hasura-actions-examples
hasura-actions-examples copied to clipboard
signup "message": "the key 'message' was not present
const fetch = require("node-fetch");
const bcrypt = require("bcryptjs");
const jwt = require("jsonwebtoken");
const HASURA_OPERATION = `
mutation ($username: String!, $password: String!) {
insert_user_one(object: {
username: $username,
password: $password,
}) {
id
}
}
`;
// execute the parent mutation in Hasura
const execute = async (variables, reqHeaders) => {
const fetchResponse = await fetch("http://localhost:8080/v1/graphql", {
method: "POST",
headers: reqHeaders || {},
body: JSON.stringify({
query: HASURA_OPERATION,
variables,
}),
});
return await fetchResponse.json();
};
// Request Handler
const handler = async (req, res) => {
// get request input
const { username, password } = req.body.input;
// run some business logic
const salt = bcrypt.genSaltSync();
let hashedPassword = await bcrypt.hash(password, salt);
// execute the Hasura operation
const { data, errors } = await execute(
{ username, password: hashedPassword },
req.headers
);
// if Hasura operation errors, then throw error
if (errors) {
return res.status(400).json({
message: errors.message,
});
}
const tokenContents = {
sub: data.insert_user_one.id.toString(),
name: username,
iat: Date.now() / 1000,
"https://hasura.io/jwt/claims": {
"x-hasura-allowed-roles": ["user", "anonymous"],
"x-hasura-user-id": data.insert_user_one.id.toString(),
"x-hasura-default-role": "user",
"x-hasura-role": "user",
},
exp: Math.floor(Date.now() / 1000) + 24 * 60 * 60,
};
const token = jwt.sign(tokenContents, process.env.AUTH_PUBLIC_KEY);
// success
return res.json({
...data.insert_user_one,
token: token,
});
};
module.exports = handler;
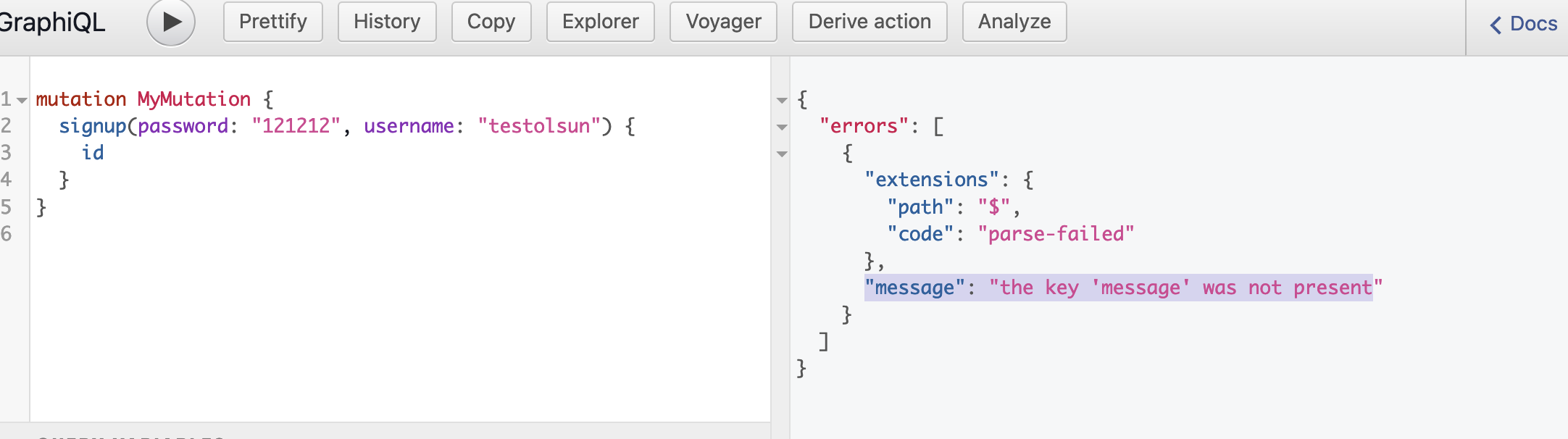
Errors might be an array: https://github.com/hasura/hasura-actions-examples/blob/master/auth/functions/nodejs-express/src/handlers/signup.js#L49
@mkalayci35 Error is an array. Please try this.
if (errors) { return res.json({ message: errors[0].message, code: 400 }) }
@alipetarian Please set status 400 as well
I faced this issue when I directed my Action's handler to my handler running on my localhost. Deploying my handler worked for me. Hope it helps.