capacitor
capacitor copied to clipboard
bug: Capacitor 4.3 Http/Cookies: Unhandled Promise Rejection: DataCloneError: The object can not be cloned.
Capacitor Doctor
Latest Dependencies:
@capacitor/cli: 4.3.0
@capacitor/core: 4.3.0
@capacitor/android: 4.3.0
@capacitor/ios: 4.3.0
Installed Dependencies:
@capacitor/cli: 4.3.0
@capacitor/core: 4.3.0
@capacitor/ios: 4.3.0
@capacitor/android: 4.3.0
Platform(s)
iOS & Android
Current Behavior
When using Capacitor Http/Cookies on a local build, Mapbox will not load its content anymore. However, this works fine when using the website, or using Capacitor and pointing to the server where the App is hosted on. So it is most likely not related to the implementation.
Possibly this is related to cookies.
Image link: https://user-images.githubusercontent.com/89409894/191803876-cd037583-7a31-4566-b31d-c7a373651b25.png
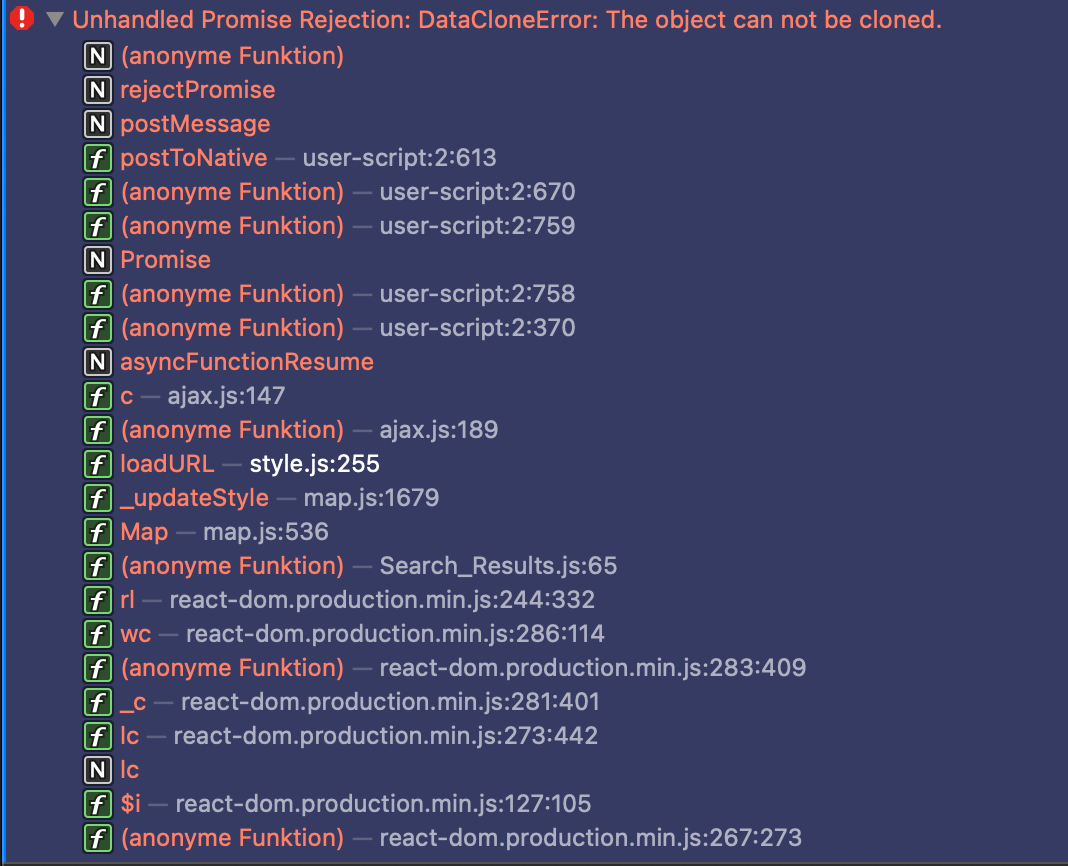
Code
import * as MapboxGeocoder from '@mapbox/mapbox-gl-geocoder';
import * as mapboxgl from '!mapbox-gl';
export default function SearchResults(...props) {
let map;
let geocoder = new MapboxGeocoder({
accessToken: "MYKEY",
types: 'place',
countries: 'de',
language: 'de-DE',
placeholder: 'Neue Suche starten',
limit: 5
});
React.useEffect(() => {
// Load MapBox API
map = new mapboxgl.Map({
accessToken: "MYTOKEN",
container: 'map',
style: 'mapbox://styles/mapbox/streets-v11',
attributionControl: false,
center: [13.381777, 52.531677],
zoom: 8
});
geocoder.addTo("#geocoder")
geocoder.on('result', (e) => {
const result = e.result
map.setCenter({ lng: result.geometry.coordinates[0], lat: result.geometry.coordinates[1] })
getUsersByPlace(result)
});
window.scrollTo(0, 0);
}, []);
}
Current Behavior
The error must not be thrown and Mapbox must load.
