ChatGPT
ChatGPT copied to clipboard
[Feature] Automatically select model for ChatGPT Plus
Feature description
ChatGPT Plus users have the option between Default and Legacy model
Default model is now the previous "Turbo" model which is quite bad compared to Legacy. Would be nice if this app could automatically select the correct model when starting a new session
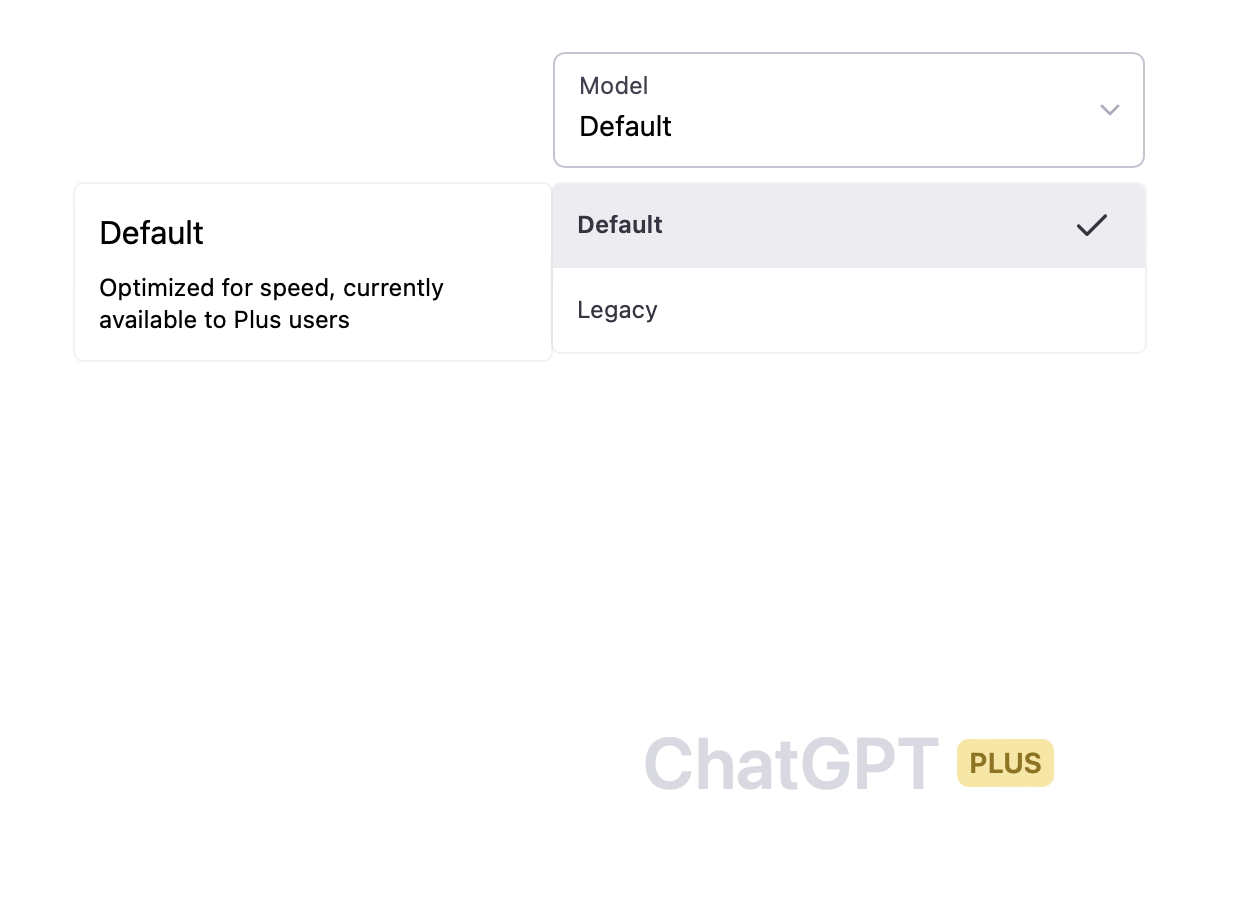
Motivation
No response
Alternatives
No response
Additional context
No response
Here's a ghetto inject script to do this:
const wantedModel = "Legacy";
setInterval(() => {
const selectedModel = document.querySelector("[id^=headlessui-listbox-button] > span").textContent;
console.log("selectedModel", selectedModel);
if (wantedModel === selectedModel) {
return;
}
// Toggle dropdown
document.querySelector("[id^=headlessui-listbox-button]").click();
// timeout to wait until dropdown opened
setTimeout(() => {
// Get models
const models = Array.from(document.querySelectorAll("[id^=headlessui-listbox-option] > li")).reduce((prev, cur) => {
prev[cur.textContent] = cur;
return prev;
}, {});
console.log("models:", models)
// Select Legacy model
models[wantedModel].click();
}, 200);
}, 1000);
Thanks @dvcrn here is the version I use to default to GPT-4:
const wantedModel = "GPT-4";
setInterval(() => {
const selectedModel = document.querySelector("[id^=headlessui-listbox-button] > span");
if (!selectedModel || wantedModel === selectedModel.textContent) {
return;
}
// Toggle dropdown
document.querySelector("[id^=headlessui-listbox-button]").click();
// timeout to wait until dropdown opened
setTimeout(() => {
// Get models
const models = Array.from(document.querySelectorAll("[id^=headlessui-listbox-option] > li")).reduce((prev, cur) => {
prev[cur.textContent] = cur;
return prev;
}, {});
// Select wanted model
models[wantedModel].click();
}, 200);
}, 1000);
changing the chat.conf.json also works
{
"titlebar": false,
"hide_dock_icon": false,
"theme": "dark",
"auto_update": "prompt",
"stay_on_top": true,
"save_window_state": true,
"global_shortcut": "Alt+C",
"default_origin": "https://chat.openai.com/chat?model=gpt-4",
"speech_lang": "Microsoft David - English (United States)",
"isinit": false,
"popup_search": false,
"main_close": false,
"main_dashboard": false,
"main_origin": "https://chat.openai.com/chat?model=gpt-4",
