FloatingActionButtonSpeedDial
FloatingActionButtonSpeedDial copied to clipboard
Grey square shown on focused FAB on API 22
Step 1: Are you in the right place?
- [x] I have verified there are no duplicate active or recent bugs, questions, or requests
- [x] I have verified that I am using the latest version of the library.
Step 2: Describe your environment
- Library version:
3.2.0
- Android version:
5.1.1
- Support library version:
1.3.0
(androidx appcompat) - Device brand:
Nexus 6 Emulator
(tried on one other device) - Device model:
Nexus 6 Emulator
Step 3: Describe the problem:
Steps to reproduce:
Open speed dial in basic app on API 22
Observed Results:
A grey square is visible overlaid on top of the first fab menu item (and on the main fab when opening, and any fab when focused or tapped)
Expected Results:
Look the same as e.g. API 23, with no squares
Relevant Code:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
SpeedDialView speedDialView = findViewById(R.id.speedDial);
speedDialView.addActionItem(new SpeedDialActionItem.Builder(R.id.first, getDrawable(R.drawable.ic_launcher_background)).create());
speedDialView.addActionItem(new SpeedDialActionItem.Builder(R.id.second, getDrawable(R.drawable.ic_launcher_background)).create());
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<com.leinardi.android.speeddial.SpeedDialView
android:id="@+id/speedDial"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:sdMainFabClosedSrc="@android:drawable/btn_plus" />
</androidx.constraintlayout.widget.ConstraintLayout>
As a side note, I noticed this while trying to debug why the first FAB item was focused after updating to 3.2.0 before realizing that this was intentional: https://github.com/leinardi/FloatingActionButtonSpeedDial/issues/149 I understand the value of having the first button focused, but the difference in color looks inconsistent. Is it possible to turn off the color change for the focus state? I don't see an easy way to manually specify the selector.
API 22:
API 23:
Yes, I, too, find this feature not quite right. We had to use 3.1.1 for our project because it fits better.
I have the same issue but running it on API 32 now, there is some kind of square in the button's focused state. Did anyone find a way to keep everything rounded?
Using:
implementation "com.google.android.material:material:1.5.0"
On the sample project.
The only way I found to solve the issue was setting rippleColor = Color.TRANSPARENT
like the following:
viewBinding.speedDial.mainFab.rippleColor = Color.TRANSPARENT
viewBinding.speedDial.addActionItem(
SpeedDialActionItem.Builder(R.id.fab_sd_commands, R.drawable.commands)
.setFabBackgroundColor(ContextCompat.getColor(this, R.color.white))
.setLabel(R.string.commands).create()
)?.fab?.rippleColor = Color.TRANSPARENT
@leinardi Is that the best way to solve this? Do you know if maybe this is a bug related to Material's Library FAB?
@fernandomantoan I'm not aware of any other solution. The bug seems to happen only on API 31+. It seems definitely an upstream bug, most probably on com.google.android.material:material
.
@fernandomantoan I'm not aware of any other solution. The bug seems to happen only on API 31+. It seems definitely an upstream bug, most probably on
com.google.android.material:material
.
Ok, thank you anyway. Regards.
I'm opening an issue on the material component library, I can reproduce the issue with just this simple layout:
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:clipChildren="false"
android:clipToPadding="false"
android:layout_gravity="center">
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical|end"
app:fabSize="mini"
app:srcCompat="@drawable/ic_add_white_24dp"
app:tint="@null"
app:useCompatPadding="true" />
</LinearLayout>
I have opened an issue upstream, please consider giving it a :+1: for visibility: https://github.com/material-components/material-components-android/issues/2617
After seeing your snippet I noticed that this attribute is causing the issue: app:useCompatPadding="true"
.
There is an issue opened for this here: https://issuetracker.google.com/issues/37088292 with the status Won't Fix
.
Doesn't seem related since is not involving com.google.android.material:material
nor API 31+.
I don't know but after removing app:useCompatPadding="true"
the issue is gone. Is there any specific reason to use this attribute? I ask this because I've never used it before.
Are you really sure you don't have the issue? I can still reproduce it without the app:useCompatPadding="true"
, if I use the app:fabSize="mini"
:
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:clipChildren="false"
android:clipToPadding="false"
android:gravity="center"
android:orientation="vertical">
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:srcCompat="@drawable/ic_add_white_24dp"
app:useCompatPadding="true" />
<Space
android:layout_width="24dp"
android:layout_height="24dp" />
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:fabSize="mini"
app:srcCompat="@drawable/ic_add_white_24dp" />
</LinearLayout>
Repro branch available here: https://github.com/leinardi/FloatingActionButtonSpeedDial/tree/issue-2617
You are right, setting app:fabSize="mini"
will also result in a bugged shadow.
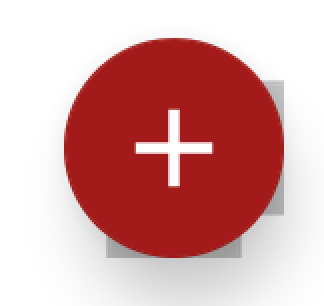