mapbox-gl-js
mapbox-gl-js copied to clipboard
Converting Blender camera settings to Mapbox
mapbox-gl-js version: 2.7.0
Currently working on a project where I need to convert three different camera settings found in Blender (position
, rotation
, fov (field of view)
) into camera settings in mapbox.
We are attempting to take a photomatch that we calculated in Blender and transfer it into the 3D environment of Mapbox.
Example:
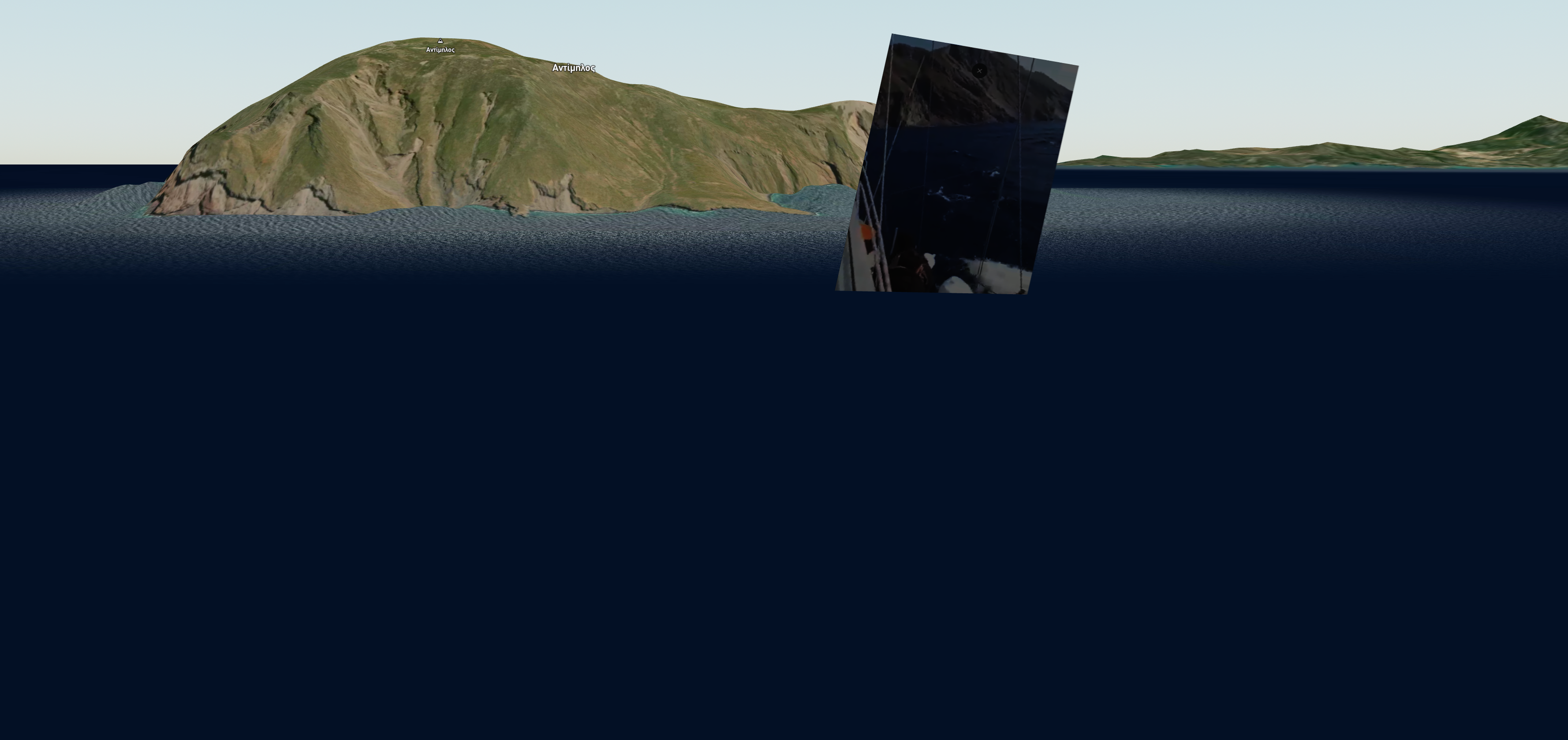
I'm currently attempting to use the freeCameraOptions
available to change these three settings and dont know how I should go about converting any of the three camera settings correctly. The following is pseudocode describing how I am thinking about changing the necessary settings.
/*
* Function to convert an euler angle in the form of (_x, _y, _z)
* into a quaternion legible for Mapbox to read as a rotation
*
* yaw = y-axis = up-down
* roll = z-axis = forward-backward
* pitch = x-axis = left-right
*/
export const eulerToQuaternion = (euler) => {
const {_x, _y, _z} = euler;
const qx = Math.sin(_z / 2) * Math.cos(_x / 2) * Math.cos(_y / 2) - Math.cos(_z / 2) * Math.sin(_x / 2) * Math.sin(_y / 2);
const qy = Math.cos(_z / 2) * Math.sin(_x / 2) * Math.cos(_y / 2) + Math.sin(_z / 2) * Math.cos(_x / 2) * Math.sin(_y / 2);
const qz = Math.cos(_z / 2) * Math.cos(_x / 2) * Math.sin(_y / 2) - Math.sin(_z / 2) * Math.sin(_x / 2) * Math.cos(_y / 2);
const qw = Math.cos(_z / 2) * Math.cos(_x / 2) * Math.cos(_y / 2) + Math.sin(_z / 2) * Math.sin(_x / 2) * Math.sin(_y / 2);
return [qx, qy, qz, qw];
}
const camera = map.getFreeCameraOptions();
// Pseudo code to represent converting cartesian coords to 3D mercator
const convertedCoords = cartesianTo3D(blenderCamera.position)
// Blender camera position is expressed in 3D Cartesian coordinates (xyz), altitude is 0?
camera.position = mapboxgl.MercatorCoordinate.fromLngLat(convertedCoords, 0);
// The rotation angle we receive from Blender is in euler form (see function above)
camera.orientation = eulerToQuaternion(rotation)
map.transform._fov = blenderCamera.fov
map.setFreeCameraOptions(camera)
Any insight to the above would be immensely helpful! Struggling right now to find the appropriate resources.
Hello, just to say that I am also struggling with camera sync'ing, but from Mapbox to Three.js. There is prior art here: https://github.com/jscastro76/threebox/blob/d2a5742a454d30dd2295a892e2e65cc1a41935b8/src/camera/CameraSync.js which may help
More generally, I'd love it if there was some canonical documentation on the algorithm/pseudo-code/logic required to sync Mapbox's camera with other cameras