gocv
gocv copied to clipboard
Memory leak when using OpenVino
Tried to use OpenVino as a backend type. All works fine except a memory leak:
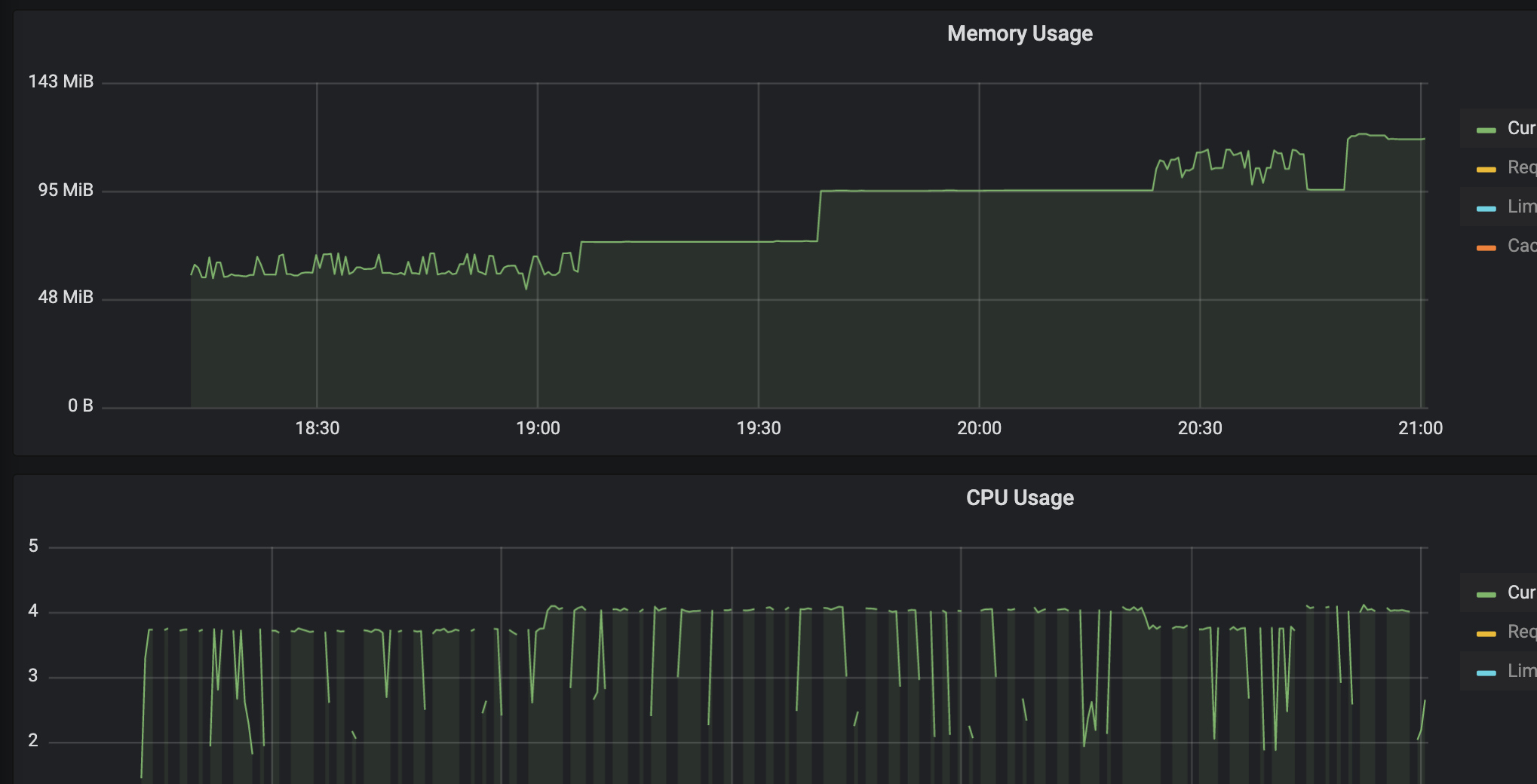
Code that leaks: I'm reading an image in an infinite loop and doing "forward" on it.
package main
import (
"fmt"
"gocv.io/x/gocv"
"image"
"time"
)
func main() {
net := gocv.ReadNet("face-detection-retail-0005.bin", "face-detection-retail-0005.xml")
if net.Empty() {
fmt.Println("Error reading network model")
return
}
net.SetPreferableBackend(gocv.NetBackendOpenVINO)
net.SetPreferableTarget(gocv.NetTargetCPU)
defer net.Close()
for {
img := gocv.IMRead("photo.jpg", gocv.IMReadColor)
blob := gocv.BlobFromImage(img, 1, image.Pt(300, 300), gocv.Scalar{},false, false)
net.SetInput(blob, "")
t1 := time.Now()
prob := net.Forward("")
fmt.Println("Inference time:", time.Since(t1))
for i := 0; i < prob.Total(); i += 7 {
confidence := prob.GetFloatAt(0, i+2)
if confidence > 0.5 {
_ = int(prob.GetFloatAt(0, i+3) * float32(img.Cols()))
_ = int(prob.GetFloatAt(0, i+4) * float32(img.Rows()))
_ = int(prob.GetFloatAt(0, i+5) * float32(img.Cols()))
_ = int(prob.GetFloatAt(0, i+6) * float32(img.Rows()))
}
}
blob.Close()
prob.Close()
img.Close()
}
}
go version go1.13.5 linux/amd64 gocv commit: 2d8275b67baa022d6abeda69508821f6b1db015c