fl_chart
fl_chart copied to clipboard
Crashing when loading large data
**Describe
the bug**
The graph freezes when the values entered are too large, ex: R$ 5,314,564.06 this has already happened to you, is there a way to get around this?
Versions
- Flutter => 2.10.5
- FlChart => 0.51.0
Please provide a reproducible code (a main.dart file code)
Hello @imaNNeoFighT, I'm stuck with this problem as well. Here's a reproducible code that you can try.
// This code is distributed under the MIT License.
// Copyright (c) 2018 Felix Angelov.
// You can find the original at https://github.com/felangel/bloc.
import 'package:flutter/material.dart';
import 'package:fl_chart/fl_chart.dart';
void main() {
runApp(App());
}
class App extends StatelessWidget {
const App({super.key});
@override
Widget build(BuildContext context) {
final theme = Theme.of(context);
return LineChart(
LineChartData(
gridData: FlGridData(
show: true,
drawVerticalLine: true,
horizontalInterval: 2,
verticalInterval: 1,
getDrawingHorizontalLine: (value) {
return FlLine(
color: theme.dividerColor,
strokeWidth: 0.8,
);
},
getDrawingVerticalLine: (value) {
return FlLine(
color: theme.dividerColor,
strokeWidth: 0.7,
);
},
),
titlesData: FlTitlesData(
show: true,
rightTitles: AxisTitles(
sideTitles: SideTitles(showTitles: false),
),
topTitles: AxisTitles(
axisNameWidget: Text(
'Population of Pinrang Regency',
style: theme.textTheme.labelLarge?.copyWith(
fontWeight: FontWeight.w600,
),
),
),
bottomTitles: AxisTitles(
axisNameWidget: Text(
'Year',
style: theme.textTheme.labelLarge,
),
sideTitles: SideTitles(
showTitles: true,
reservedSize: 30,
interval: 1,
getTitlesWidget: (value, meta) {
return SideTitleWidget(
axisSide: meta.axisSide,
child: Text(
value.toStringAsFixed(0),
style: theme.textTheme.caption?.copyWith(
fontSize: 12,
fontWeight: FontWeight.w600,
),
),
);
},
),
),
leftTitles: AxisTitles(
axisNameWidget: Text(
'Population',
style: theme.textTheme.labelLarge,
),
sideTitles: SideTitles(
showTitles: true,
interval: 2,
getTitlesWidget: (value, meta) {
return SideTitleWidget(
axisSide: meta.axisSide,
space: 8.0,
child: Text(meta.formattedValue),
);
},
reservedSize: 42,
),
),
),
borderData: FlBorderData(
show: true,
border: Border(
left: BorderSide(
color: theme.dividerColor,
width: 1,
),
bottom: BorderSide(
color: theme.dividerColor,
width: 1,
),
),
),
lineTouchData: LineTouchData(
touchTooltipData: LineTouchTooltipData(
tooltipBgColor: theme.canvasColor,
tooltipRoundedRadius: 12,
getTooltipItems: (spots) {
final textStyle = theme.textTheme.labelLarge?.copyWith(
color: theme.colorScheme.primary,
fontWeight: FontWeight.w400,
) ??
theme.textTheme.bodyMedium!;
return [
LineTooltipItem(
'Year ${spots.first.x.toStringAsFixed(0)}:\n', textStyle,
textAlign: TextAlign.start,
children: [
TextSpan(
text: spots.first.y.toStringAsFixed(0),
style: textStyle.copyWith(
fontWeight: FontWeight.w600,
),
),
TextSpan(
text: ' Population',
)
]),
];
},
),
),
lineBarsData: [
LineChartBarData(
spots: [
FlSpot(2010, 354652),
FlSpot(2011, 360019),
FlSpot(2012, 361293),
FlSpot(2013, 364087),
],
preventCurveOverShooting: true,
isCurved: true,
gradient: LinearGradient(
colors: [
theme.primaryColor,
theme.primaryColorLight,
],
begin: Alignment.centerLeft,
end: Alignment.centerRight,
),
barWidth: 5,
isStrokeCapRound: true,
shadow: Shadow(
color: theme.colorScheme.primary.withOpacity(0.5),
blurRadius: 10,
),
dotData: FlDotData(
show: false,
),
belowBarData: BarAreaData(
show: true,
),
),
],
),
);
}
}
I notice that its only happening when a value inside of FlSpot parameter is greater than 10,000. Once the value goes beyond that limit, the app will crash/not responding. But when the value is less than the limit, the app is working fine and chart can be rendered successfully to the UI.
Hello, the same thing happens to me, is there a solution for this?
