react-native-oauth
react-native-oauth copied to clipboard
some requested scopes were invalid
The README says:
manager.authorize('google', {scopes: 'profile,email'}
but this gives the error 'some requested scopes were invalid'
using the following works so I will raise a PR on the README
manager.authorize('google', {scopes: 'profile email'}
Maybe setting the credential's scopes into fully qualified URL separated by '+' will work for you:
google: {
...,
scopes: {
'https://www.googleapis.com/auth/plus.login+' +
'https://www.googleapis.com/auth/calendar+' +
'https://www.googleapis.com/auth/calendar.readonly+' +
'https://www.googleapis.com/auth/contacts+' +
'https://www.googleapis.com/auth/contacts.readonly+' +
'https://www.googleapis.com/auth/userinfo.profile+' +
'https://www.googleapis.com/auth/userinfo.email+' +
'https://www.googleapis.com/auth/user.addresses.read+' +
'https://www.googleapis.com/auth/user.birthday.read+' +
'https://www.googleapis.com/auth/user.emails.read+' +
'https://www.googleapis.com/auth/user.phonenumbers.read'
}
}
In my case I have another error appearing after validating the scopes hand check.
with:
OAuth.authorize( 'google', { scopes: 'profile+email' } )
.then( ( oauthCredit:Object ):void => {
console.log( oauthCredit ); // <-- status 'ok', the token is received.
const token:string = oauthCredit.response.credentials.accessToken;
const url:string = 'https://www.googleapis.com/oauth2/v1/userinfo';
//const url:string = 'https://www.googleapis.com/plus/v1/people/me'; // <-- either with this WS and without parameters
OAuth.makeRequest( 'google', url, {
method : 'get',
params : {
alt : 'json',
access_token : token
}
}
)
.then( ( rep:Object ):void => {
console.log( rep ); // <-- crash before arriving here
});
});
Everything works fine on iOS simulator but on the device I've got in XCode logs: { status: 'error', msg: 'The operation couldn’t be completed. (NSURLErrorDomain error -1012.)' }
Is anyone facing the same issue?
Ok I fixed that issue, the : NSURLErrorDomain error -1012 mean error 401, when the scopes get updates (one scope more or one scope less) the token is invalidated, it needs to be reseted and assigned again.
Couldn't make this work on android, invalid_scope
error
manager.authorize('google', {scopes: 'email,profile'})
.then(resp => console.log(resp))
.catch(err => console.log(err));
Try {scopes: 'email profile'} i.e. without the comma. I've updated the README to correct this Martin
Sent from my iPhone
On 10 Feb 2017, at 11:35, EL YOUBI [email protected] wrote:
Couldn't make this work on android, invalid_scope error
manager.authorize('google', {scopes: 'email,profile'}) .then(resp => console.log(resp)) .catch(err => console.log(err)); — You are receiving this because you authored the thread. Reply to this email directly, view it on GitHub, or mute the thread.
For me it works with {scopes: 'email+profile'}.
{scopes: 'email profile'} didn't work, {scopes: 'email+profile'} worked. Thanks @buskerone
Submitted #121 for this issue
neither {scopes: 'email+profile'} nor {scopes: 'email,profile'} nor {scopes: 'email profile'} worked for me. Though doing just {scopes: 'email'} or {scopes: 'profile'} did work for me.
I am getting this error too, no matter what I use as a delimiter. Single scopes work fine but that is no help to me.
Not sure if someone has a better solution or a newer fork, but here's what seemed to work for me:
TL;DR For android use the rawScopes: "true"
in your config and use space as the delimiter, for iOS use +
as the delimiter. Note that for the android config, "true"
is a string not a boolean.
let config
let scopes
if (Platform.OS === 'ios') {
config = {
google: {
client_id: '...',
callback_url: '...',
},
}
scopes = 'openid+email+profile'
} else if (Platform.OS === 'android') {
config = {
google: {
client_id: '...',
client_secret: '...',
callback_url: 'http://localhost/google',
rawScopes: 'true',
},
}
scopes = 'openid email profile'
}
manager.configure(config)
// ...
manager.authorize('google', { scopes })
@maxlang The error on Android while using "react-native-oauth": "^2.2.0"
. Do you encounter it?
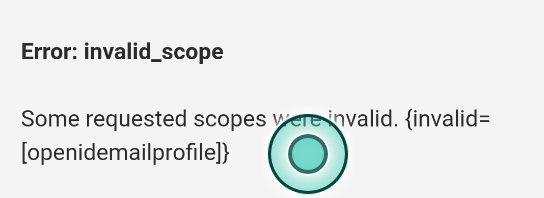
@haotangio I did originally, but worked around it using the code above. Did you try using rawScopes: 'true'
?
As for version, I'm actually using https://github.com/exentrich/react-native-oauth to work around some other issues I was having, but the android code should be the same I think.
@maxlang I did try rawScopes: 'true'
before posting the previous comment.
However, the issue was still there.
Ok, it looks like the change to support rawScopes was never officially "released" as a version.
Try the following:
npm uninstall --save react-native-ouath
npm install --save fullstackreact/react-native-oauth
This should update you to master.
Make sure rawScopes
appears in .../node_modules/react-native-oauth/android/src/main/java/io/fullstack/oauth/OAuthManagerProviders.java
as it does here: https://github.com/fullstackreact/react-native-oauth/blob/master/android/src/main/java/io/fullstack/oauth/OAuthManagerProviders.java#L271
@maxlang Thank you so much for your information. I will try soon. I guess it will work.
Leaving it here for the Google Search :tm: users:
https://developers.google.com/identity/protocols/oauth2/scopes
Make sure you have the right scopes enabled in the project. For e.g., to get https://www.googleapis.com/auth/userinfo.email
you need to enable https://console.cloud.google.com/apis/library/script.googleapis.com
you can also use the scopes in this way. create a route like this and pass in the parameters in the passport.authenticate middleware router.get('/users/auth/google',passport.authenticate('google',{scope:['profile','email']}));