jnativehook
jnativehook copied to clipboard
Printable character keys not triggering NativeKeyListener
Environment info:
IDE = Eclipse 2019-12 IDE
JavaFX = version 13
jnativehook = version 2.1.0
OS = macOS Catalina 10.15.3
I’m trying to set up a simple test to respond to system keyboard events using this library. Here is the relevant class:
import java.util.logging.Level;
import org.jnativehook.GlobalScreen;
import org.jnativehook.NativeHookException;
import org.jnativehook.keyboard.NativeKeyEvent;
import org.jnativehook.keyboard.NativeKeyListener;
import org.jnativehook.mouse.NativeMouseEvent;
import org.jnativehook.mouse.NativeMouseInputListener;
/*
* My application class calls Watcher.init()
*/
public class Watcher {
private static final int KEY_SHF_L_MAC = 42;
private static final int KEY_SHF_R_MAC = 54;
private static final int KEY_CAP_MAC = 58;
private static final int KEY_CTL_MAC = 29;
private static final int KEY_ALT_L_MAC = 56;
private static final int KEY_ALT_R_MAC = 3640;
private static final int KEY_CMD_L_MAC = 3675;
private static final int KEY_CMD_R_MAC = 3676;
public static void init() throws NativeHookException {
//disable jnativehook logging
java.util.logging.Logger logger = java.util.logging.Logger.getLogger(GlobalScreen.class.getPackage().getName());
logger.setLevel(Level.WARNING);
logger.setUseParentHandlers(false);
//register native hook
GlobalScreen.registerNativeHook();
NativeKeyListener nativeKeyListener = new NativeKeyListener() {
public void nativeKeyPressed(NativeKeyEvent e) {
int key = e.getKeyCode();
boolean alphanumeric = (key >= NativeKeyEvent.VC_A && key <= NativeKeyEvent.VC_Z) ||
(key >= NativeKeyEvent.VC_1 && key <= NativeKeyEvent.VC_0);
String message = "native key " + key + " pressed, name = ";
String keyString;
switch (key) {
case KEY_SHF_L_MAC:
case KEY_SHF_R_MAC:
keyString = "SHIFT";
break;
case KEY_CAP_MAC:
keyString = "CAPS_LOCK";
break;
case KEY_CTL_MAC:
keyString = "CONTROL";
break;
case KEY_ALT_L_MAC:
case KEY_ALT_R_MAC:
keyString = "ALT/OPTION";
break;
case KEY_CMD_L_MAC:
case KEY_CMD_R_MAC:
keyString = "COMMAND";
break;
default:
keyString = "UNKNOWN";
break;
}
System.out.println(message + keyString);
}
public void nativeKeyReleased(NativeKeyEvent e) {
int key = e.getKeyCode();
boolean alphanumeric = (key >= NativeKeyEvent.VC_A && key <= NativeKeyEvent.VC_Z) ||
(key >= NativeKeyEvent.VC_1 && key <= NativeKeyEvent.VC_0);
System.out.println("native key " + key + " released, alphanumeric=" + alphanumeric);
}
public void nativeKeyTyped(NativeKeyEvent e) {
int keychar = e.getKeyChar();
System.out.println("native key char " + keychar + " typed");
}
};
GlobalScreen.addNativeKeyListener(nativeKeyListener);
System.out.println("watcher init success");
}
}
To test, I then ran the program and proceeded to hit some keys: 1, 2, 3, ..., 0, Q, W, E, ..., M, some punctuation keys, caps lock, left shift, fn, left control, left alt, left command, space, right command, right alt, right shift, and the arrow keys. Below are the results in System.out:
native key 58 pressed, name = CAPS_LOCK
native key 58 released, alphanumeric=false
native key 58 pressed, name = CAPS_LOCK
native key 58 released, alphanumeric=false
native key 42 pressed, name = SHIFT
native key 42 released, alphanumeric=true
native key 29 pressed, name = CONTROL
native key 29 released, alphanumeric=false
native key 56 pressed, name = ALT/OPTION
native key 56 released, alphanumeric=false
native key 3675 pressed, name = COMMAND
native key 3675 released, alphanumeric=false
native key 3676 pressed, name = COMMAND
native key 3676 released, alphanumeric=false
native key 3640 pressed, name = ALT/OPTION
native key 3640 released, alphanumeric=false
native key 54 pressed, name = SHIFT
native key 54 released, alphanumeric=false
I’m not getting any indication of keys being pressed or released by any other characters other than caps lock, shift, control, alt, and command. Anyone know why?
I think this error pretty much exactly matches issue #201, though that issue has been closed without a clear solution.
When I turn on logging, for the keys that do register I also get these messages:
mar. 31, 2020 11:27:55 A. M. org.jnativehook.GlobalScreen$NativeHookThread enable
INFO: process_modifier_changed [479]: Modifiers Changed for key 0X38. (0X100)
mar. 31, 2020 11:27:55 A. M. org.jnativehook.GlobalScreen$NativeHookThread enable
INFO: process_key_released [468]: Key 0X2A released. (0X38)
But most keys don’t generate any messages at all.
I’ve also tried restarting my laptop and running the program again, but with no changes.
I recently tried using jnativehook version 2.1.0 instead of 2.0.2, but no luck still.
~I was wondering if the issue fixed in libuiohook pull request 69 is perhaps related?~ Edit: those changes seem not related after all.
Taking into account that jnativehook uses libuiohook to connect to the system keyboard events, I downloaded and compiled demo_hook from univrsal/libuiohook, which is the version I can compile on my computer.
I wanted to run a program with libuiohook on its own to confirm it works. Even though the version I compiled and ran is not exactly the same as the dependency for jnativehook v2.1.0, I figure they’re pretty close. Below is the output from demo_hook (I moved my mouse to a text editor window, clicked, typed a sentence, then pressed cmd+q
to close the editor, and ctrl+c
to close demo_hook):
id=1,when=38589988581407,mask=0x0
id=5,when=38589986040502,mask=0xA000,keycode=28,rawcode=0x24
id=9,when=38592321590535,mask=0xA000,x=334,y=132,button=0,clicks=0
id=9,when=38592409592420,mask=0xA000,x=335,y=132,button=0,clicks=0
id=9,when=38592441580180,mask=0xA000,x=335,y=132,button=0,clicks=0
id=9,when=38592489656687,mask=0xA000,x=335,y=132,button=0,clicks=0
id=9,when=38592497449294,mask=0xA000,x=336,y=132,button=0,clicks=0
id=9,when=38592513594675,mask=0xA000,x=336,y=132,button=0,clicks=0
id=9,when=38592521813463,mask=0xA000,x=337,y=132,button=0,clicks=0
id=9,when=38592529467416,mask=0xA000,x=338,y=132,button=0,clicks=0
id=9,when=38592537661948,mask=0xA000,x=339,y=132,button=0,clicks=0
id=9,when=38592545295346,mask=0xA000,x=340,y=132,button=0,clicks=0
id=9,when=38592553621626,mask=0xA000,x=342,y=132,button=0,clicks=0
id=9,when=38592561276682,mask=0xA000,x=344,y=131,button=0,clicks=0
id=9,when=38592569400853,mask=0xA000,x=345,y=131,button=0,clicks=0
id=9,when=38592577465675,mask=0xA000,x=347,y=131,button=0,clicks=0
id=9,when=38592585491107,mask=0xA000,x=349,y=131,button=0,clicks=0
