redux-persist
redux-persist copied to clipboard
persist/REHYDRATE payload and err is undefined
I use redux-persist 5.7.2 with react-starter-kit.
I tried 5.6.12, it doesn't run persist/REHYDRATE.
configureStore.js
const authPersistConfig = { key: 'root', storage: storageSession, };
export default function configureStore(initialState, helpersConfig) {
const helpers = createHelpers(helpersConfig);
const middleware = [thunk.withExtraArgument(helpers)];
let enhancer;
if (__DEV__) {
middleware.push(createLogger());
let devToolsExtension = f => f;
if (process.env.BROWSER && window.devToolsExtension) {
devToolsExtension = window.devToolsExtension(); }
enhancer = compose(applyMiddleware(...middleware), devToolsExtension);
} else {
enhancer = applyMiddleware(...middleware);}
const rootReducer = createRootReducer();
const persistedReducer = persistReducer(authPersistConfig, rootReducer);
const store = createStore(persistedReducer, initialState, enhancer);
if (__DEV__ && module.hot) {
module.hot.accept('../reducers', () =>
if you change reducers back to normal rootReducer.
store.replaceReducer(require('../reducers').default()),
);}
const persistor = persistStore(store);
return { store, persistor };
}
Do i set it wrong?
part of client.js
const { store, persistor } = configureStore(window.App.state, { apolloClient, fetch, history, });
const renderReactApp = isInitialRender ? ReactDOM.hydrate : ReactDOM.render;
appInstance = renderReactApp(
<App context={context}>
<PersistGate loading={null} persistor={persistor}>
{route.component}
</PersistGate>
</App>,container,
() => {
if (isInitialRender) {
const elem = document.getElementById('css');
if (elem) elem.parentNode.removeChild(elem);
return;
}
The PersistGate in server.js is same as client.js
are you using android? react native AsyncStorage has a bug with android when the debugger is open causing this to happen. As you can see the persist is timing out (check the error on the REHYDRATE action).
The only workaround for this is to use a different storage engine: https://github.com/rt2zz/redux-persist#storage-engines
No, i am writing website using react
hm, then possibly a bug with storageSession
? You could try using localForage (https://github.com/localForage/localForage) and see if that works?
[13:12:54] Finished 'server' compilation after 15634 ms
[13:12:54] Launching server...
redux-persist failed to create sync storage. falling back to memory storage.
...
persist/REHYDRATE: { type: 'persist/REHYDRATE', payload: undefined, err: undefined, key: 'root' }
This is the error message when i yarn start my project.
ah interesting, so session storage is failing - that explains the behavior. We need to figure out why.
That means this line is failing: https://github.com/rt2zz/redux-persist/blob/master/src/storage/getStorage.js#L35
are you running this in a standard browser? Is it a private browsing session perhaps?
I run in the latest chrome and tried localstorage & LOCALFORAGE, but also fail. I move PersistGate from client.js to App.js and create persist reducer to show all persist action.
export default function persist(state = null, action) {
console.warn(action);
switch (action.type) {
case 'persist/REHYDRATE':
return {
...state,
persistedState: action.payload,
};
default:
return state;}}
This is the result.
I can get the previous result (user), before execute the persist/REHYDRATE.
After executed the persist/REHYDRATE, the result was set to null (user).
Ok I see REHYDRATE is being called twice, can you make sure you are on the latest version of redux persist? I believe 5.7.x may have had a bug causing this
yes, the redux persist version in my project is 5.8.0. however, the result is same as 5.7.2.
I have to say I've been stuck in this problem for a while now and it was my fault. I forgot to return an action on an Epic. I hope this helps anyone
@challme28 could you explain a little more? maybe a code snippet?
@tonykawa you can use ``` code in here ```
for multi-line code fragments. It gets rendered nicely, for example
// configureStore.js
const authPersistConfig = {
key: 'root',
storage: storageSession,
};
export default function configureStore(initialState, helpersConfig) {
const helpers = createHelpers(helpersConfig);
const middleware = [thunk.withExtraArgument(helpers)];
let enhancer;
if (__DEV__) {
middleware.push(createLogger());
let devToolsExtension = f => f;
if (process.env.BROWSER && window.devToolsExtension) {
devToolsExtension = window.devToolsExtension();
}
enhancer = compose(applyMiddleware(...middleware), devToolsExtension);
} else {
enhancer = applyMiddleware(...middleware);
}
const rootReducer = createRootReducer();
const persistedReducer = persistReducer(authPersistConfig, rootReducer);
const store = createStore(persistedReducer, initialState, enhancer);
if (__DEV__ && module.hot) {
module.hot.accept('../reducers', () =>
// if you change reducers back to normal
rootReducer.store.replaceReducer(require('../reducers').default()),
);
}
const persistor = persistStore(store);
return {
store,
persistor
};
}
Markdown:
@tonykawa I had it like this:
export function rehydrateEpic(action$: ActionsObservable<RehydrateAction>) {
return action$.ofType(REHYDRATE)
.mergeMap(({ payload }) => {
const { authenticated } = payload.auth;
if (authenticated) {
return Observable.of(HomeAction)
}
});
}
If the user is not authenticated it does not return anything so Rehydrated is called again
So i had to add a else return Observable.of(notAuthenticated());
. This stopped the second call on REHYDRATE
@rt2zz is dispatching REHYDRATE
twice a bug?
only happening when the storage is empty, so there's nothing to hydrate from?
