proxy
proxy copied to clipboard
MacOS g++-12 compile default linker error and mold linker with bus error
When I was trying to compile the project in MacOS 13 with default linker, it outcomes
When I switched linker to mold, the error becomes runtime:
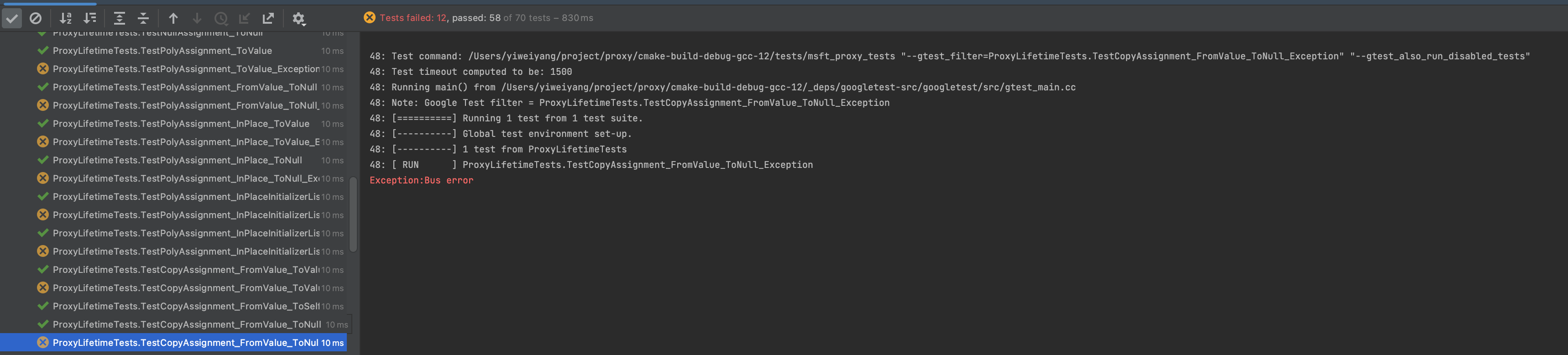
And I test the mvp with
#include <iostream>
#include <map>
#include <string>
#include <cassert>
#include <vector>
#include <proxy/proxy.h>
enum class LifetimeOperationType {
kNone,
kValueConstruction,
kInitializerListConstruction,
kCopyConstruction,
kMoveConstruction,
kDestruction
};
struct LifetimeOperation {
LifetimeOperation(int id, LifetimeOperationType type) : id_(id), type_(type) {}
bool operator==(const LifetimeOperation& rhs) const
{ return id_ == rhs.id_ && type_ == rhs.type_; }
int id_;
LifetimeOperationType type_;
};
struct ConstructionFailure : std::exception {
ConstructionFailure(LifetimeOperationType type) : type_(type) {}
LifetimeOperationType type_;
};
class LifetimeTracker {
public:
LifetimeTracker() = default;
LifetimeTracker(const LifetimeTracker&) = delete;
class Session {
public:
Session(LifetimeTracker* host)
: id_(host->AllocateId(LifetimeOperationType::kValueConstruction)),
host_(host) {}
Session(std::initializer_list<int>, LifetimeTracker* host)
: id_(host->AllocateId(LifetimeOperationType::kInitializerListConstruction)),
host_(host) {}
Session(const Session& rhs)
: id_(rhs.host_->AllocateId(LifetimeOperationType::kCopyConstruction)),
host_(rhs.host_) {}
Session(Session&& rhs) noexcept :
id_(rhs.host_->AllocateId(LifetimeOperationType::kMoveConstruction)),
host_(rhs.host_) {}
~Session() { host_->ops_.emplace_back(id_, LifetimeOperationType::kDestruction); }
Session& operator*() { return *this; }
friend std::string to_string(const Session& self) { return "Session " + std::to_string(self.id_); }
private:
int id_;
LifetimeTracker* const host_;
};
const std::vector<LifetimeOperation>& GetOperations() const { return ops_; }
void ThrowOnNextConstruction() { throw_on_next_construction_ = true; }
private:
int AllocateId(LifetimeOperationType operation_type) {
if (throw_on_next_construction_) {
throw_on_next_construction_ = false;
throw ConstructionFailure{ operation_type };
}
ops_.emplace_back(++max_id_, operation_type);
return max_id_;
}
int max_id_ = 0;
bool throw_on_next_construction_ = false;
std::vector<LifetimeOperation> ops_;
};
struct ToString : pro::dispatch<std::string()> {