id=9,when=38592593678477,mask=0xA000,x=350,y=131,button=0,clicks=0
id=9,when=38592601598851,mask=0xA000,x=351,y=131,button=0,clicks=0
id=9,when=38592609186953,mask=0xA000,x=352,y=131,button=0,clicks=0
id=9,when=38592617152498,mask=0xA000,x=353,y=131,button=0,clicks=0
id=9,when=38592625148465,mask=0xA000,x=354,y=131,button=0,clicks=0
id=9,when=38592633282426,mask=0xA000,x=355,y=131,button=0,clicks=0
id=9,when=38592641161886,mask=0xA000,x=357,y=131,button=0,clicks=0
id=9,when=38592651176098,mask=0xA000,x=358,y=131,button=0,clicks=0
id=9,when=38592659172687,mask=0xA000,x=359,y=131,button=0,clicks=0
id=9,when=38592667139608,mask=0xA000,x=361,y=131,button=0,clicks=0
id=9,when=38592675158240,mask=0xA000,x=362,y=131,button=0,clicks=0
id=9,when=38592683168238,mask=0xA000,x=363,y=131,button=0,clicks=0
id=9,when=38592691147944,mask=0xA000,x=364,y=131,button=0,clicks=0
id=9,when=38592699141517,mask=0xA000,x=366,y=131,button=0,clicks=0
id=9,when=38592707235158,mask=0xA000,x=367,y=131,button=0,clicks=0
id=9,when=38592715149791,mask=0xA000,x=369,y=131,button=0,clicks=0
id=9,when=38592723204969,mask=0xA000,x=370,y=131,button=0,clicks=0
id=9,when=38592731137230,mask=0xA000,x=372,y=131,button=0,clicks=0
id=9,when=38592739137723,mask=0xA000,x=374,y=131,button=0,clicks=0
id=9,when=38592747148717,mask=0xA000,x=378,y=131,button=0,clicks=0
id=9,when=38592755157019,mask=0xA000,x=381,y=131,button=0,clicks=0
id=9,when=38592763142066,mask=0xA000,x=383,y=131,button=0,clicks=0
id=9,when=38592771169021,mask=0xA000,x=387,y=130,button=0,clicks=0
id=9,when=38592779219501,mask=0xA000,x=389,y=130,button=0,clicks=0
id=9,when=38592787162099,mask=0xA000,x=392,y=129,button=0,clicks=0
id=9,when=38592795132503,mask=0xA000,x=394,y=129,button=0,clicks=0
id=9,when=38592803212689,mask=0xA000,x=395,y=129,button=0,clicks=0
id=9,when=38592811147450,mask=0xA000,x=397,y=129,button=0,clicks=0
id=9,when=38592819169498,mask=0xA000,x=398,y=129,button=0,clicks=0
id=9,when=38592827139795,mask=0xA000,x=400,y=129,button=0,clicks=0
id=9,when=38592835146534,mask=0xA000,x=401,y=129,button=0,clicks=0
id=9,when=38592843190477,mask=0xA000,x=403,y=129,button=0,clicks=0
id=9,when=38592851404187,mask=0xA000,x=406,y=129,button=0,clicks=0
id=9,when=38592859582973,mask=0xA000,x=410,y=129,button=0,clicks=0
id=9,when=38592867448849,mask=0xA000,x=414,y=129,button=0,clicks=0
id=9,when=38592875586560,mask=0xA000,x=417,y=129,button=0,clicks=0
id=9,when=38592883454242,mask=0xA000,x=424,y=129,button=0,clicks=0
id=9,when=38592891297784,mask=0xA000,x=430,y=129,button=0,clicks=0
id=9,when=38592899365213,mask=0xA000,x=435,y=129,button=0,clicks=0
id=9,when=38592907596352,mask=0xA000,x=440,y=129,button=0,clicks=0
id=9,when=38592915517950,mask=0xA000,x=446,y=130,button=0,clicks=0
id=9,when=38592923449956,mask=0xA000,x=452,y=131,button=0,clicks=0
id=9,when=38592931153186,mask=0xA000,x=458,y=133,button=0,clicks=0
id=9,when=38592939154632,mask=0xA000,x=465,y=134,button=0,clicks=0
id=9,when=38592947181545,mask=0xA000,x=472,y=134,button=0,clicks=0
id=9,when=38592955558451,mask=0xA000,x=480,y=136,button=0,clicks=0
id=9,when=38592963370434,mask=0xA000,x=488,y=138,button=0,clicks=0
id=9,when=38592971412495,mask=0xA000,x=497,y=139,button=0,clicks=0
id=9,when=38592979447546,mask=0xA000,x=508,y=141,button=0,clicks=0
id=9,when=38592987427019,mask=0xA000,x=518,y=143,button=0,clicks=0
id=9,when=38592995712910,mask=0xA000,x=528,y=145,button=0,clicks=0
id=9,when=38593003597947,mask=0xA000,x=539,y=147,button=0,clicks=0
id=9,when=38593011158839,mask=0xA000,x=551,y=151,button=0,clicks=0
id=9,when=38593019391903,mask=0xA000,x=561,y=153,button=0,clicks=0
id=9,when=38593027399527,mask=0xA000,x=572,y=156,button=0,clicks=0
id=9,when=38593035583598,mask=0xA000,x=584,y=158,button=0,clicks=0
id=9,when=38593043599478,mask=0xA000,x=596,y=160,button=0,clicks=0
id=9,when=38593051193594,mask=0xA000,x=608,y=164,button=0,clicks=0
id=9,when=38593059634005,mask=0xA000,x=621,y=166,button=0,clicks=0
id=9,when=38593067449422,mask=0xA000,x=633,y=168,button=0,clicks=0
id=9,when=38593075588067,mask=0xA000,x=643,y=170,button=0,clicks=0
id=9,when=38593083493350,mask=0xA000,x=654,y=172,button=0,clicks=0
id=9,when=38593091411045,mask=0xA000,x=662,y=173,button=0,clicks=0
id=9,when=38593099141834,mask=0xA000,x=666,y=174,button=0,clicks=0
id=9,when=38593107604683,mask=0xA000,x=673,y=176,button=0,clicks=0
id=9,when=38593115356648,mask=0xA000,x=679,y=177,button=0,clicks=0
id=9,when=38593123384550,mask=0xA000,x=686,y=178,button=0,clicks=0
id=9,when=38593131293425,mask=0xA000,x=695,y=180,button=0,clicks=0
id=9,when=38593139588773,mask=0xA000,x=699,y=180,button=0,clicks=0
id=9,when=38593147430080,mask=0xA000,x=705,y=181,button=0,clicks=0
id=9,when=38593155615571,mask=0xA000,x=711,y=182,button=0,clicks=0
id=9,when=38593163380226,mask=0xA000,x=716,y=183,button=0,clicks=0
id=9,when=38593171419552,mask=0xA000,x=722,y=184,button=0,clicks=0
id=9,when=38593179609739,mask=0xA000,x=724,y=184,button=0,clicks=0
id=9,when=38593187450854,mask=0xA000,x=729,y=185,button=0,clicks=0
id=9,when=38593195589619,mask=0xA000,x=733,y=186,button=0,clicks=0
id=9,when=38593203605945,mask=0xA000,x=737,y=186,button=0,clicks=0
id=9,when=38593211166840,mask=0xA000,x=741,y=186,button=0,clicks=0
id=9,when=38593219400645,mask=0xA000,x=745,y=186,button=0,clicks=0
id=9,when=38593227663458,mask=0xA000,x=752,y=186,button=0,clicks=0
id=9,when=38593235586634,mask=0xA000,x=754,y=186,button=0,clicks=0
id=9,when=38593243578261,mask=0xA000,x=762,y=186,button=0,clicks=0
id=9,when=38593251204281,mask=0xA000,x=766,y=186,button=0,clicks=0
id=9,when=38593259595207,mask=0xA000,x=772,y=186,button=0,clicks=0
id=9,when=38593267271722,mask=0xA000,x=775,y=186,button=0,clicks=0
id=9,when=38593275560704,mask=0xA000,x=780,y=186,button=0,clicks=0
id=9,when=38593283399500,mask=0xA000,x=784,y=186,button=0,clicks=0
id=9,when=38593291383975,mask=0xA000,x=789,y=186,button=0,clicks=0
id=9,when=38593299389626,mask=0xA000,x=792,y=186,button=0,clicks=0
id=9,when=38593307604406,mask=0xA000,x=796,y=186,button=0,clicks=0
id=9,when=38593315518896,mask=0xA000,x=799,y=186,button=0,clicks=0
id=9,when=38593323510210,mask=0xA000,x=802,y=185,button=0,clicks=0
id=9,when=38593331178668,mask=0xA000,x=806,y=184,button=0,clicks=0
id=9,when=38593339596365,mask=0xA000,x=809,y=184,button=0,clicks=0
id=9,when=38593347697056,mask=0xA000,x=813,y=183,button=0,clicks=0
id=9,when=38593355747899,mask=0xA000,x=816,y=182,button=0,clicks=0
id=9,when=38593363571557,mask=0xA000,x=822,y=181,button=0,clicks=0