This is most likely related to the http
plugin failing to serialize the data being passed from web -> native. The team will be investigating a fix
See: https://developer.mozilla.org/en-US/docs/Web/API/Web_Workers_API/Structured_clone_algorithm
I'm getting the same error when trying to upload a file (FormData).
Example:
const options = {
url: 'https://example.com/my/api',
headers: { 'X-Fake-Header': 'Fake-Value' },
data: FormData,
};
await CapacitorHttp.post(options);
I get the same error even if I set content type header like so: 'Content-Type': 'multipart/form-data'
.
I am getting the same error when I try to download a pdf with window.fetch(pdfurl);
I also ran into this error using window.fetch(new URL(...), ...)
. I had to .toString()
on the URL
.
I also ran into this error while connecting to a remote PouchDB() (https://pouchdb.com/).
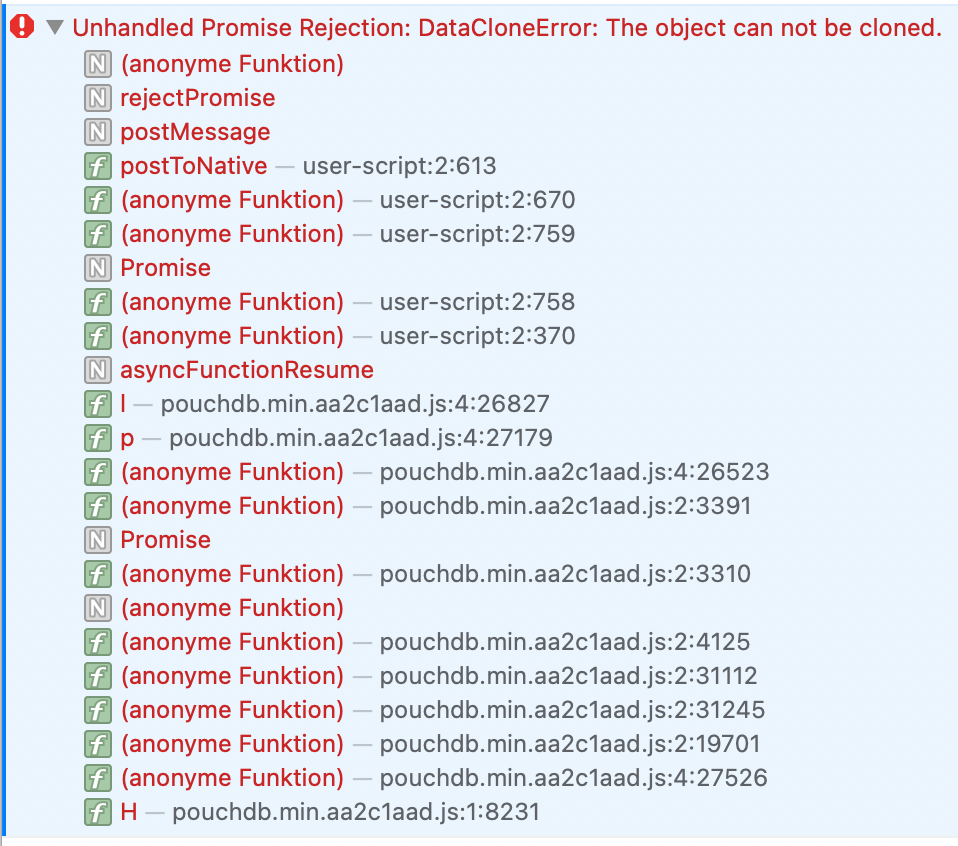
I'm getting the same error when trying to upload a file (FormData).