template <class T>
std::string operator()(const T& self) {
using std::to_string;
return to_string(self);
}
};
struct TestFacade : pro::facade<ToString> {
static constexpr auto minimum_copyability = pro::constraint_level::nontrivial;
};
int main() {
LifetimeTracker tracker;
std::vector<LifetimeOperation> expected_ops;
{
tracker.ThrowOnNextConstruction();
bool exception_thrown = false;
try {
pro::proxy<TestFacade> p{ std::in_place_type<LifetimeTracker::Session>, &tracker };
} catch (const ConstructionFailure& e) {
exception_thrown = true;
assert(e.type_ ==LifetimeOperationType::kValueConstruction);
}
assert(exception_thrown==true);
assert(tracker.GetOperations() == expected_ops);
}
return 0;
}
The TestFacade outcomes the std::__is_constant_evaluated
segfault. How to resolve this issue in MacOS g++?
Hi @victoryang00, thank you for the feedback! We have tried with a Mac machine but did not reproduce the issue. The version of Apple Clang on our test machine is 13.1.6, which seems to be higher than yours. We will investigate further and ultimately add a pipeline for MacOS platform. In the meantime, could you try to update your Apple Clang compiler and let us know if the issue repros?
It's on M1 mac with compiler gcc 12.1 installed with brew.
It seems that apple clang does not support pmr.
It seems that apple clang does not support pmr.
Maybe we should update the integration test not to rely on pmr
when building a pipeline to run tests on Mac machines, but it should be a separate issue.
@victoryang00
It's on M1 mac with compiler gcc 12.1 installed with brew.
I also tried to install gcc 12.1 on an M1 machine but could not repro either. Here is the command I used to install gcc:
brew install gcc@12
My Homebrew version:
> brew -v
Homebrew 3.5.10
Homebrew/homebrew-core (git revision 817a78a2860; last commit 2022-08-24)
Homebrew/homebrew-cask (git revision c1ae8e54a3; last commit 2022-08-24)
My gcc version:
> g++-12 --version
g++-12 (Homebrew GCC 12.1.0) 12.1.0
My OS configuration:
OS Version: macOS Monterey (Version 12.5.1)
Model Name: MacBook Pro
Model Identifier: MacBookPro18,2
Chip: Apple M1 Max
Memory: 64 GB
System Firmware Version: 7459.141.1
OS Loader Version: 7459.141.1
Interestingly, it still reproduces on my machine with only a difference of macOS 13.
~/project/proxy/cmake-build-debug-gcc-12 on main [!?] via △ v3.23.3
❯ gcc-12 -v (base)
Using built-in specs.