id=9,when=38593371357486,mask=0xA000,x=824,y=180,button=0,clicks=0
id=9,when=38593379533030,mask=0xA000,x=830,y=179,button=0,clicks=0
id=9,when=38593387458571,mask=0xA000,x=834,y=178,button=0,clicks=0
id=9,when=38593395399549,mask=0xA000,x=838,y=176,button=0,clicks=0
id=9,when=38593405589188,mask=0xA000,x=842,y=175,button=0,clicks=0
id=9,when=38593411193264,mask=0xA000,x=847,y=174,button=0,clicks=0
id=9,when=38593421417275,mask=0xA000,x=853,y=173,button=0,clicks=0
id=9,when=38593429602492,mask=0xA000,x=857,y=172,button=0,clicks=0
id=9,when=38593437593120,mask=0xA000,x=862,y=171,button=0,clicks=0
id=9,when=38593445585234,mask=0xA000,x=868,y=169,button=0,clicks=0
id=9,when=38593453411926,mask=0xA000,x=874,y=167,button=0,clicks=0
id=9,when=38593461588270,mask=0xA000,x=877,y=167,button=0,clicks=0
id=9,when=38593469452153,mask=0xA000,x=882,y=165,button=0,clicks=0
id=9,when=38593477694992,mask=0xA000,x=886,y=164,button=0,clicks=0
id=9,when=38593485540161,mask=0xA000,x=890,y=162,button=0,clicks=0
id=9,when=38593493379425,mask=0xA000,x=892,y=162,button=0,clicks=0
id=9,when=38593501200422,mask=0xA000,x=895,y=160,button=0,clicks=0
id=9,when=38593509633325,mask=0xA000,x=896,y=160,button=0,clicks=0
id=9,when=38593517505687,mask=0xA000,x=897,y=160,button=0,clicks=0
id=9,when=38593525339644,mask=0xA000,x=897,y=159,button=0,clicks=0
id=9,when=38593533203709,mask=0xA000,x=898,y=159,button=0,clicks=0
id=9,when=38593541586751,mask=0xA000,x=898,y=159,button=0,clicks=0
id=9,when=38593549620800,mask=0xA000,x=899,y=159,button=0,clicks=0
id=9,when=38593557510165,mask=0xA000,x=899,y=159,button=0,clicks=0
id=9,when=38593597504602,mask=0xA000,x=899,y=158,button=0,clicks=0
id=9,when=38593605189982,mask=0xA000,x=899,y=158,button=0,clicks=0
id=7,when=38593897684548,mask=0xA100,x=899,y=158,button=1,clicks=1
id=8,when=38593904372228,mask=0xA000,x=899,y=158,button=1,clicks=1
id=6,when=38593904372228,mask=0xA000,x=899,y=158,button=1,clicks=1
id=4,when=38601066217981,mask=0xA010,keycode=54,rawcode=0x3C
id=4,when=38601186223084,mask=0xA010,keycode=20,rawcode=0x11
id=3,when=38601186223084,mask=0xA010,keychar=T,rawcode=17
id=5,when=38601266210078,mask=0xA010,keycode=20,rawcode=0x11
id=5,when=38601282224696,mask=0xA000,keycode=54,rawcode=0x3C
id=4,when=38601890108834,mask=0xA000,keycode=18,rawcode=0xE
id=3,when=38601890108834,mask=0xA000,keychar=e,rawcode=14
id=5,when=38601962209205,mask=0xA000,keycode=18,rawcode=0xE
id=4,when=38602146114515,mask=0xA000,keycode=31,rawcode=0x1
id=3,when=38602146114515,mask=0xA000,keychar=s,rawcode=1
id=4,when=38602218084521,mask=0xA000,keycode=20,rawcode=0x11
id=3,when=38602218084521,mask=0xA000,keychar=t,rawcode=17
id=5,when=38602258496808,mask=0xA000,keycode=31,rawcode=0x1
id=5,when=38602314077842,mask=0xA000,keycode=20,rawcode=0x11
id=4,when=38602706014908,mask=0xA000,keycode=23,rawcode=0x22
id=3,when=38602706014908,mask=0xA000,keychar=i,rawcode=34
id=4,when=38602762009357,mask=0xA000,keycode=49,rawcode=0x2D
id=3,when=38602762009357,mask=0xA000,keychar=n,rawcode=45
id=5,when=38602810042792,mask=0xA000,keycode=23,rawcode=0x22
id=4,when=38602826042474,mask=0xA000,keycode=34,rawcode=0x5
id=3,when=38602826042474,mask=0xA000,keychar=g,rawcode=5
id=5,when=38602866078833,mask=0xA000,keycode=49,rawcode=0x2D
id=5,when=38602930062769,mask=0xA000,keycode=34,rawcode=0x5
id=4,when=38603722039635,mask=0xA000,keycode=57,rawcode=0x31
id=3,when=38603722039635,mask=0xA000,keychar= ,rawcode=49
id=5,when=38603810016846,mask=0xA000,keycode=57,rawcode=0x31
id=4,when=38604042210310,mask=0xA000,keycode=32,rawcode=0x2
id=3,when=38604042210310,mask=0xA000,keychar=d,rawcode=2
id=5,when=38604114039320,mask=0xA000,keycode=32,rawcode=0x2
id=4,when=38604570206737,mask=0xA000,keycode=18,rawcode=0xE
id=3,when=38604570206737,mask=0xA000,keychar=e,rawcode=14
id=5,when=38604650014270,mask=0xA000,keycode=18,rawcode=0xE
id=4,when=38604722035297,mask=0xA000,keycode=50,rawcode=0x2E
id=3,when=38604722035297,mask=0xA000,keychar=m,rawcode=46
id=5,when=38604802014286,mask=0xA000,keycode=50,rawcode=0x2E
id=4,when=38606074054488,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38606074054488,mask=0xA000,keychar=o,rawcode=31
id=5,when=38606146063903,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38606346128907,mask=0xA010,keycode=54,rawcode=0x3C
id=4,when=38606410069251,mask=0xA010,keycode=12,rawcode=0x1B
id=3,when=38606410069251,mask=0xA010,keychar=_,rawcode=27
id=5,when=38606522062650,mask=0xA010,keycode=12,rawcode=0x1B
id=5,when=38606530066558,mask=0xA000,keycode=54,rawcode=0x3C
id=4,when=38606930087658,mask=0xA000,keycode=35,rawcode=0x4
id=3,when=38606930087658,mask=0xA000,keychar=h,rawcode=4
id=5,when=38606994078457,mask=0xA000,keycode=35,rawcode=0x4
id=4,when=38607122203319,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38607122203319,mask=0xA000,keychar=o,rawcode=31
id=5,when=38607170033957,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38607234231803,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38607234231803,mask=0xA000,keychar=o,rawcode=31
id=5,when=38607306218527,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38607410209332,mask=0xA000,keycode=37,rawcode=0x28
id=3,when=38607410209332,mask=0xA000,keychar=k,rawcode=40
id=5,when=38607490224058,mask=0xA000,keycode=37,rawcode=0x28
id=4,when=38607682012899,mask=0xA000,keycode=57,rawcode=0x31
id=3,when=38607682012899,mask=0xA000,keychar= ,rawcode=49
id=5,when=38607746038707,mask=0xA000,keycode=57,rawcode=0x31
id=4,when=38607834024568,mask=0xA000,keycode=30,rawcode=0x0
id=3,when=38607834024568,mask=0xA000,keychar=a,rawcode=0
id=5,when=38607906159931,mask=0xA000,keycode=30,rawcode=0x0
id=4,when=38607930218873,mask=0xA000,keycode=49,rawcode=0x2D
id=3,when=38607930218873,mask=0xA000,keychar=n,rawcode=45
id=5,when=38607994158265,mask=0xA000,keycode=49,rawcode=0x2D
id=4,when=38608002061736,mask=0xA000,keycode=32,rawcode=0x2
id=3,when=38608002061736,mask=0xA000,keychar=d,rawcode=2
id=5,when=38608074206179,mask=0xA000,keycode=32,rawcode=0x2
id=4,when=38608114076609,mask=0xA000,keycode=57,rawcode=0x31
id=3,when=38608114076609,mask=0xA000,keychar= ,rawcode=49
id=5,when=38608210209955,mask=0xA000,keycode=57,rawcode=0x31
id=4,when=38608746017739,mask=0xA000,keycode=38,rawcode=0x25
id=3,when=38608746017739,mask=0xA000,keychar=l,rawcode=37
id=5,when=38608866012302,mask=0xA000,keycode=38,rawcode=0x25
id=4,when=38609138223209,mask=0xA000,keycode=23,rawcode=0x22
id=3,when=38609138223209,mask=0xA000,keychar=i,rawcode=34
id=5,when=38609218206777,mask=0xA000,keycode=23,rawcode=0x22
