react-date-range
react-date-range copied to clipboard
How can we not have initial selection in DateRange?
I am trying to use a DateRange component and I would like to have my initial Calendar empty with nothing selected. Once I start clicking on the calendar then I expect the onChange event to be triggered so we can select the ranges. Looks like if I pass dateRange: {startDate: null, endDate: null} it comes up with no selection, but if I click on the calendar, onChange event is not being called. Am I doing something wrong here? Any help would be appreciated. Thanks.
I think in your case, you should also set a key named 'key' to your selection:
import { useState } from 'react';
const [state, setState] = useState([
{
startDate: null,
endDate: null,
key: 'selectionName'
}
]);
<DateRangePicker
onChange={item => setState([item.selectionName])}
showSelectionPreview={true}
moveRangeOnFirstSelection={false}
ranges={state}
direction="horizontal"
/>;
I'm curious about this as well. I only have 1 range and am setting the initial state of startDate
and endDate
to null
while setting a key. I would expect the calendar to initially render with no selection. The initial render shows the current month's dates highlighted as if selected even though startDate
and endDate
are set to null
.
https://codesandbox.io/s/react-date-range-demo-s1tik
I've got the same problem, seems as though there's no way to have an empty selection. You either have:
an empty array in the initial state: throws an exception:
Cannot read property 'color' of undefined
DateRange.eval [as updatePreview]
https://4y5g3.csb.app/node_modules/react-date-range/dist/components/DateRange/index.js:182:43
onPreviewChange
https://4y5g3.csb.app/node_modules/react-date-range/dist/components/DateRange/index.js:209:18
the null
suggestion above which results in the entire month being selected:
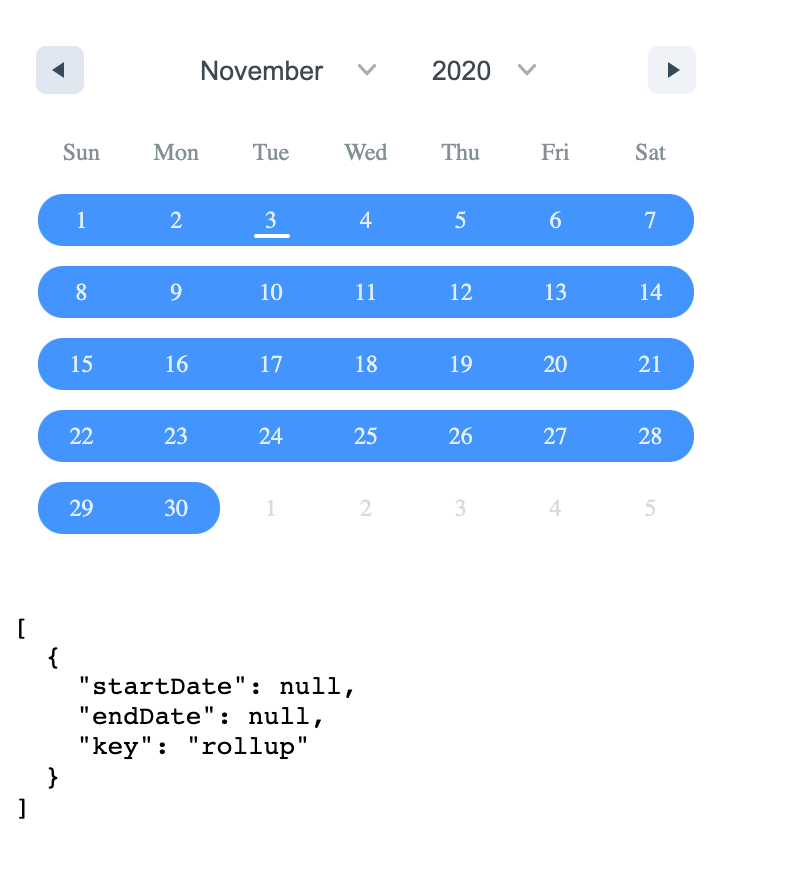
I found a solution. Do not just pass null. Pass it as new Date(null). startDate: new Date(null), endDate: new Date(null).
Very cool, but defaults to Jan 1 1970.
I ended up hacking around this by using:
startDate: new Date(),
endDate: addDays(new Date(), 1),
I too am looking to not have an initial value for the DateRange picker. Can we re-open this issue as it's currently not resolved?
I'm also facing this issue.
There is currently no way to not have an initial value for the DateRange picker. This issue should not have been closed.
I found a workaround. If you have multiple ranges in your initial state object just leave out the range which you do not want to define in the calendar.
Instead of:
const [ranges, setRanges] = useState({
selection: {
startDate: new Date(),
endDate: subDays(new Date(), 7),
key: 'selection',
},
compare: {
startDate: new Date(),
endDate: subDays(new Date(), 7),
key: 'compare',
},
});
Leave out compare:
const [ranges, setRanges] = useState({
selection: {
startDate: new Date(),
endDate: subDays(new Date(), 7),
key: 'selection',
},
});
And then in the onChange handler add the missing range in the state object and it should appear on the calendar.
Note: I am using custom date display inputs instead of default ones, so I am not sure if this will work but maybe the idea will help.
ranges
expects an array, are you using that ranges
var directly in the DatePicker
?
I played around with the codesandbox in the comment above and couldn't get it to work that way. Curious if you would have any luck with that approach there @icelic ?
I had multiple ranges and I wanted the second one not to be selected by default, but I am not sure if that can be applied to this case/sandobx.
any new updates? i'm also facing same issue @onurkerimov
how to show picker without a range selected? set start/end as null, its selecting all days
Any update for this issue?
This shouldn't require "hacking" for a solution. Should be fixed asap
Any update for this issue?
Hey guys...Try this
startDate : null
endDate : new Date("")
key : 'selection'
Hey guys...Try this
startDate : null endDate : new Date("") key : 'selection'
It works, but this is a workaround, maybe the date range should have an empty state
Hey guys...Try this
startDate : null endDate : new Date("") key : 'selection'
It works, but this is a workaround, maybe the date range should have an empty state
Yes ofc....it should support that.
why is it closed? The problem is still not resolved
hi, are there any plans to solve this anytime soon?
It should be the default non-selected state. Any updates?
Same problem here
I have the same issue
I found a "fix" for this, but I updated the source code to accomplish this and I don't know if it can affect the functionality of other components:
I just set the startDate
and the endDate
to null and to avoid errors with that, on the DayCell
component I added this condition:
const inRanges = ranges.reduce((result, range) => {
let startDate = range.startDate;
let endDate = range.endDate;
+ if (!startDate || !endDate) return result;
if (startDate && endDate && isBefore(endDate, startDate)) {
[startDate, endDate] = [endDate, startDate];
}
startDate = startDate ? endOfDay(startDate) : null;
endDate = endDate ? startOfDay(endDate) : null;
const isInRange =
(!startDate || isAfter(day, startDate)) && (!endDate || isBefore(day, endDate));
const isStartEdge = !isInRange && isSameDay(day, startDate);
const isEndEdge = !isInRange && isSameDay(day, endDate);
if (isInRange || isStartEdge || isEndEdge) {
return [
...result,
{
isStartEdge,
isEndEdge: isEndEdge,
isInRange,
...range,
},
];
}
return result;
}, []);
@hugo-licon I do what you've mentioned but without success ! Any ideas to solve this? Thx !
Any fixes for this ?
the problem is still live and kicking
Any updates?
Any updates about that?
+1 on this one. Currently had to resort to setting the endDate to be invalid as @davisraimon has suggested, but i really do not like this workaround. :/