flutter
flutter copied to clipboard
[mobile] Selected DropdownMenuItem isn't highlighted on mobile only
Steps to Reproduce
- Run the code following on the mobile simulator or physical mobile device
code sample
/// Flutter code sample for DropdownButton
// @dart = 2.9
// This sample shows a `DropdownButton` with a large arrow icon,
// purple text style, and bold purple underline, whose value is one of "One",
// "Two", "Free", or "Four".
//
// 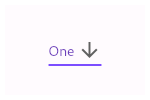
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
/// This is the main application widget.
class MyApp extends StatelessWidget {
static const String _title = 'Flutter Code Sample';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: Center(
child: MyStatefulWidget(),
),
),
);
}
}
/// This is the stateful widget that the main application instantiates.
class MyStatefulWidget extends StatefulWidget {
MyStatefulWidget({Key key}) : super(key: key);
@override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
/// This is the private State class that goes with MyStatefulWidget.
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
String dropdownValue = 'One';
@override
Widget build(BuildContext context) {
return DropdownButton<String>(
value: dropdownValue,
icon: Icon(Icons.arrow_downward),
iconSize: 24,
elevation: 16,
style: TextStyle(color: Colors.deepPurple),
underline: Container(
height: 2,
color: Colors.deepPurpleAccent,
),
onChanged: (String newValue) {
setState(() {
dropdownValue = newValue;
});
},
items: <String>[
'One',
'Two',
'Free',
'Four',
'Five',
'Six',
'Seven',
'Eight',
'Nine',
'Ten',
'Eleven',
'Twelve',
'Thirteen',
'Fourteen',
'Fifteen',
'Sixteen',
'Eighteen',
'Nineteen',
'Twenty',
'Twenty One',
'Twenty Two',
'Twenty Three',
'Twenty Four',
'Twenty Five'
].map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
);
}
}
- Select an item and then close the dropdown menu, open it again, selected isn't highlighted
Preview
Mobile | Web & Desktop |
---|---|
![]() |
![]() |
Additional context:
- Selected item is correctly highlighted on the web and desktop using the same code sample.
- Issue reproduced on
stable
,beta
,master
flutter doctor -v
[✓] Flutter (Channel stable, 1.22.3, on Mac OS X 10.15.7 19H15, locale en-GB)
• Flutter version 1.22.3 at /Users/tahatesser/Code/flutter_stable
• Framework revision 8874f21e79 (13 days ago), 2020-10-29 14:14:35 -0700
• Engine revision a1440ca392
• Dart version 2.10.3
[✓] Android toolchain - develop for Android devices (Android SDK version 30.0.2)
• Android SDK at /Users/tahatesser/Code/sdk
• Platform android-30, build-tools 30.0.2
• ANDROID_HOME = /Users/tahatesser/Code/sdk
• Java binary at: /Applications/Android
Studio.app/Contents/jre/jdk/Contents/Home/bin/java
• Java version OpenJDK Runtime Environment (build
1.8.0_242-release-1644-b3-6915495)
• All Android licenses accepted.
[✓] Xcode - develop for iOS and macOS (Xcode 12.2)
• Xcode at /Volumes/SanDisk/Xcode-beta.app/Contents/Developer
• Xcode 12.2, Build version 12B5044c
• CocoaPods version 1.10.0
[!] Android Studio (version 4.1)
• Android Studio at /Applications/Android Studio.app/Contents
✗ Flutter plugin not installed; this adds Flutter specific functionality.
✗ Dart plugin not installed; this adds Dart specific functionality.
• Java version OpenJDK Runtime Environment (build
1.8.0_242-release-1644-b3-6915495)
[✓] VS Code (version 1.51.0)
• VS Code at /Applications/Visual Studio Code.app/Contents
• Flutter extension version 3.16.0
[✓] Connected device (2 available)
• RMX2001 (mobile) • EUYTFEUSQSRGDA6D • android-arm64 • Android 10
(API 29)
• Taha’s iPad (mobile) • 00008020-000255113EE8402E • ios • iOS 14.2
! Doctor found issues in 1 category.
[✓] Flutter (Channel beta, 1.23.0-18.1.pre, on Mac OS X 10.15.7 19H15 x86_64, locale
en-GB)
• Flutter version 1.23.0-18.1.pre at /Users/tahatesser/Code/flutter_beta
• Framework revision 198df796aa (4 weeks ago), 2020-10-15 12:04:33 -0700
• Engine revision 1d12d82d9c
• Dart version 2.11.0 (build 2.11.0-213.1.beta)
[✓] Android toolchain - develop for Android devices (Android SDK version 30.0.2)
• Android SDK at /Users/tahatesser/Code/sdk
• Platform android-30, build-tools 30.0.2
• ANDROID_HOME = /Users/tahatesser/Code/sdk
• Java binary at: /Applications/Android
Studio.app/Contents/jre/jdk/Contents/Home/bin/java
• Java version OpenJDK Runtime Environment (build
1.8.0_242-release-1644-b3-6915495)
• All Android licenses accepted.