id=4,when=38609514218022,mask=0xA000,keycode=48,rawcode=0xB
id=3,when=38609514218022,mask=0xA000,keychar=b,rawcode=11
id=5,when=38609602015667,mask=0xA000,keycode=48,rawcode=0xB
id=4,when=38610778016133,mask=0xA000,keycode=22,rawcode=0x20
id=3,when=38610778016133,mask=0xA000,keychar=u,rawcode=32
id=5,when=38610842224108,mask=0xA000,keycode=22,rawcode=0x20
id=4,when=38611178064051,mask=0xA000,keycode=23,rawcode=0x22
id=3,when=38611178064051,mask=0xA000,keychar=i,rawcode=34
id=5,when=38611250226635,mask=0xA000,keycode=23,rawcode=0x22
id=4,when=38611474222848,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38611474222848,mask=0xA000,keychar=o,rawcode=31
id=5,when=38611554282281,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38612146217367,mask=0xA000,keycode=35,rawcode=0x4
id=3,when=38612146217367,mask=0xA000,keychar=h,rawcode=4
id=5,when=38612218223541,mask=0xA000,keycode=35,rawcode=0x4
id=4,when=38612426303800,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38612426303800,mask=0xA000,keychar=o,rawcode=31
id=5,when=38612474053316,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38612546019529,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38612546019529,mask=0xA000,keychar=o,rawcode=31
id=5,when=38612618100605,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38613562218067,mask=0xA000,keycode=37,rawcode=0x28
id=3,when=38613562218067,mask=0xA000,keychar=k,rawcode=40
id=5,when=38613626214621,mask=0xA000,keycode=37,rawcode=0x28
id=4,when=38616234220162,mask=0xA000,keycode=57,rawcode=0x31
id=3,when=38616234220162,mask=0xA000,keychar= ,rawcode=49
id=5,when=38616306165560,mask=0xA000,keycode=57,rawcode=0x31
id=4,when=38616402213841,mask=0xA000,keycode=2,rawcode=0x12
id=3,when=38616402213841,mask=0xA000,keychar=1,rawcode=18
id=5,when=38616482192856,mask=0xA000,keycode=2,rawcode=0x12
id=4,when=38617642043836,mask=0xA000,keycode=57,rawcode=0x31
id=3,when=38617642043836,mask=0xA000,keychar= ,rawcode=49
id=5,when=38617706048774,mask=0xA000,keycode=57,rawcode=0x31
id=4,when=38617874225299,mask=0xA000,keycode=20,rawcode=0x11
id=3,when=38617874225299,mask=0xA000,keychar=t,rawcode=17
id=5,when=38617938219342,mask=0xA000,keycode=20,rawcode=0x11
id=4,when=38618178265355,mask=0xA000,keycode=23,rawcode=0x22
id=3,when=38618178265355,mask=0xA000,keychar=i,rawcode=34
id=4,when=38618226065767,mask=0xA000,keycode=50,rawcode=0x2E
id=3,when=38618226065767,mask=0xA000,keychar=m,rawcode=46
id=5,when=38618266052731,mask=0xA000,keycode=23,rawcode=0x22
id=5,when=38618298213598,mask=0xA000,keycode=50,rawcode=0x2E
id=4,when=38618330217218,mask=0xA000,keycode=18,rawcode=0xE
id=3,when=38618330217218,mask=0xA000,keychar=e,rawcode=14
id=5,when=38618394206863,mask=0xA000,keycode=18,rawcode=0xE
id=4,when=38619986031675,mask=0xA000,keycode=52,rawcode=0x2F
id=3,when=38619986031675,mask=0xA000,keychar=.,rawcode=47
id=5,when=38620058223362,mask=0xA000,keycode=52,rawcode=0x2F
id=4,when=38620538224635,mask=0xA000,keycode=28,rawcode=0x24
,rawcode=368620538224635,mask=0xA000,keychar=
id=5,when=38620610012832,mask=0xA000,keycode=28,rawcode=0x24
id=4,when=38621226226346,mask=0xA010,keycode=54,rawcode=0x3C
id=4,when=38621330222308,mask=0xA010,keycode=35,rawcode=0x4
id=3,when=38621330222308,mask=0xA010,keychar=H,rawcode=4
id=5,when=38621426222587,mask=0xA010,keycode=35,rawcode=0x4
id=5,when=38621434218535,mask=0xA000,keycode=54,rawcode=0x3C
id=4,when=38621602226722,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38621602226722,mask=0xA000,keychar=o,rawcode=31
id=5,when=38621658038874,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38621722070222,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38621722070222,mask=0xA000,keychar=o,rawcode=31
id=5,when=38621802042140,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38622010235973,mask=0xA000,keycode=19,rawcode=0xF
id=3,when=38622010235973,mask=0xA000,keychar=r,rawcode=15
id=5,when=38622082206717,mask=0xA000,keycode=19,rawcode=0xF
id=4,when=38622402220578,mask=0xA000,keycode=30,rawcode=0x0
id=3,when=38622402220578,mask=0xA000,keychar=a,rawcode=0
id=5,when=38622474160207,mask=0xA000,keycode=30,rawcode=0x0
id=4,when=38622506224929,mask=0xA000,keycode=21,rawcode=0x10
id=3,when=38622506224929,mask=0xA000,keychar=y,rawcode=16
id=5,when=38622562217049,mask=0xA000,keycode=21,rawcode=0x10
id=4,when=38626562206300,mask=0xA010,keycode=54,rawcode=0x3C
id=4,when=38626706038176,mask=0xA010,keycode=2,rawcode=0x12
id=3,when=38626706038176,mask=0xA010,keychar=!,rawcode=18
id=5,when=38626770009216,mask=0xA010,keycode=2,rawcode=0x12
id=5,when=38626818119351,mask=0xA000,keycode=54,rawcode=0x3C
id=4,when=38628146220858,mask=0xA000,keycode=15,rawcode=0x30
id=3,when=38628146220858,mask=0xA000,keychar= ,rawcode=48
id=5,when=38628234218754,mask=0xA000,keycode=15,rawcode=0x30
id=4,when=38629442100980,mask=0xA000,keycode=15,rawcode=0x30
id=3,when=38629442100980,mask=0xA000,keychar= ,rawcode=48
id=5,when=38629530220950,mask=0xA000,keycode=15,rawcode=0x30
id=4,when=38630754016935,mask=0xA010,keycode=54,rawcode=0x3C
id=4,when=38631042219147,mask=0xA010,keycode=41,rawcode=0x32
id=3,when=38631042219147,mask=0xA010,keychar=~,rawcode=50
id=5,when=38631122013505,mask=0xA010,keycode=41,rawcode=0x32
id=5,when=38631162225234,mask=0xA000,keycode=54,rawcode=0x3C
id=4,when=38632658025829,mask=0xA000,keycode=24,rawcode=0x1F
id=3,when=38632658025829,mask=0xA000,keychar=o,rawcode=31
id=5,when=38632738042752,mask=0xA000,keycode=24,rawcode=0x1F
id=4,when=38632802151950,mask=0xA000,keycode=34,rawcode=0x5
id=3,when=38632802151950,mask=0xA000,keychar=g,rawcode=5
id=5,when=38632866174677,mask=0xA000,keycode=34,rawcode=0x5
id=4,when=38632978222868,mask=0xA000,keycode=30,rawcode=0x0
id=3,when=38632978222868,mask=0xA000,keychar=a,rawcode=0
id=5,when=38633050094510,mask=0xA000,keycode=30,rawcode=0x0
id=4,when=38633066226491,mask=0xA000,keycode=38,rawcode=0x25
id=3,when=38633066226491,mask=0xA000,keychar=l,rawcode=37
id=5,when=38633130226478,mask=0xA000,keycode=38,rawcode=0x25
id=4,when=38633194208524,mask=0xA000,keycode=38,rawcode=0x25
id=3,when=38633194208524,mask=0xA000,keychar=l,rawcode=37
id=4,when=38633234077731,mask=0xA000,keycode=30,rawcode=0x0
id=3,when=38633234077731,mask=0xA000,keychar=a,rawcode=0
id=5,when=38633282222953,mask=0xA000,keycode=38,rawcode=0x25
id=4,when=38633322081408,mask=0xA000,keycode=34,rawcode=0x5
id=3,when=38633322081408,mask=0xA000,keychar=g,rawcode=5
id=5,when=38633378283428,mask=0xA000,keycode=30,rawcode=0x0
id=5,when=38633394201966,mask=0xA000,keycode=34,rawcode=0x5