COLLECT_GCC=gcc-12
COLLECT_LTO_WRAPPER=/opt/homebrew/Cellar/gcc@12/12.1.0_1/bin/../libexec/gcc/aarch64-apple-darwin21/12/lto-wrapper
Target: aarch64-apple-darwin21
Configured with: ../configure --prefix=/opt/homebrew/opt/gcc@12 --libdir=/opt/homebrew/opt/gcc@12/lib/gcc/12 --disable-nls --enable-checking=release --with-gcc-major-version-only --enable-languages=c,c++,objc,obj-c++,fortran --program-suffix=-12 --with-gmp=/opt/homebrew/opt/gmp --with-mpfr=/opt/homebrew/opt/mpfr --with-mpc=/opt/homebrew/opt/libmpc --with-isl=/opt/homebrew/opt/isl --with-zstd=/opt/homebrew/opt/zstd --with-pkgversion='Homebrew GCC 12.1.0_1' --with-bugurl=https://github.com/Homebrew/homebrew-core/issues --with-system-zlib --build=aarch64-apple-darwin21 --with-native-system-header-dir=/usr/include --with-sysroot=/Library/Developer/CommandLineTools/SDKs/MacOSX12.sdk
Thread model: posix
Supported LTO compression algorithms: zlib zstd
gcc version 12.1.0 (Homebrew GCC 12.1.0_1)
~/project/proxy/cmake-build-debug-gcc-12 on main [!?] via △ v3.23.3
❯ ld -v (base)
@(#)PROGRAM:ld PROJECT:ld64-819.6
BUILD 15:07:15 Aug 3 2022
configured to support archs: armv6 armv7 armv7s arm64 arm64e arm64_32 i386 x86_64 x86_64h armv6m armv7k armv7m armv7em
LTO support using: LLVM version 14.0.0, (clang-1400.0.29.102) (static support for 29, runtime is 29)
TAPI support using: Apple TAPI version 14.0.0 (tapi-1400.0.11)
~/project/proxy/cmake-build-debug-gcc-12 on main [!?] via △ v3.23.3
❯ uname -a (base)
Darwin eduroam-169-233-207-200.ucsc.edu 22.0.0 Darwin Kernel Version 22.0.0: Mon Aug 1 06:32:20 PDT 2022; root:xnu-8792.0.207.0.6~26/RELEASE_ARM64_T6000 arm64
~/project/proxy/cmake-build-debug-gcc-12 on main [!?] via △ v3.23.3
❯ ninja (base)
[1/3] Linking CXX executable tests/msft_proxy_tests
FAILED: tests/msft_proxy_tests tests/msft_proxy_tests[1]_tests.cmake /Users/yiweiyang/project/proxy/cmake-build-debug-gcc-12/tests/msft_proxy_tests[1]_tests.cmake
: && /opt/homebrew/Cellar/gcc@12/12.1.0_1/bin/g++-12 -Wno-error=packed-not-aligned -Wno-error=shadow -fuse-ld=mold -g -arch arm64 -isysroot /Library/Developer/CommandLineTools/SDKs/MacOSX13.0.sdk -Wl,-search_paths_first -Wl,-headerpad_max_install_names tests/CMakeFiles/msft_proxy_tests.dir/proxy_creation_tests.cpp.o tests/CMakeFiles/msft_proxy_tests.dir/proxy_integration_tests.cpp.o tests/CMakeFiles/msft_proxy_tests.dir/proxy_invocation_tests.cpp.o tests/CMakeFiles/msft_proxy_tests.dir/proxy_lifetime_tests.cpp.o tests/CMakeFiles/msft_proxy_tests.dir/proxy_reflection_tests.cpp.o tests/CMakeFiles/msft_proxy_tests.dir/proxy_traits_tests.cpp.o -o tests/msft_proxy_tests lib/libgtest_maind.a lib/libgtestd.a && cd /Users/yiweiyang/project/proxy/cmake-build-debug-gcc-12/tests && /Applications/CLion.app/Contents/bin/cmake/mac/bin/cmake -D TEST_TARGET=msft_proxy_tests -D TEST_EXECUTABLE=/Users/yiweiyang/project/proxy/cmake-build-debug-gcc-12/tests/msft_proxy_tests -D TEST_EXECUTOR= -D TEST_WORKING_DIR=/Users/yiweiyang/project/proxy/cmake-build-debug-gcc-12/tests -D TEST_EXTRA_ARGS= -D TEST_PROPERTIES= -D TEST_PREFIX= -D TEST_SUFFIX= -D TEST_FILTER= -D NO_PRETTY_TYPES=FALSE -D NO_PRETTY_VALUES=FALSE -D TEST_LIST=msft_proxy_tests_TESTS -D CTEST_FILE=/Users/yiweiyang/project/proxy/cmake-build-debug-gcc-12/tests/msft_proxy_tests[1]_tests.cmake -D TEST_DISCOVERY_TIMEOUT=5 -D TEST_XML_OUTPUT_DIR= -P /Applications/CLion.app/Contents/bin/cmake/mac/share/cmake-3.23/Modules/GoogleTestAddTests.cmake
0 0x104f801a0 __assert_rtn + 140
1 0x104e07a8c mach_o::relocatable::Parser<arm64>::parse(mach_o::relocatable::ParserOptions const&) + 4536
2 0x104dd9d38 mach_o::relocatable::Parser<arm64>::parse(unsigned char const*, unsigned long long, char const*, long, ld::File::Ordinal, mach_o::relocatable::ParserOptions const&) + 148
3 0x104e424ac ld::tool::InputFiles::makeFile(Options::FileInfo const&, bool) + 1468
4 0x104e45360 ___ZN2ld4tool10InputFilesC2ER7Options_block_invoke + 56
5 0x1a185a5b0 _dispatch_client_callout2 + 20
6 0x1a186e024 _dispatch_apply_invoke + 224
7 0x1a185a570 _dispatch_client_callout + 20
8 0x1a186c0c0 _dispatch_root_queue_drain + 684
9 0x1a186c7c0 _dispatch_worker_thread2 + 164
10 0x1a19dd0c4 _pthread_wqthread + 228
A linker snapshot was created at:
/tmp/msft_proxy_tests-2022-08-24-092232.ld-snapshot
ld: Assertion failed: (_file->_atomsArrayCount == computedAtomCount && "more atoms allocated than expected"), function parse, file macho_relocatable_file.cpp, line 2061.
