yaml-language-server
yaml-language-server copied to clipboard
Problem with trigger characters and autocompletion
After we have set the trigger characters up there seems to be a few issues with the trigger characters and autocompletion. Sometimes you'll find the trigger characters activate autocompletion one space too early which causes invalid autocompletion elements. It might have to do with something in https://github.com/redhat-developer/yaml-language-server/blob/master/src/server.ts#L503
In order to test the issue you can set the trigger character to " " and then you can set the schema to be kubernetes then have
apiVersion: v1
metadata:
name: test
and click space on the very last row. When you click space you'll notice that a few of the suggestions are not correct and autocompleting them results in errors.
Trigger characters has been reverted as a result until this is resolved.
What kind of logic would be needed to make this robust enough to restore the trigger characters? Could the indentation level be validated in getPropertyCompletions? I'm not familiar enough with YAML to know whether there are more complex considerations.
I think at this point the problem is that when performing auto completion it will sometimes show bogus results that when you auto complete them it will result in errors.
I.e. if you are using http://json.schemastore.org/composer as your set schema and you have
abandoned: true
in your document if you try and autocomplete 4 spaces after true on that same line it will pop up with auto completion items. If you use those auto completion items you will get errors in your document. The auto completion shouldn't be showing up on that same line. Once that gets fixed trigger characters can be re-added.
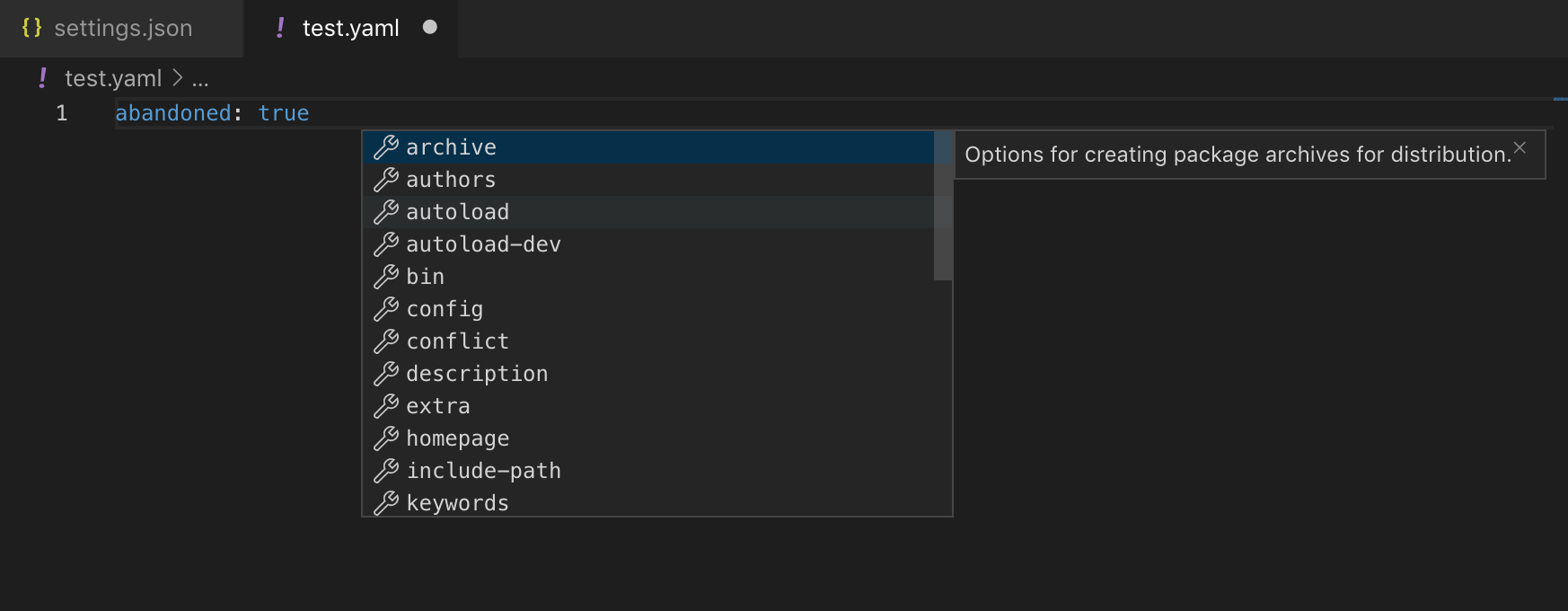
Also for some reason if you auto complete on a different line after true with a few spaces indented it tries to auto complete for values true/false
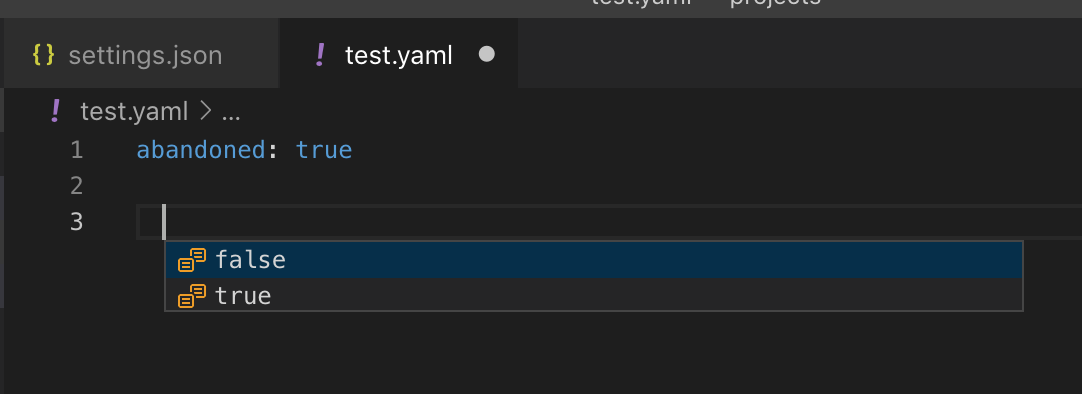