id=4,when=38633434211906,mask=0xA000,keycode=35,rawcode=0x4
id=3,when=38633434211906,mask=0xA000,keychar=h,rawcode=4
id=5,when=38633490043759,mask=0xA000,keycode=35,rawcode=0x4
id=4,when=38633530072511,mask=0xA000,keycode=18,rawcode=0xE
id=3,when=38633530072511,mask=0xA000,keychar=e,rawcode=14
id=4,when=38633570057082,mask=0xA000,keycode=19,rawcode=0xF
id=3,when=38633570057082,mask=0xA000,keychar=r,rawcode=15
id=5,when=38633618039635,mask=0xA000,keycode=18,rawcode=0xE
id=5,when=38633642041128,mask=0xA000,keycode=19,rawcode=0xF
id=4,when=38719239536778,mask=0xA004,keycode=3675,rawcode=0x37
id=4,when=38719399335578,mask=0xA004,keycode=16,rawcode=0xC
id=3,when=38719399335578,mask=0xA004,keychar=q,rawcode=12
id=5,when=38719495334600,mask=0xA004,keycode=16,rawcode=0xC
id=5,when=38719527343499,mask=0xA000,keycode=3675,rawcode=0x37
id=4,when=38726591544930,mask=0xA002,keycode=29,rawcode=0x3B
id=4,when=38726751333442,mask=0xA002,keycode=46,rawcode=0x8
id=3,when=38726751333442,mask=0xA002,keychar=c,rawcode=8
^C
And this is what I typed in the editor during the test:
Testing demo_hook and libuiohook 1 time.
Hooray! ~ogallagher
In conclusion, from what I can tell it looks like my issues with NativeKeyListener are not caused by libuiohook, which appears to work perfectly.
I’ve been trying to investigate this issue for the past few days by cloning this project, modifying the java and JNI c code (just inserting debugging outputs and such), rebuilding jnativehook-2.1.0.jar
following the wiki instructions with ant, and including the jar in a test program. ~However, though my edits to the java source files do appear, I can’t seem to successfully modify the c files and have those changes carry through to appear in my testing program~ (I did figure out how to recompile the dylib
eventually). I’m pretty sure ant is recompiling and including lib/darwin/x86_64/libJNativeHook.dylib
in the new output jar that I use in the testing program, so I’m confused.
At this point I’d add the question
label if I could.
From testing I’ve confirmed as fact the following (on my computer):
- libuiohook registers all events correctly when demonstrated by the demo program included, demo_hook.
- jnativehook only handles mouse and non-printable character key events.
- I tried adding output/print statements to key places in libuiohook, such as the beginning of input_hook.c:hook_event_proc(tap_proxy,type,event_ref,refcon), being the method that handles each CGEvent and passes it to the correct event processor method. These print statements do appear when running demo_hook.c, but do not appear for the problem keys when running JNativeHookDemo.java. In JNativeHookDemo it’s still as if nothing happens for the printable character keys.
I’m still stuck on this problem. @kwhat or anyone do you know what might be issue?
I think the issue has to do with some events not passing through to the native event tap when created by the JVM. This time, I created a custom spin-off of demo_hook (let’s call it uiohook_driver), which I was planning to call via java.lang.Runtime.exec(systemCommand, null, dir)
, instead of using the JNI.
When running uiohook_driver by itself from the terminal, here’s the output, corresponding to moving the mouse, clicking a text box, typing “hello, there”, typing ESC twice, and quitting via CTRL+C:
when=159936164480283,type=mm,x=337,y=103,button=0,clicks=0
...
when=159937374226685,type=mp,x=1374,y=12,button=1,clicks=1
when=159937380788772,type=mr,x=1374,y=12,button=1,clicks=1
when=159937380788772,type=mc,x=1374,y=12,button=1,clicks=1
when=159939928768192,type=kp,key=35
when=159940008780773,type=kr,key=35
when=159941448741900,type=kp,key=18
when=159941512740047,type=kr,key=18
when=159941656745560,type=kp,key=38
when=159941720753008,type=kr,key=38
when=159941800772991,type=kp,key=38
when=159941880748966,type=kr,key=38
when=159942368941766,type=kp,key=24
when=159942432752232,type=kr,key=24
when=159943104746834,type=kp,key=51
when=159943168741390,type=kr,key=51
when=159944968743920,type=kp,key=57
when=159945048756616,type=kr,key=57
when=159945240931084,type=kp,key=20
when=159945328748690,type=kr,key=20
when=159945424766993,type=kp,key=35
when=159945496741727,type=kr,key=35
when=159945504743328,type=kp,key=18
when=159945576753214,type=kp,key=19
when=159945584749856,type=kr,key=18
when=159945656743989,type=kr,key=19
when=159945672764047,type=kp,key=18
when=159945768737558,type=kr,key=18
when=159951840719182,type=kp,key=1
when=159951944773448,type=kr,key=1
when=159952848749498,type=kp,key=1
when=159952960935658,type=kr,key=1
when=159954424943012,type=kp,key=29
when=159954528952144,type=kp,key=46
Here’s the output of the same program, uiohook_driver, when called from within the Java application using Runtime.exec(...)
:
when=161421329539307,type=mm,x=1242,y=38,button=0,clicks=0
...
when=161424743526776,type=mp,x=1031,y=41,button=1,clicks=1
when=161424750539657,type=mr,x=1031,y=41,button=1,clicks=1
when=161424750539657,type=mc,x=1031,y=41,button=1,clicks=1
when=161425496180319,type=kp,key=3676
when=161425584171660,type=kr,key=3676
when=161428280204338,type=kp,key=3676
when=161428360177103,type=kr,key=3676
when=161429577571385,type=mm,x=1031,y=41,button=0,clicks=0
when=161429585428518,type=mm,x=1036,y=41,button=0,clicks=0
when=161429593545132,type=mm,x=1042,y=40,button=0,clicks=0
The only key that was registered when invoked from the JVM was the right command/meta key, which is key code 3676, when I quit the Java app with CMD+Q.
Did you ever find a solution for this? I have the exact same problem. On Mac OS JNativeHook only detects shift, ctrl, alt, cmd, and then the media keys (prev, next, play, volume up, volume down, eject).
@mjparme Can you try the latest 2.2.2? I'll have to look into this tomorrow and see if I can duplicate the issue. I don't have a physical mac so I may not be able to reproduce the issue. Please add any details that will help reproduce the problem.
I just tested with 2.2.2 w/ Java 17 on Catalina and it seems to be working. I am able to get key press, typed and released events for basic letter keys (a, s, d, f, g, etc).
I am using 2.2.2 with Java 17 on Big Sur and I do not get notifications for the basic letter keys.
Well, I've wasted several hours trying to upgrade my VM to Big Sur and I've given up for the foreseeable future. I am flabbergasted at how difficult Apple makes something as simple as disk management or partition resizing. Even restoring a disk to a new larger drive is too difficult for the Disk Utility. I give up.
