canvas-sketch
canvas-sketch copied to clipboard
GUI/HUD Feature
I'm working in a feature/hud
branch to test out the idea of a built-in GUI system for canvas-sketch
. It would be like dat.gui but a bit more opinionated, a lot more declarative, and will integrate easily with canvas-sketch as well as features like exporting/importing serialized data (for example, making prints parameters reproducible).
Here is an example, no fancy styling yet:
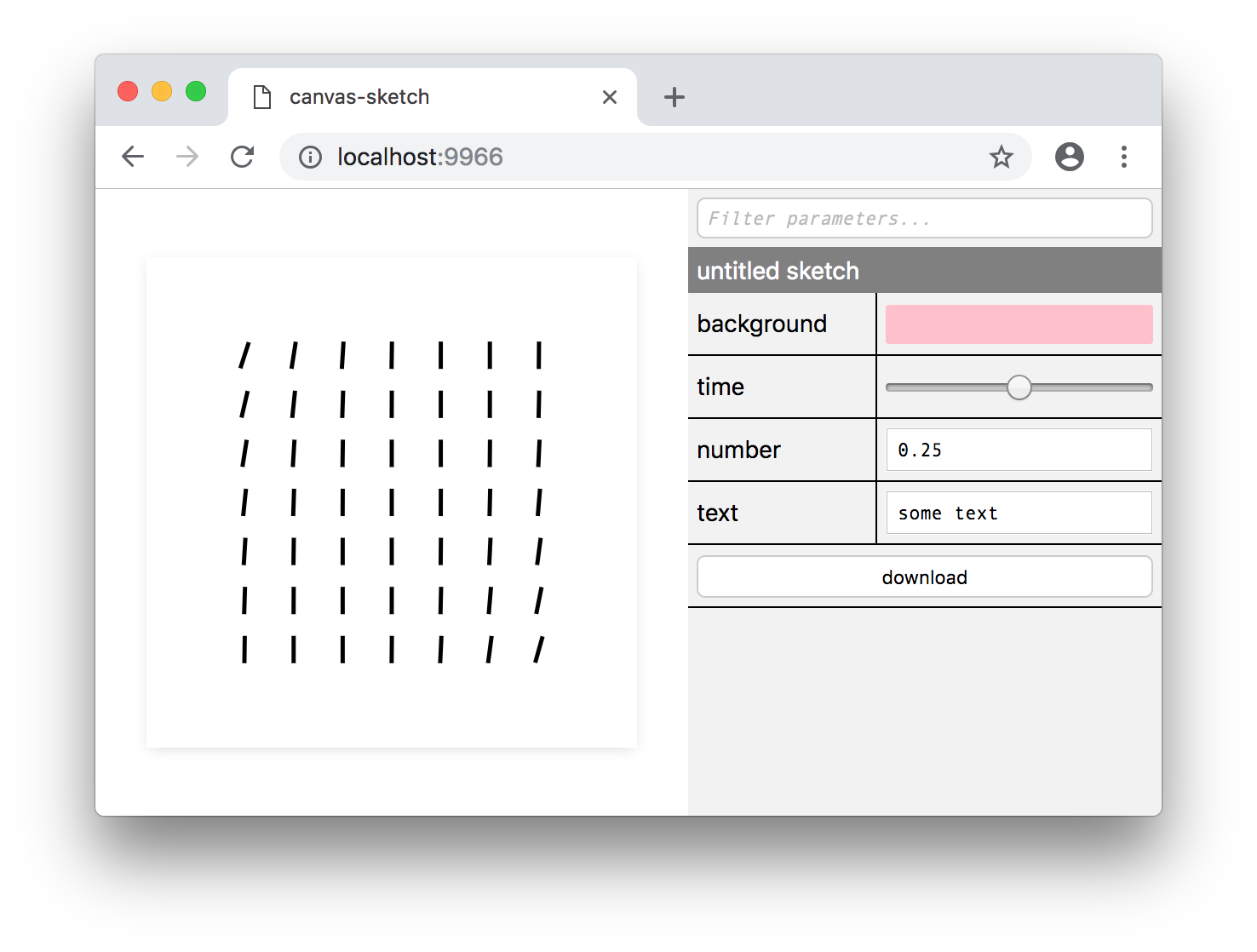
And a video in this tweet.
It would be built with Preact to keep the canvas-sketch
library small.
Syntax
I don't want to introduce a lot of new API concepts to users, and I want it to be declarative and in line with the rest of the ethos of pure render functions. My current thought is to have something like this:
- When
params
is passed tosettings
orupdate()
function, the sketch will be added to a global HUD panel, and the panel will be made visible if it has one or more sketches attached to it. - If the param is an object it can include settings like display name, min/max, etc.
- In the sketch & renderer functions, the
param
prop is converted into literal values (e.g. instead of a descriptor with options, you just get the raw number value).
const canvasSketch = require('canvas-sketch');
const settings = {
dimensions: [ 640, 640 ],
params: {
background: 'pink',
time: {
value: 0.5,
min: 0,
max: 1,
step: 0.001
},
number: 0.25,
text: 'some text',
download: ({ exportFrame }) => exportFrame()
}
};
canvasSketch(() => {
return ({ params }) => {
const { background, radius } = params;
console.log('Current background:', background); // e.g. #ff0000
console.log('Current radius:', radius); // e.g. 0.523
};
}, settings);
Motivation
It won't be all that different than dat.gui, but:
- It will work well out of the box, and will require zero "wiring" to get properties hooked up to GUIs or render events
- It will be declarative, so it can technically be stripped away by a higher-order function that passes props down (like React props & components)
- It will integrate well with canvas-sketch already, but can also make some nice considerations for exporting, e.g. serialize JSON to a file so that each frame/export is saved with the parameters, or adding a
--params
flag to the CLI tool to override params with a JSON file - The global HUD can be a place for other requested features to canvas-sketch, like a play/pause, export button, etc
Features
Should support:
- Text input, number spinners, sliders, color input, 2D XY pad, 3D orbit rotation (?), drop-down, checkbox, buttons, file drag & drop (images etc), ...?
- Fuzzy searching of parameters to quickly drill down big lists?
- Folders or some other way of letting the user organize things?
Questions
- Syntax for buttons/click events? Often users will want to handle the event within the sketch function, rather than before the sketch loads. Maybe some sort of event system?
- Should the parameters be persisted with localStorage? etc.
- How to serialize/unserialize the parameters?
- How to map params to built-in sketch properties like
time
,dimensions
,duration
etc? - Is this a can of worms I don't even want to get into?