Example:
const options = { url: 'https://example.com/my/api', headers: { 'X-Fake-Header': 'Fake-Value' }, data: FormData, }; await CapacitorHttp.post(options);
I get the same error even if I set content type header like so:
'Content-Type': 'multipart/form-data'
.
I'm having the same issue... Did you figure out a work-around for this?
I am always having the issue with posting FormData, does anyone have a solution?
I fixed this by providing the URL as a string, rather than a URL object.
(In other words, stick .toString()
on the end.)
Of note, a similar issue happens if you provider headers as a Headers object instead of a plain object. Regular fetch accepts this and I believe this should too. @KevinKelchen so it seems https://github.com/ionic-team/capacitor/issues/6132 and a Headers
object both cause the DataCloneError issue.
Reference: https://developer.mozilla.org/en-US/docs/Web/API/Headers
This will be probably solved when PR https://github.com/ionic-team/capacitor/pull/6206 is merged
Hit this with 5.3.0.
[Error] Unhandled Promise Rejection: DataCloneError: The object can not be cloned.
postMessage
postToNative (user-script:2:753)
(anonymous function) (user-script:2:810)
(anonymous function) (user-script:2:899)
Promise
(anonymous function) (user-script:2:898)
(anonymous function) (user-script:2:439)
as part of OIDC token exchange:
[Log] Object (user-script:2, line 298)
callbackId: "126897699"
methodName: "request"
options: Object
data: URLSearchParams {append: function, delete: function, get: function, getAll: function, has: function, …}
dataType: "json"
headers: Object
Accept: "application/json"
Content-Type: "application/x-www-form-urlencoded"
Object Prototype
method: "POST"
url: "https://login.local.dev/connect/token"
Object Prototype
pluginId: "CapacitorHttp"
type: "message"
Object Prototype
https://github.com/VQComms/oidc-client-ts/blob/main/src/TokenClient.ts#L177
which uses
https://github.com/VQComms/oidc-client-ts/blob/main/src/JsonService.ts#L129
which passes url
as a string already
Ok it seems the Capacitor Http plugin can't handle URLSearchParams
as a body. You have to turn it to a string
with a toString()
call.
The official docs suggest body
can be of this type?
https://developer.mozilla.org/en-US/docs/Web/API/Request/Request
Any body that you want to add to your request: this can be a [Blob](https://developer.mozilla.org/en-US/docs/Web/API/Blob), an [ArrayBuffer](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/ArrayBuffer), a [TypedArray](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray), a [DataView](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/DataView), a [FormData](https://developer.mozilla.org/en-US/docs/Web/API/FormData), a [URLSearchParams](https://developer.mozilla.org/en-US/docs/Web/API/URLSearchParams), a string, or a [ReadableStream](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream) object. Note that a request using the GET or HEAD method cannot have a body.
JSON.parse(JSON.stringify(object))
Parsing and objects in formdata through the above solved this problem for me
can't reproduce on Capacitor 5.7.0, so it was probably fixed when https://github.com/ionic-team/capacitor/pull/6206 got merged as a comment pointed out
Ok it seems the Capacitor Http plugin can't handle
URLSearchParams
as a body. You have to turn it to astring
with atoString()
call.The official docs suggest
body
can be of this type?https://developer.mozilla.org/en-US/docs/Web/API/Request/Request
Any body that you want to add to your request: this can be a [Blob](https://developer.mozilla.org/en-US/docs/Web/API/Blob), an [ArrayBuffer](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/ArrayBuffer), a [TypedArray](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray), a [DataView](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/DataView), a [FormData](https://developer.mozilla.org/en-US/docs/Web/API/FormData), a [URLSearchParams](https://developer.mozilla.org/en-US/docs/Web/API/URLSearchParams), a string, or a [ReadableStream](https://developer.mozilla.org/en-US/docs/Web/API/ReadableStream) object. Note that a request using the GET or HEAD method cannot have a body.
@jcesarmobile or everyone coming across this now:
The above still seems to be an issue. Meaning with version 5.7.2
I am still getting the DataCloneError
when sending an URLSearchParams
object as the body with content type application/x-www-form-urlencoded
.
Passing the parameters via toString()
call as suggested works without issue though.
Thanks for the issue! This issue is being locked to prevent comments that are not relevant to the original issue. If this is still an issue with the latest version of Capacitor, please create a new issue and ensure the template is fully filled out.