Here is a gif of the problem I am having. Detects the non-printable keys but not the letter keys. I have another mac that has Monterey on it, I will give it a test on that.
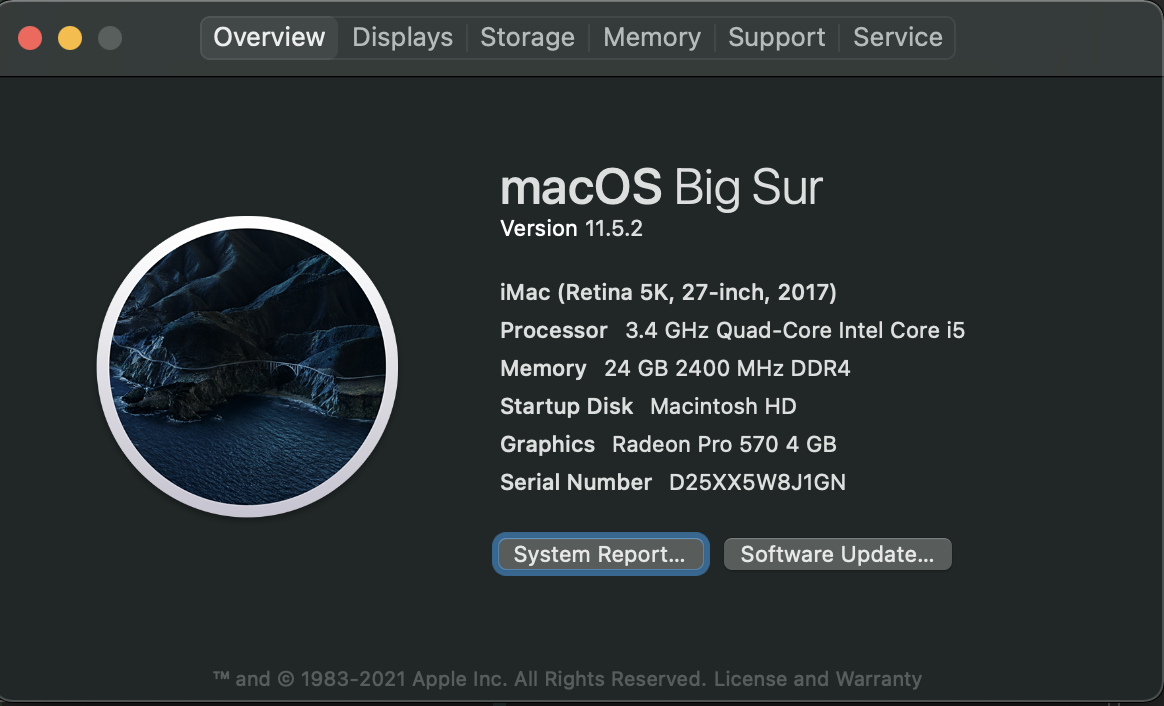
I am using Java 17 with nativehook 2.2.2 (implementation 'com.github.kwhat:jnativehook:2.2.2'
I am using the GlobalKeyListener from the documentation.
I have kind of worked around it for now. I used a double tap of ctrl as my hotkey (two taps within 250 ms). That will be fine for now.
Set the log level to debug and pastebin that output. I doubt it will be enough to track down the problem but its better than nothing. 🤷
I cannot duplicate the issue on Big Sur with JRE17.
IF this is a problem with the library, the problem is likely here. The hook_event_proc
isn't getting kCGEventKeyDown
or kCGEventKeyUp
events for some reason which doesn't make any sense because it is basically listening for everything.
The animated gif provided does not show the demo jar running so I suspect something else is wrong here. Please run the demo jar and provide a log at level ALL.
I had wanted to try the demo jar as part of my troubleshooting before commenting on this issue but unfortunately I have been unable to find it. It isn't in the repo and as far as I can tell there is no maven target to build it. I also don't see it on the releases page. So I have been unable to test it out.
The README simply says The graphical example application exists to provide a real-time demonstration of raw output for all available native events. To run the application simply execute the jar file provided.
But the jar file isn't provided anywhere as far as I can tell.
I have configured ALL logging as documented here (https://github.com/kwhat/jnativehook/blob/2.2/doc/ConsoleOutput.md) in the Set the log level to display everything.
section. But no log is being generated and no log output is going to the console. So I don't know where the log is going. I have never used java.util.logging (never even seen it used quite honestly) so don't know how to figure out where the log is going.
I’ve not revisited this issue for a long time, so I’m rusty on the details and am playing around with a clean slate.
However, I did just clone v2.2 and prep the project for building the jar files as described in compiling.md by downloading library-resources
to src/main/resources
and configuring my IDE to use Java SE 13.
Below is output of the maven package
command’s tests phase. Note some tests fail. Is this relevant?
`mvn package` tests output
[INFO] -------------------------------------------------------
[INFO] T E S T S
[INFO] -------------------------------------------------------
[DEBUG] Determined Maven Process ID 25775
[DEBUG] boot classpath: /Users/owengallagher/.m2/repository/org/apache/maven/surefire/surefire-booter/3.0.0-M4/surefire-booter-3.0.0-M4.jar /Users/owengallagher/.m2/repository/org/apache/maven/surefire/surefire-api/3.0.0-M4/surefire-api-3.0.0-M4.jar /Users/owengallagher/.m2/repository/org/apache/maven/surefire/surefire-logger-api/3.0.0-M4/surefire-logger-api-3.0.0-M4.jar /Users/owengallagher/Documents/java/eclipse/jnativehook/target/test-classes /Users/owengallagher/.m2/repository/org/junit/jupiter/junit-jupiter/5.8.2/junit-jupiter-5.8.2.jar /Users/owengallagher/.m2/repository/org/junit/jupiter/junit-jupiter-api/5.7.0/junit-jupiter-api-5.7.0.jar /Users/owengallagher/.m2/repository/org/apiguardian/apiguardian-api/1.1.0/apiguardian-api-1.1.0.jar /Users/owengallagher/.m2/repository/org/opentest4j/opentest4j/1.2.0/opentest4j-1.2.0.jar /Users/owengallagher/.m2/repository/org/junit/platform/junit-platform-commons/1.7.0/junit-platform-commons-1.7.0.jar /Users/owengallagher/.m2/repository/org/junit/jupiter/junit-jupiter-params/5.7.0/junit-jupiter-params-5.7.0.jar /Users/owengallagher/.m2/repository/org/junit/jupiter/junit-jupiter-engine/5.7.0/junit-jupiter-engine-5.7.0.jar /Users/owengallagher/.m2/repository/org/junit/platform/junit-platform-engine/1.7.0/junit-platform-engine-1.7.0.jar /Users/owengallagher/.m2/repository/org/apache/maven/surefire/surefire-junit-platform/3.0.0-M4/surefire-junit-platform-3.0.0-M4.jar /Users/owengallagher/.m2/repository/org/apache/maven/surefire/common-java5/3.0.0-M4/common-java5-3.0.0-M4.jar /Users/owengallagher/.m2/repository/org/junit/platform/junit-platform-launcher/1.7.0/junit-platform-launcher-1.7.0.jar
[DEBUG] boot(compact) classpath: surefire-booter-3.0.0-M4.jar surefire-api-3.0.0-M4.jar surefire-logger-api-3.0.0-M4.jar test-classes junit-jupiter-5.8.2.jar junit-jupiter-api-5.7.0.jar apiguardian-api-1.1.0.jar opentest4j-1.2.0.jar junit-platform-commons-1.7.0.jar junit-jupiter-params-5.7.0.jar junit-jupiter-engine-5.7.0.jar junit-platform-engine-1.7.0.jar surefire-junit-platform-3.0.0-M4.jar common-java5-3.0.0-M4.jar junit-platform-launcher-1.7.0.jar
[DEBUG] Path to args file: /Users/owengallagher/Documents/java/eclipse/jnativehook/target/surefire/surefireargs9331846715161585654
[DEBUG] args file content:
--module-path
"/Users/owengallagher/Documents/java/eclipse/jnativehook/target/classes"
--class-path
"/Users/owengallagher/.m2/repository/org/apache/maven/surefire/surefire-booter/3.0.0-M4/surefire-booter-3.0.0-M4.jar:/Users/owengallagher/.m2/repository/org/apache/maven/surefire/surefire-api/3.0.0-M4/surefire-api-3.0.0-M4.jar:/Users/owengallagher/.m2/repository/org/apache/maven/surefire/surefire-logger-api/3.0.0-M4/surefire-logger-api-3.0.0-M4.jar:/Users/owengallagher/Documents/java/eclipse/jnativehook/target/test-classes:/Users/owengallagher/.m2/repository/org/junit/jupiter/junit-jupiter/5.8.2/junit-jupiter-5.8.2.jar:/Users/owengallagher/.m2/repository/org/junit/jupiter/junit-jupiter-api/5.7.0/junit-jupiter-api-5.7.0.jar:/Users/owengallagher/.m2/repository/org/apiguardian/apiguardian-api/1.1.0/apiguardian-api-1.1.0.jar:/Users/owengallagher/.m2/repository/org/opentest4j/opentest4j/1.2.0/opentest4j-1.2.0.jar:/Users/owengallagher/.m2/repository/org/junit/platform/junit-platform-commons/1.7.0/junit-platform-commons-1.7.0.jar:/Users/owengallagher/.m2/repository/org/junit/jupiter/junit-jupiter-params/5.7.0/junit-jupiter-params-5.7.0.jar:/Users/owengallagher/.m2/repository/org/junit/jupiter/junit-jupiter-engine/5.7.0/junit-jupiter-engine-5.7.0.jar:/Users/owengallagher/.m2/repository/org/junit/platform/junit-platform-engine/1.7.0/junit-platform-engine-1.7.0.jar:/Users/owengallagher/.m2/repository/org/apache/maven/surefire/surefire-junit-platform/3.0.0-M4/surefire-junit-platform-3.0.0-M4.jar:/Users/owengallagher/.m2/repository/org/apache/maven/surefire/common-java5/3.0.0-M4/common-java5-3.0.0-M4.jar:/Users/owengallagher/.m2/repository/org/junit/platform/junit-platform-launcher/1.7.0/junit-platform-launcher-1.7.0.jar"
