remote-config
remote-config copied to clipboard
A remote library to config variables, appearance and behavior of your app without publishing an app update
Android Remote Config Library
A cool alternative for Google firebase remote-config library! Remote config the variables, appearance and the behavior of your app without publishing an app update. The library is based on Google Volley library.
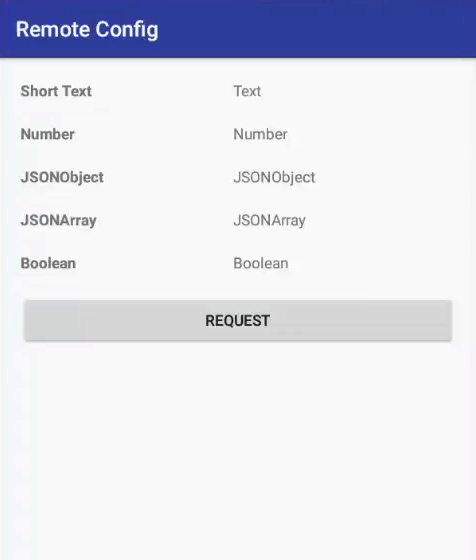
Library retrieves the JSON file from https://github.com/gayanvoice/android-remote-config-library/blob/master/remote-config.json.
To configure the data in the JSON file, you can fork
the library and change the data in the JSON file.
Get
Gradle
- Add this to
build.gradle
of project gradle dependency
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
- Add this to
build.gradle
of app gradle dependency
dependencies {
implementation 'com.github.gayanvoice:android-remote-config-library-firebase:1.0.3'
}
Or
Maven
- Add this to
build.gradle
of project gradle dependency
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
- Add this to
build.gradle
of module gradle dependency
<dependency>
<groupId>com.github.gayanvoice</groupId>
<artifactId>remote-config</artifactId>
<version>1.0.3</version>
</dependency>
Usage
Set internet permission
<uses-permission android:name="android.permission.INTERNET" />
Java
Import remote config library
import com.remoteconfig.library.*;
Set request
// set request
RequestQueue queue = FetchRemote.newRequestQueue(MainActivity.this);
// url of the json file
String url ="https://raw.githubusercontent.com/gayanvoice/remote-config/master/remote-config.json";
// request the json file
RemoteConfig remoteConfig = new RemoteConfig(MainActivity.this, url,
new Response.Listener<String>() {
@Override
public void onComplete() {
// json file retrieved
// declare remote param
RemoteParams remoteParams = new RemoteParams(MainActivity.this);
// get String value
String stringValue = remoteParams.getString("short_text", "default_text");
// get int values
int intValue = remoteParams.getInt("number", 200);
// get JSON Object
JSONObject jsonObject = remoteParams.getJSONObject("json_object");
// get JSON Array
JSONArray jsonArray = remoteParams.getJSONArray("json_array");
// get boolean value
boolean booleanValue = remoteParams.getBoolean("boolean", false);
}
},
new Response.ErrorListener() {
@Override
public void onError(RemoteError error) {
// json file retrieve error
}
}
);
// clear cache
remoteConfig.setShouldCache(false);
queue.add(remoteConfig);
Kotlin
Import remote config library
import com.remoteconfig.library.*
Set request
// set request
val queue = FetchRemote.newRequestQueue(this@MainActivity)
// url of the json file
val url = "https://raw.githubusercontent.com/gayanvoice/remote-config/master/remote-config.json"
// request the json file
val remoteConfig = RemoteConfig(this@MainActivity, url,
Response.Listener<String> {
// json file retrieved
// declare remote param
val remoteParams = RemoteParams(this@MainActivity)
// get String value
val stringValue = remoteParams.getString("short_text", "default_text")
// get int values
val intValue = remoteParams.getInt("number", 200)
// get JSON Object
val jsonObject = remoteParams.getJSONObject("json_object")
// get JSON Array
val jsonArray = remoteParams.getJSONArray("json_array")
// get boolean value
val booleanValue = remoteParams.getBoolean("boolean", false)
},
Response.ErrorListener {
// json file retrieve error
}
)
// clear cache
remoteConfig.setShouldCache(false)
queue.add(remoteConfig)
Develop the library
- Select
Git
fromCheck out project from Version Control
in your Android Studio - Paste the repository url and click
Clone
button - Click
Yes
to open the repository -
Build
using the latestGradle
version
Go to https://github.com/gayanvoice/android-vpn-client-ics-openvpn#develop see the steps