reactdatagrid
reactdatagrid copied to clipboard
Inline edit with cell navigation is not working
- what edition are you using - enterprise
- version for
@inovua/reactdatagrid-enterprise
- 4.1.24
Relevant code or config
import React, { useState, useCallback } from 'react'
import ReactDataGrid from '@inovua/reactdatagrid-enterprise'
import '@inovua/reactdatagrid-enterprise/index.css'
const gridStyle = { minHeight: 550 };
const columns = [
{ name: 'id', header: 'Id', defaultVisible: false, minWidth: 300, type: 'number' },
{ name: 'name', header: 'Name', defaultFlex: 1, minWidth: 250 },
{ name: 'country', header: 'Country', defaultFlex: 1, minWidth: 100 },
{ name: 'city', header: 'City', defaultFlex: 1, minWidth: 300 },
{ name: 'age', header: 'Age', minWidth: 150, type: 'number' }
];
const App = () => {
const [dataSource, setDataSource] = useState([{
id: 1,
name: 'Bob',
country: 'uk',
city: 'London',
age: 5
}, {
id: 2,
name: 'Alice',
country: 'uk',
city: 'London',
age: 5
}]);
const onEditComplete = useCallback(({ value, columnId, rowIndex }) => {
const data = [...dataSource];
data[rowIndex][columnId] = value;
setDataSource(data);
}, [dataSource])
const [activeCell, setActiveCell] = useState([0,0])
return (
<div>
<h3>Grid with inline edit</h3>
<ReactDataGrid
idProperty="id"
style={gridStyle}
onEditComplete={onEditComplete}
enableKeyboardNavigation={true}
editable={true}
columns={columns}
dataSource={dataSource}
activeCell={activeCell}
onActiveCellChange={setActiveCell}
/>
</div>
);
}
export default () => <App />
What you did:
When cell keyboard navigation is enabled and columns are editable the Edit
keyboard shortcut makes the wrong cell editable.
Pressing Ctrl+E
on a highlighted cell always makes the first top cell editable, not the one that is active.
What happened:
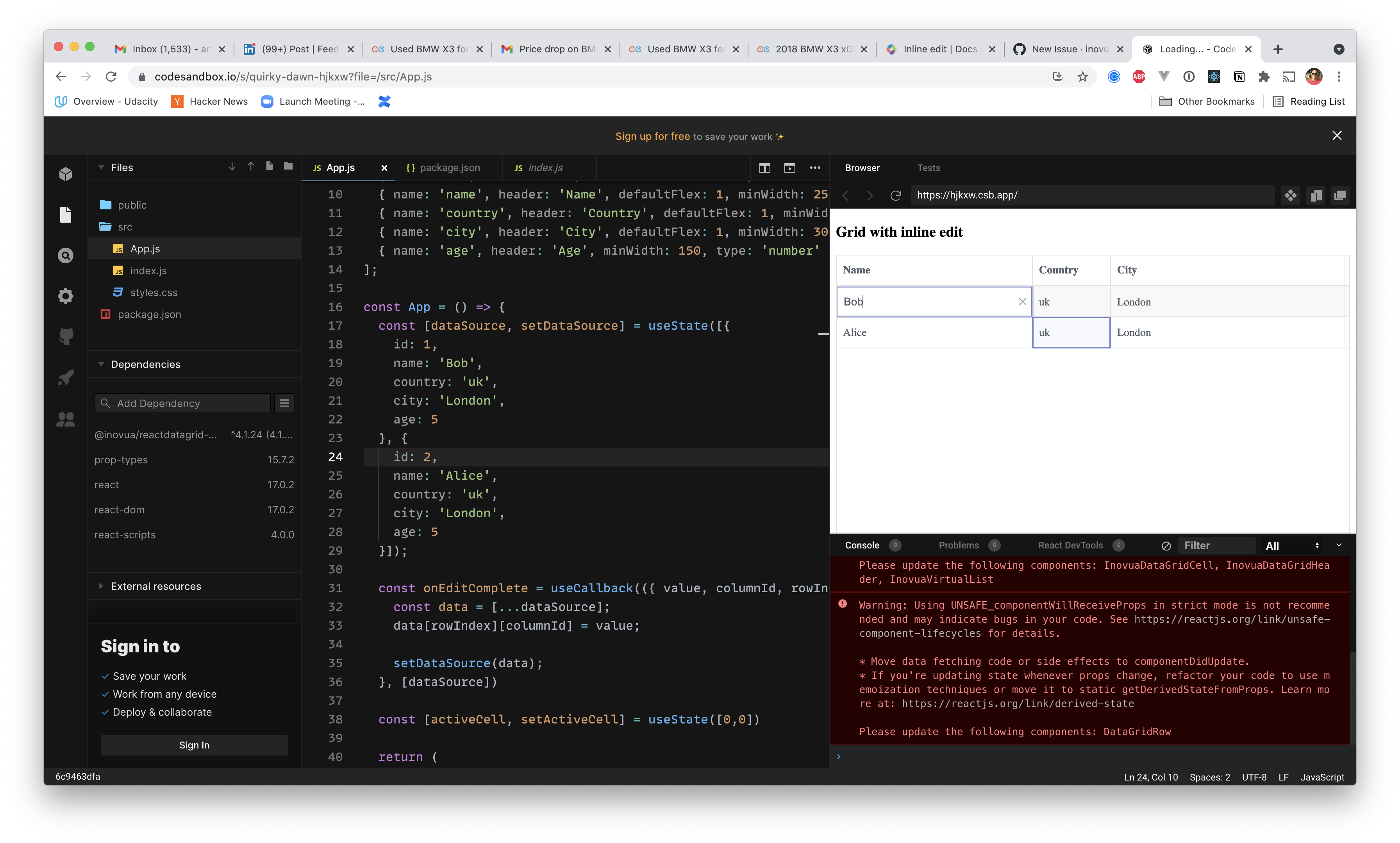
It is expected that the active cell becomes editable when pressing Ctrl+E not the first cell in the first column
Reproduction repository:
https://codesandbox.io/s/quirky-dawn-hjkxw
Problem description:
It is expected that the active cell becomes editable when pressing Ctrl+E not the first cell in the first column
Suggested solution:
I looked at the code and I think that it does not handle shortcuts properly when cell navigation is enabled. It only looks at the activeIndex
not activeCell
property.
I found a workaround for now but I don't think it is pretty. I invoke tryStartEdit right within isStartEditKeyPressed
with the correct rowIndex
and columnId
values
const isStartEditKeyPressed = ({ event, handle: { current: computedProps } }) => {
if (event.key === 'Enter' && !event.shiftKey) {
if (activeCell?.length) {
computedProps.tryStartEdit({
rowIndex: activeCell[0],
columnId: activeCell[1],
dir: 1,
});
}
}
};