--patch-module
com.github.kwhat.jnativehook="/Users/owengallagher/Documents/java/eclipse/jnativehook/target/test-classes"
--add-exports
com.github.kwhat.jnativehook/com.github.kwhat.jnativehook=ALL-UNNAMED
--add-exports
com.github.kwhat.jnativehook/com.github.kwhat.jnativehook.dispatcher=ALL-UNNAMED
--add-exports
com.github.kwhat.jnativehook/com.github.kwhat.jnativehook.keyboard=ALL-UNNAMED
--add-exports
com.github.kwhat.jnativehook/com.github.kwhat.jnativehook.keyboard.listeners=ALL-UNNAMED
--add-exports
com.github.kwhat.jnativehook/com.github.kwhat.jnativehook.mouse=ALL-UNNAMED
--add-exports
com.github.kwhat.jnativehook/com.github.kwhat.jnativehook.mouse.listeners=ALL-UNNAMED
--add-modules
com.github.kwhat.jnativehook
--add-reads
com.github.kwhat.jnativehook=ALL-UNNAMED
org.apache.maven.surefire.booter.ForkedBooter
[DEBUG] Forking command line: /bin/sh -c cd /Users/owengallagher/Documents/java/eclipse/jnativehook && /Library/Java/JavaVirtualMachines/jdk-13.0.2.jdk/Contents/Home/bin/java '-javaagent:/Users/owengallagher/.m2/repository/org/jacoco/org.jacoco.agent/0.8.6/org.jacoco.agent-0.8.6-runtime.jar=destfile=/Users/owengallagher/Documents/java/eclipse/jnativehook/target/jacoco.exec,excludes=META-INF/**' @/Users/owengallagher/Documents/java/eclipse/jnativehook/target/surefire/surefireargs9331846715161585654 /Users/owengallagher/Documents/java/eclipse/jnativehook/target/surefire 2022-03-23T00-33-27_071-jvmRun1 surefire12926747091390317366tmp surefire_08569509359147751363tmp
[DEBUG] PpidChecker{ppid=25775, stopped=false, canExecuteLocalUnixPs=false, canExecuteStandardUnixPs=true}
[INFO] Running com.github.kwhat.jnativehook.dispatcher.VoidDispatchServiceTest
[INFO] Tests run: 5, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.102 s - in com.github.kwhat.jnativehook.dispatcher.VoidDispatchServiceTest
[INFO] Running com.github.kwhat.jnativehook.mouse.SwingMouseAdapterTest
[INFO] Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.469 s - in com.github.kwhat.jnativehook.mouse.SwingMouseAdapterTest
[INFO] Running com.github.kwhat.jnativehook.mouse.NativeMouseMotionListenerTest
Received id=2504,when=0,mask=100000000,modifiers=Button1NATIVE_MOUSE_DRAGGED,(50,75),button=0,modifiers=Button1,clickCount=0
Received id=2503,when=0,mask=0,modifiers=NATIVE_MOUSE_MOVED,(50,75),button=0,clickCount=0
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.02 s - in com.github.kwhat.jnativehook.mouse.NativeMouseMotionListenerTest
[INFO] Running com.github.kwhat.jnativehook.mouse.NativeMouseWheelEventTest
[INFO] Tests run: 4, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.018 s - in com.github.kwhat.jnativehook.mouse.NativeMouseWheelEventTest
[INFO] Running com.github.kwhat.jnativehook.mouse.NativeMouseListenerTest
Received id=2500,when=0,mask=0,modifiers=NATIVE_MOUSE_CLICKED,(50,75),button=1,clickCount=1
Received id=2502,when=0,mask=0,modifiers=NATIVE_MOUSE_RELEASED,(50,75),button=1,clickCount=1
nativeMousePressed
Received id=2501,when=0,mask=0,modifiers=NATIVE_MOUSE_PRESSED,(50,75),button=1,clickCount=1
[INFO] Tests run: 3, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.012 s - in com.github.kwhat.jnativehook.mouse.NativeMouseListenerTest
[INFO] Running com.github.kwhat.jnativehook.mouse.NativeMouseWheelListenerTest
[INFO] Tests run: 1, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.006 s - in com.github.kwhat.jnativehook.mouse.NativeMouseWheelListenerTest
[INFO] Running com.github.kwhat.jnativehook.mouse.NativeMouseEventTest
[INFO] Tests run: 6, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.054 s - in com.github.kwhat.jnativehook.mouse.NativeMouseEventTest
[INFO] Running com.github.kwhat.jnativehook.NativeHookExceptionTest
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.004 s - in com.github.kwhat.jnativehook.NativeHookExceptionTest
[INFO] Running com.github.kwhat.jnativehook.NativeInputEventTest
[INFO] Tests run: 6, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.015 s - in com.github.kwhat.jnativehook.NativeInputEventTest
[INFO] Running com.github.kwhat.jnativehook.NativeSystemTest
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.057 s - in com.github.kwhat.jnativehook.NativeSystemTest
[INFO] Running com.github.kwhat.jnativehook.keyboard.NativeKeyListenerTest
[INFO] Tests run: 3, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.012 s - in com.github.kwhat.jnativehook.keyboard.NativeKeyListenerTest
[INFO] Running com.github.kwhat.jnativehook.keyboard.NativeKeyEventTest
[ERROR] Tests run: 11, Failures: 1, Errors: 0, Skipped: 0, Time elapsed: 0.178 s <<< FAILURE! - in com.github.kwhat.jnativehook.keyboard.NativeKeyEventTest
[ERROR] com.github.kwhat.jnativehook.keyboard.NativeKeyEventTest.testParamString Time elapsed: 0.011 s <<< FAILURE!
org.opentest4j.AssertionFailedError: expected: <true> but was: <false>
at com.github.kwhat.jnativehook/com.github.kwhat.jnativehook.keyboard.NativeKeyEventTest.testParamString(NativeKeyEventTest.java:191)
[INFO] Running com.github.kwhat.jnativehook.NativeMonitorInfoTest
[INFO] Tests run: 6, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.04 s - in com.github.kwhat.jnativehook.NativeMonitorInfoTest
[INFO]
[INFO] Results:
[INFO]
[ERROR] Failures:
[ERROR] NativeKeyEventTest.testParamString:191 expected: <true> but was: <false>
[INFO]
[ERROR] Tests run: 52, Failures: 1, Errors: 0, Skipped: 0
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] BUILD FAILURE
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 14.024 s
[INFO] Finished at: 2022-03-23T00:33:31-04:00
Current environment info:
Apache Maven 3.8.4 (9b656c72d54e5bacbed989b64718c159fe39b537)
Maven home: /Users/owengallagher/Documents/java/eclipse/jnativehook/EMBEDDED
Java version: 13.0.2, vendor: Oracle Corporation, runtime: /Library/Java/JavaVirtualMachines/jdk-13.0.2.jdk/Contents/Home
Default locale: es_419, platform encoding: UTF-8
OS name: "mac os x", version: "10.15.7", arch: "x86_64", family: "mac"
I finally whipped java.util.logging into shape. I had to add a file handler and simple formatter and then I started getting logs in the file indicated in the file handler. For some reason JUL writes binary log files. No idea why. But I do now understand why no ones uses JUL and instead uses 3rd party logging libraries. JUL is all around awful.