My team is building an extension for an internal tool. I have added an additional CompletionProvider
that essentially provides the trigger character functionality that this issue refers to by a) subscribing to trigger characters and b) calling the 'vscode.executeCompletionItemProvider'
command. But before simply calling that command, it does do some logic that addresses at least the first screenshot you provided. Perhaps this offers a helpful start:
const schemaCompletionItemProvider: vscode.CompletionItemProvider = {
async provideCompletionItems(document, position, token, context) {
if (!context.triggerCharacter) return [];
// Only trying to augment autocompletion of keys, so don't do anything if the line already has a colon
if (document.lineAt(position).text.includes(":")) return [];
const editor = vscode.window.activeTextEditor;
if (!editor) return [];
// Gets the text of the current line before the given position
const textBeforePosition = getTextBeforePosition(document, position, true);
// Provide completions if the preceding text only contains whitespace and hyphens
if (!/^[-\s]*$/.test(textBeforePosition)) return [];
// Only provide completions if we are at a tab stop, not including the very start of the line
const tabSize = editor.options.tabSize as number;
const len = textBeforePosition.length
if (!len && len % tabSize) return []
return vscode.commands.executeCommand(
"vscode.executeCompletionItemProvider",
document.uri,
position
) as Promise<vscode.CompletionList>;
}
};
vscode.languages.registerCompletionItemProvider(
"yaml", schemaCompletionItemProvider, " ", "\n"
);
Regarding the second screenshot, is it valid YAML to start that line indented? If not, that's a situation where I wonder whether logic around "is this a valid place for some text?" in getPropertyCompletions
could be a potential solution.
Thanks! That code will be very helpful! I don't think in the second screenshot it would be valid yaml so yeah its probably something that we could check in getPropertyCompletions