"ua_window": "",
"main_width": 600.0,
"main_height": 600.0,
"tray_width": 530.0,
"tray_height": 800.0,
"tray": true,
"tray_dashboard": false,
"tray_origin": "https://chat.openai.com/chat?model=gpt-4",
"ua_tray": ""
}
changing the chat.conf.json also works
{ "titlebar": false, "hide_dock_icon": false, "theme": "dark", "auto_update": "prompt", "stay_on_top": true, "save_window_state": true, "global_shortcut": "Alt+C", "default_origin": "https://chat.openai.com/chat?model=gpt-4", "speech_lang": "Microsoft David - English (United States)", "isinit": false, "popup_search": false, "main_close": false, "main_dashboard": false, "main_origin": "https://chat.openai.com/chat?model=gpt-4", "ua_window": "", "main_width": 600.0, "main_height": 600.0, "tray_width": 530.0, "tray_height": 800.0, "tray": true, "tray_dashboard": false, "tray_origin": "https://chat.openai.com/chat?model=gpt-4", "ua_tray": "" }
In case someone's trying to find this, it's located under this path (on Windows):
C:\Users\Your_User_Name.chatgpt\chat.conf
Even though this works, clicking on the New chat button would default to GPT 3.5 again. This only works when the app is launched, or when the page is refreshed.
Here's a ghetto inject script to do this:
const wantedModel = "Legacy"; setInterval(() => { const selectedModel = document.querySelector("[id^=headlessui-listbox-button] > span").textContent; console.log("selectedModel", selectedModel); if (wantedModel === selectedModel) { return; } // Toggle dropdown document.querySelector("[id^=headlessui-listbox-button]").click(); // timeout to wait until dropdown opened setTimeout(() => { // Get models const models = Array.from(document.querySelectorAll("[id^=headlessui-listbox-option] > li")).reduce((prev, cur) => { prev[cur.textContent] = cur; return prev; }, {}); console.log("models:", models) // Select Legacy model models[wantedModel].click(); }, 200); }, 1000);
Where do you create the inject script? Did you edit main.js? Some simple instructions would be much appreciated.
@chaoscreater You just replace or append to the contents of main.js which is found in ..\%user%\.chatgpt\scripts\main.js
One thing to note is that the script needs to be modified now that there are multiple options for GPT-4 (web browsing, plugins) and this script will prevent you from being able to select them.