There are a lot of mouse move entries, I wanted to remove those but the binary log format didn't make that very easy. Since it is binary grep -v
just said "binary file matches".
When I ran my little test app I got log entries when pressing the non-printable characters, but no log entries occurred when I pressed the alphanumeric characters.
I am still trying to locate the demo jar so I can test with that. It has remained elusive.
Ok, well now I feel dumb. The library jar also has the demo app in it. java -jar jnativehook-2.2.2.jar
resulted in the demo app firing up (for some reason I decided to give that a try and wasn't expecting anything and then it actually worked).
The demo app works correctly:
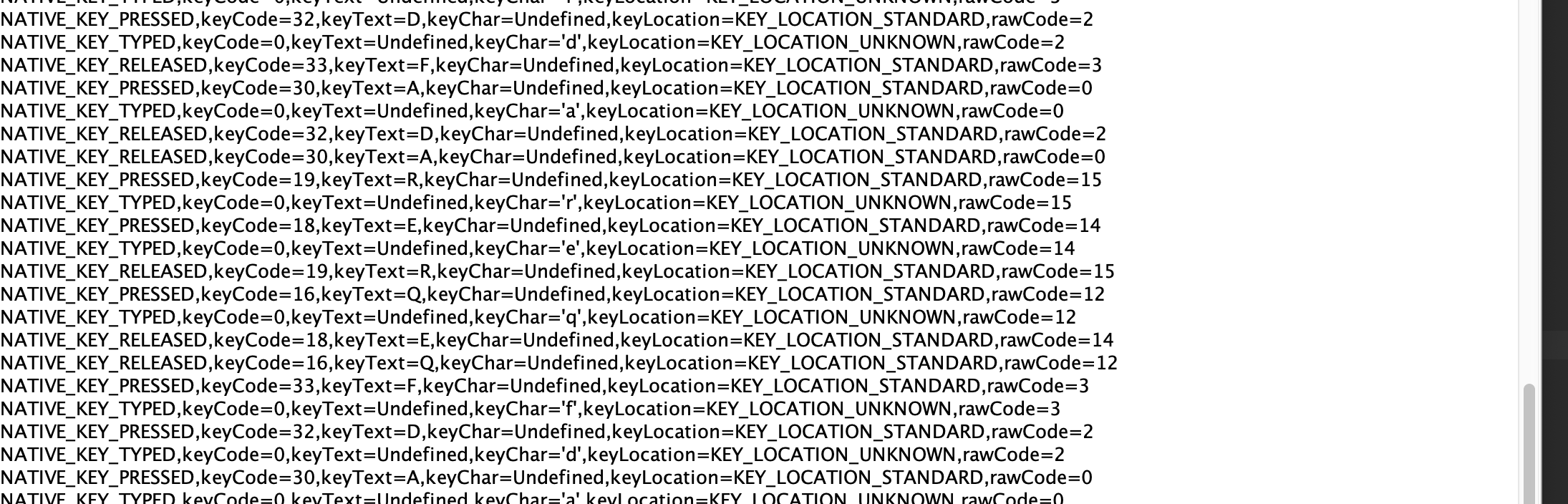
I will take a look at its source to see what I am doing differently than what it is doing.
I just don't see what is different between what the demo app is doing and what my app is doing. I also decided to give that Watcher
class a try that @ogallagher posted in his first comment. I added a main() to it so I could run it standalone and I get the same behavior with that code as I do my own. (i.e. non-printable keys work, printable characters do not).
Here I captured the startup of that Watcher class in the log (i did press alphanumeric keys here to test but they don't show in the logs...they just aren't being detected):
Mar 23, 2022 12:12:35 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: hook_run [1270]: Accessibility API is enabled.
Mar 23, 2022 12:12:35 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: hook_run [1275]: CFRunLoopGetCurrent successful.
Mar 23, 2022 12:12:35 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: create_event_runloop_info [1188]: CGEventTapCreate Successful.
Mar 23, 2022 12:12:35 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: create_event_runloop_info [1200]: CFMachPortCreateRunLoopSource successful.
Mar 23, 2022 12:12:35 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: create_event_runloop_info [1218]: CFRunLoopObserverCreate successful.
Mar 23, 2022 12:12:35 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: dispatch_event [116]: Dispatching event type 1.
Mar 23, 2022 12:12:46 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_modifier_changed [376]: Modifiers Changed for key 0X37. (0X100108)
Mar 23, 2022 12:12:46 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_key_pressed [265]: Key 0XE5B pressed. (0X37)
Mar 23, 2022 12:12:46 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: dispatch_event [116]: Dispatching event type 4.
Mar 23, 2022 12:12:46 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_key_pressed [278]: Using dispatch_sync_f for key typed events.
Mar 23, 2022 12:12:46 AM com.thebadprogrammer.autoclicker.Watcher$1 nativeKeyPressed
FINE: key pressed3675
Mar 23, 2022 12:12:46 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_modifier_changed [376]: Modifiers Changed for key 0X37. (0X100)
Mar 23, 2022 12:12:46 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_key_released [365]: Key 0XE5B released. (0X37)
Mar 23, 2022 12:12:46 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: dispatch_event [116]: Dispatching event type 5.
Mar 23, 2022 12:12:53 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_modifier_changed [376]: Modifiers Changed for key 0X3A. (0X80120)
Mar 23, 2022 12:12:53 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_key_pressed [265]: Key 0X38 pressed. (0X3A)
Mar 23, 2022 12:12:53 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: dispatch_event [116]: Dispatching event type 4.
Mar 23, 2022 12:12:53 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_key_pressed [278]: Using dispatch_sync_f for key typed events.
Mar 23, 2022 12:12:53 AM com.thebadprogrammer.autoclicker.Watcher$1 nativeKeyPressed
FINE: key pressed56
Mar 23, 2022 12:12:54 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_modifier_changed [376]: Modifiers Changed for key 0X3A. (0X100)
Mar 23, 2022 12:12:54 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: process_key_released [365]: Key 0X38 released. (0X3A)
Mar 23, 2022 12:12:54 AM com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread enable
FINE: dispatch_event [116]: Dispatching event type 5.
Below is output of the maven
package
command’s tests phase. Note some tests fail. Is this relevant?
@ogallagher The test failure on OS X has been resolved in the 2.2 branch. It was not serious, just an oversight on my part because I never use OS X.
@mjparme, @ogallagher So what thread is keeping the Watcher program running? I presume we aren't doing this:
public static void main(String[] args)
{
Watcher.init();
}
The only thing I can think of is that there is a thread safety issue somewhere but without a full working example I'm not going to figure it out.
@kwhat it looks like it is the com.github.kwhat.jnativehook.GlobalScreen$NativeHookThread
thread that keeps it alive. That is not a daemon thread and as far as I can tell this.enable()
starts a native thread and then blocks until an exception is thrown or until the JVM exits.
FWIW, my main method looks like this:
public static void main(String[] args) throws NativeHookException, IOException {
Watcher watcher = new Watcher();
watcher.init();
}
I changed init
to be non-static. It stays alive until I stop it.