@rt2zz tried the different storage engines (localStorage, sessionStorage, localForage), but all of them are showing the same error:
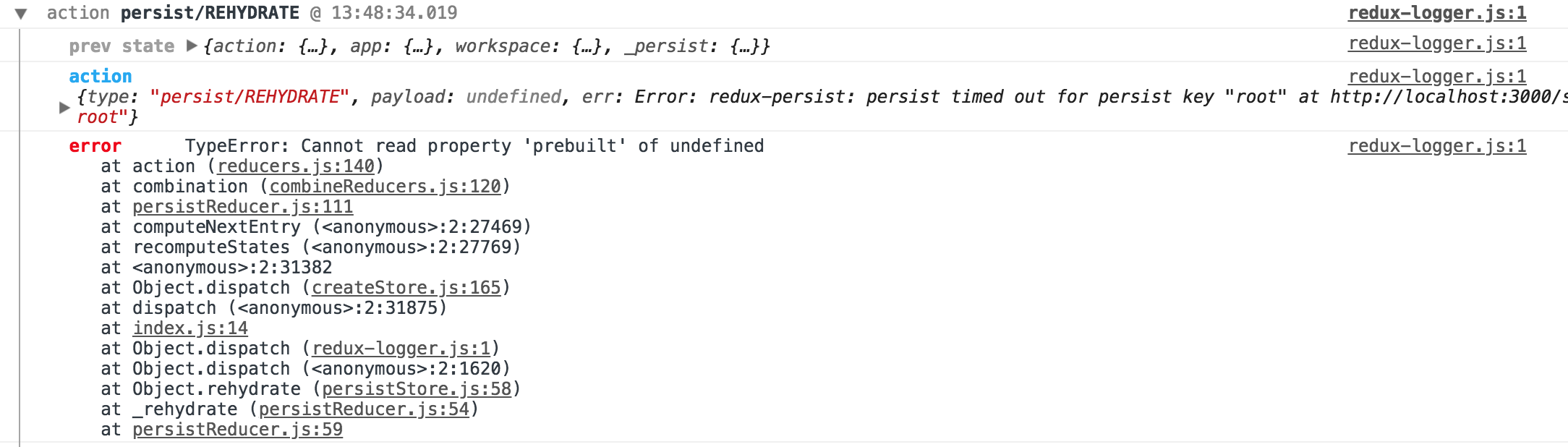
Temporary workaround is to check if action.payload
is defined
export const app = (state = appDefaultState, action) => {
switch (action.type) {
case REHYDRATE:
if (action.payload) { // <- I guess this works, but it's kinda ugly
return {
...state,
user: action.payload.user,
};
} else {
return state;
}
...
@atav32 you are seeing persist dispatched twice when there is no prior storage? That is definitely a bug, but I dont see how that can happen as we only allow rehydrate to be called once (per persistReducer): https://github.com/rt2zz/redux-persist/blob/master/src/persistReducer.js#L71-L86
I have the same issue.
Using version 5.9.1 I get an undefined payload if there's nothing in localstorage (same with localForage).
@mattmcdonald-uk I just ended up doing this, https://egghead.io/lessons/javascript-redux-persisting-the-state-to-the-local-storage
@rt2zz I'm still still getting these issues, although they only seem to affect random devices. In my office there's 10 of us using my app, and only 2 people seem to get this.
In my case, rehydrate only happens once, but payload and err are both empty. Is it perhaps a memory issue?
put on code in a lazy component (200-300 ms) and your project will work again.
Example:
<LazyComponent> <Provider>...</Provider> </LazyComponent>
@rt2zz I'm still still getting these issues, although they only seem to affect random devices. In my office there's 10 of us using my app, and only 2 people seem to get this.
In my case, rehydrate only happens once, but payload and err are both empty. Is it perhaps a memory issue?
same issue, but in my case, it happens when hermes engine is enabled
I experienced very similar issue caused by my store. Make sure you always return something from the store. Even if you don't plan to change anything.
I was missing the default branch for following switch.
case USER_LOGIN:
return {
...state,
...action.payload
}
case USER_LOGOUT:
return {
...state,
user: undefined
}
default:
return state
@rt2zz I'm still still getting these issues, although they only seem to affect random devices. In my office there's 10 of us using my app, and only 2 people seem to get this. In my case, rehydrate only happens once, but payload and err are both empty. Is it perhaps a memory issue?
same issue, but in my case, it happens when hermes engine is enabled
@DeVoresyah hello, have you figured out why we have timeout error after Hermes is enabled?