collect2.bak: error: ld returned 1 exit status
CMake Error at /Applications/CLion.app/Contents/bin/cmake/mac/share/cmake-3.23/Modules/GoogleTestAddTests.cmake:93 (message):
Specified test executable does not exist.
Path: '/Users/yiweiyang/project/proxy/cmake-build-debug-gcc-12/tests/msft_proxy_tests'
Call Stack (most recent call first):
/Applications/CLion.app/Contents/bin/cmake/mac/share/cmake-3.23/Modules/GoogleTestAddTests.cmake:225 (gtest_discover_tests_impl)
[2/3] Building CXX object samples/resource_dictionary/CMakeFiles/resource_dictionary.dir/main.cpp.o
FAILED: samples/resource_dictionary/CMakeFiles/resource_dictionary.dir/main.cpp.o
/opt/homebrew/Cellar/gcc@12/12.1.0_1/bin/g++-12 -I/Users/yiweiyang/project/proxy -Wno-error=packed-not-aligned -Wno-error=shadow -fuse-ld=mold -I/Users/yiweiyang/project -g -arch arm64 -isysroot /Library/Developer/CommandLineTools/SDKs/MacOSX13.0.sdk -std=gnu++20 -MD -MT samples/resource_dictionary/CMakeFiles/resource_dictionary.dir/main.cpp.o -MF samples/resource_dictionary/CMakeFiles/resource_dictionary.dir/main.cpp.o.d -o samples/resource_dictionary/CMakeFiles/resource_dictionary.dir/main.cpp.o -c /Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp
/Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp: In function 'std::string PrintDrawableToString(pro::proxy<DrawableFacade>)':
/Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp:33:21: error: aggregate 'std::stringstream result' has incomplete type and cannot be defined
33 | std::stringstream result;
| ^~~~~~
/Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp: In function 'int main()':
/Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp:50:18: error: use of deleted function 'DrawableFacade::DrawableFacade()'
50 | DrawableFacade facade;
| ^~~~~~
/Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp:16:8: note: 'DrawableFacade::DrawableFacade()' is implicitly deleted because the default definition would be ill-formed:
16 | struct DrawableFacade : pro::facade<Draw, Area> {};
| ^~~~~~~~~~~~~~
/Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp:16:8: error: use of deleted function 'pro::facade<Ds>::facade() [with Ds = {Draw, Area}]'
In file included from /Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp:3:
/Users/yiweiyang/project/proxy/proxy.h:38:3: note: declared here
38 | facade() = delete;
| ^~~~~~
/Users/yiweiyang/project/proxy/samples/resource_dictionary/main.cpp:52:36: error: could not convert 'facade' from 'DrawableFacade' to 'pro::proxy<DrawableFacade>'
52 | std::cout<<PrintDrawableToString(facade);
| ^~~~~~
| |
| DrawableFacade
ninja: build stopped: subcommand failed.
I think it's the latest macOS 13 that has some fault in implementing the linker and OS. Since it does not seem to be an implementation of proxy
problem I will close the issue.