Ace.Editor,
session: Ace.EditSession,
pos: Ace.Point,
prefix: string,
callback: Ace.CompleterCallback
): void => {
callback(
null,
sqlTables.map((table) => ({
caption: `${table.name}: ${table.description}`,
value: table.name,
meta: 'Table',
} as Ace.Completion))
);
},
};
langTools.addCompleter(sqlTablesCompleter);
}, []);
return (
<AceEditor
mode='sql'
theme='github'
name={name}
editorProps={{ $blockScrolling: true }}
setOptions={{
enableBasicAutocompletion: true,
enableLiveAutocompletion: true,
}}
/>
);
};
export default Editor;
help sources
- https://gist.github.com/yuvalherziger/aa48782568c6914b55066d290ff88360
- https://github.com/ajaxorg/ace-builds/blob/a4103cb0a672a4fec9a16fc344f3116b51d6bcda/ace.d.ts
- https://stackoverflow.com/a/46501877
Is there any way to accomplish an async auto-completer? I have thousands of hard-coded tokens/keywords and I would like to dynamically fetch autocomplete suggestions as the user types.
Guys, I need to use it differently. The comment from @sheep0729 and @kanghyojun helped me a lot, but I realized that using:
...
} as Ace.Completion))
);
},
};
langTools.addCompleter(staticWordCompleter);
}, []);
// returned: addCompleter is not defined
At first, my const langTools was not being recognized, even though I used:
const langTools = ace.require('ace/ext/language_tools');
so I used it directly in the import:
import { addCompleter } from "ace-builds/src-noconflict/ext-language_tools";
and replaces langTools.addCompleter() with addCompleter() directly:
...
} as Ace.Completion))
);
},
};
addCompleter(staticWordCompleter)
}, []);
That way it worked perfectly.