DinkToPdf
DinkToPdf copied to clipboard
Unable to load DLL 'libwkhtmltox' or one of its dependencies: The specified module could not be found.
When building locally I keep getting Unable to load DLL 'libwkhtmltox' or one of its dependencies: The specified module could not be found.
My function looks like this.
public class Html2Pdf
{
[FunctionName("Html2Pdf")]
public async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
var converter = new SynchronizedConverter(new PdfTools());
var doc = new HtmlToPdfDocument()
{
GlobalSettings = {
ColorMode = ColorMode.Color,
Orientation = Orientation.Landscape,
PaperSize = PaperKind.A4Plus,
},
Objects = {
new ObjectSettings() {
PagesCount = true,
HtmlContent = @"Lorem ipsum dolor sit amet, consectetur adipiscing elit. In consectetur mauris eget ultrices iaculis. Ut odio viverra, molestie lectus nec, venenatis turpis.",
WebSettings = { DefaultEncoding = "utf-8" },
HeaderSettings = { FontSize = 9, Right = "Page [page] of [toPage]", Line = true, Spacing = 2.812 }
}
}
};
try
{
converter.Convert(doc);
}
catch (Exception e)
{
Console.WriteLine(e);
throw;
}
return new OkObjectResult("ok");
}
}
In startup.cs
namespace Html2Pdf
{
public class Startup : FunctionsStartup
{
public override void Configure(IFunctionsHostBuilder builder)
{
if (builder == null)
throw new ArgumentException(nameof(builder));
}
public void ConfigureServices(IServiceCollection services)
{
// Add converter to DI
services.AddSingleton(typeof(IConverter), new SynchronizedConverter(new PdfTools()));
}
}
}
I got same error.
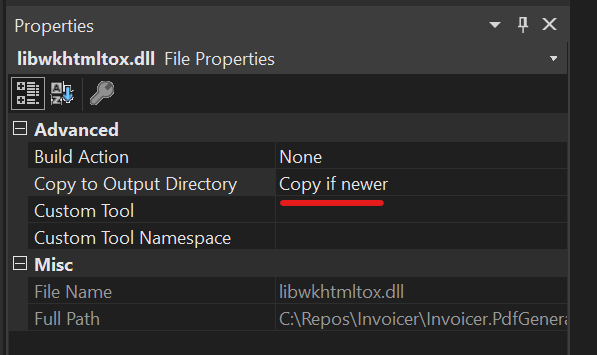
Check the properties of the libwkhtmltox files. If 'Copy to Put Directory' is set to 'None', set it to 'Copy always' or 'Copy is newer'
Try adding this package: https://www.nuget.org/packages/DinkToPdfCopyDependencies
Add library files to directory wkhtmltox
in project then:
Add to *.csproj
<ItemGroup>
<Folder Include="wkhtmltox" />
</ItemGroup>
<Target Name="CopyRequiredLibrary" AfterTargets="AfterBuild">
<Copy SourceFiles="wkhtmltox/libwkhtmltox.dll" DestinationFolder="$(OutDir)" />
<Copy SourceFiles="wkhtmltox/libwkhtmltox.dylib" DestinationFolder="$(OutDir)" />
<Copy SourceFiles="wkhtmltox/libwkhtmltox.so" DestinationFolder="$(OutDir)" />
</Target>
<Target Name="CopyRequiredLibraryOnPublish" AfterTargets="Publish">
<Copy SourceFiles="wkhtmltox/libwkhtmltox.dll" DestinationFolder="$(PublishDir)" />
<Copy SourceFiles="wkhtmltox/libwkhtmltox.dylib" DestinationFolder="$(PublishDir)" />
<Copy SourceFiles="wkhtmltox/libwkhtmltox.so" DestinationFolder="$(PublishDir)" />
</Target>
Now all files will be added to proper location
I am facing the exact same issue :
My code:
Console.WriteLine("Starting Pechkin"); var converter = new SynchronizedConverter(new PdfTools()); Console.WriteLine("Converter");
var doc = new HtmlToPdfDocument()
{
GlobalSettings = {
ColorMode = ColorMode.Color,
Orientation = Orientation.Landscape,
PaperSize = PaperKind.A4Plus,
},
Objects = {
new ObjectSettings() {
PagesCount = true,
HtmlContent = @"<!DOCTYPE html> <html> <body> <h1>My First Heading</h1> <p>My first paragraph. ا </p> </body> </html>",
WebSettings = { DefaultEncoding = "utf-8" },
HeaderSettings = { FontSize = 9, Right = "Page [page] of [toPage]", Line = true, Spacing = 2.812 }
}
}
};
Console.WriteLine("Document");
byte[] pdf = converter.Convert(doc);
Console.WriteLine("Converted");
Console.WriteLine("Done with bytes length: " + pdf.Length);
I tried adding the above targets to my .csproj but it says - Could not copy the file "wkhtmltox/libwkhtmltox.dll" because it was not found.
Do we need to install wkhtmltopdf?
Quick question, the library says it's a wrapper over wkhtltopdf, if we end up installing/adding wkhtltopdf dlls ourselves then how come this library is providing any benefit? At the end of the day, I want to simply deploy my code to a container and don't want to get into those complexities of adding/managing wkhtmltopdf dlls.
Any help on this?