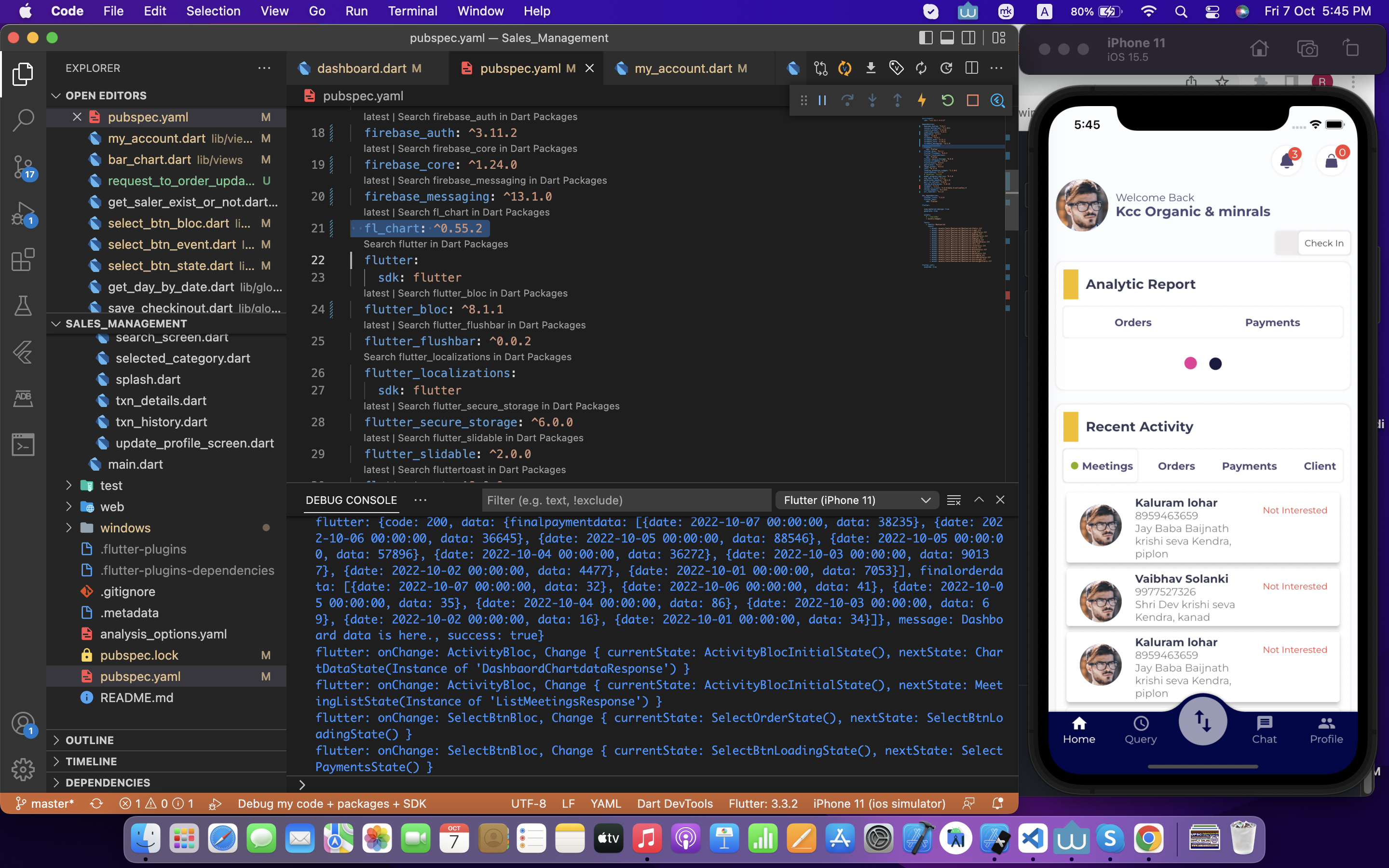
Same is for me i'm shwing large values then my app is crashing/stucking frame
Hi, I am experiencing a similar problem... every time values go higher than 1 Million, the app runs extremely slow, every time the mouse hover by the dot it just freezes for a while and then shows the indicator
Hi, i have the same problem too on my website, just 1440 values make my website freeze a lot when the mouse is over the chart.
Same problem here. My financial app have large money data and it crash my app. Any solution?
Mesmo problema aqui. Meu aplicativo financeiro tem grandes dados de dinheiro e trava meu aplicativo. Qualquer solução?
I had this same problem, when the values arrived in the millions, and it generated the graph, when I clicked to check the points, the app crashes, it's not a fixed solution, it's just to get out of the choke, what I did was, before to generate the graphs I took the value, and added it in two lists, in the first list I added the correct/real value, in the other I took it and divided it by a constant that gives a value ex: 1000, then with the values that were divided I generated the graph, and with the real values, I used it to visualize the points, when the user clicks on the point, he will not see the value that the graph was generated, but the real value that I saved, then it is necessary put an identifier on each result to be able to verify. that took me out of the choke,