[✓] Xcode - develop for iOS and macOS (Xcode 12.2)
• Xcode at /Volumes/SanDisk/Xcode-beta.app/Contents/Developer
• Xcode 12.2, Build version 12B5044c
• CocoaPods version 1.10.0
[✓] Chrome - develop for the web
• Chrome at /Applications/Google Chrome.app/Contents/MacOS/Google Chrome
[✓] Android Studio (version 4.1)
• Android Studio at /Applications/Android Studio.app/Contents
• Flutter plugin can be installed from:
🔨 https://plugins.jetbrains.com/plugin/9212-flutter
• Dart plugin can be installed from:
🔨 https://plugins.jetbrains.com/plugin/6351-dart
• Java version OpenJDK Runtime Environment (build
1.8.0_242-release-1644-b3-6915495)
[✓] VS Code (version 1.51.0)
• VS Code at /Applications/Visual Studio Code.app/Contents
• Flutter extension version 3.16.0
[✓] Connected device (5 available)
• RMX2001 (mobile) • EUYTFEUSQSRGDA6D • android-arm64 •
Android 10 (API 29)
• Taha’s iPad (mobile) • 00008020-000255113EE8402E • ios •
iOS 14.2
• iPhone 11 (mobile) • FF1F0A30-1571-44C9-B79D-E18CE0622B1B • ios •
com.apple.CoreSimulator.SimRuntime.iOS-14-2 (simulator)
• Web Server (web) • web-server • web-javascript •
Flutter Tools
• Chrome (web) • chrome • web-javascript •
Google Chrome 86.0.4240.193
• No issues found!
[✓] Flutter (Channel master, 1.24.0-8.0.pre.194, on Mac OS X 10.15.7 19H15
darwin-x64, locale en-GB)
• Flutter version 1.24.0-8.0.pre.194 at /Users/tahatesser/Code/flutter_master
• Framework revision 018467cdb1 (8 hours ago), 2020-11-11 02:04:03 -0500
• Engine revision 81f219c59c
• Dart version 2.12.0 (build 2.12.0-31.0.dev)
[✓] Android toolchain - develop for Android devices (Android SDK version 30.0.2)
• Android SDK at /Users/tahatesser/Code/sdk
• Platform android-30, build-tools 30.0.2
• ANDROID_HOME = /Users/tahatesser/Code/sdk
• Java binary at: /Applications/Android
Studio.app/Contents/jre/jdk/Contents/Home/bin/java
• Java version OpenJDK Runtime Environment (build
1.8.0_242-release-1644-b3-6915495)
• All Android licenses accepted.
[✓] Xcode - develop for iOS and macOS (Xcode 12.2)
• Xcode at /Volumes/SanDisk/Xcode-beta.app/Contents/Developer
• Xcode 12.2, Build version 12B5044c
• CocoaPods version 1.10.0
[✓] Chrome - develop for the web
• Chrome at /Applications/Google Chrome.app/Contents/MacOS/Google Chrome
[✓] Android Studio (version 4.1)
• Android Studio at /Applications/Android Studio.app/Contents
• Flutter plugin can be installed from:
🔨 https://plugins.jetbrains.com/plugin/9212-flutter
• Dart plugin can be installed from:
🔨 https://plugins.jetbrains.com/plugin/6351-dart
• Java version OpenJDK Runtime Environment (build
1.8.0_242-release-1644-b3-6915495)
[✓] VS Code (version 1.51.0)
• VS Code at /Applications/Visual Studio Code.app/Contents
• Flutter extension version 3.16.0
[✓] Connected device (6 available)
• RMX2001 (mobile) • EUYTFEUSQSRGDA6D • android-arm64 •
Android 10 (API 29)
• Taha’s iPad (mobile) • 00008020-000255113EE8402E • ios •
iOS 14.2
• iPhone 11 (mobile) • FF1F0A30-1571-44C9-B79D-E18CE0622B1B • ios •
com.apple.CoreSimulator.SimRuntime.iOS-14-2 (simulator)
• macOS (desktop) • macos • darwin-x64 •
Mac OS X 10.15.7 19H15 darwin-x64
• Web Server (web) • web-server • web-javascript •
Flutter Tools
• Chrome (web) • chrome • web-javascript •
Google Chrome 86.0.4240.193
• No issues found!
I was able to reproduce. Adding regression label because I'm pretty sure that this worked fine before but can't confirm right now, feel free to remove if it is not.
flutter doctor -v
[✓] Flutter (Channel stable, 1.22.3, on Mac OS X 10.15.7 19H2, locale en)
• Flutter version 1.22.3 at /Users/pedromassango/dev/SDKs/flutter_stable
• Framework revision 8874f21e79 (2 weeks ago), 2020-10-29 14:14:35 -0700
• Engine revision a1440ca392
• Dart version 2.10.3
[✓] Android toolchain - develop for Android devices (Android SDK version 30.0.2)
• Android SDK at /Users/pedromassango/Library/Android/sdk
• Platform android-30, build-tools 30.0.2
• Java binary at: /Applications/Android Studio.app/Contents/jre/jdk/Contents/Home/bin/java
• Java version OpenJDK Runtime Environment (build 1.8.0_242-release-1644-b3-6222593)
• All Android licenses accepted.
[✓] Xcode - develop for iOS and macOS (Xcode 12.1)
• Xcode at /Applications/Xcode.app/Contents/Developer
• Xcode 12.1, Build version 12A7403
• CocoaPods version 1.9.3
[!] Android Studio (version 4.0)
• Android Studio at /Applications/Android Studio.app/Contents
✗ Flutter plugin not installed; this adds Flutter specific functionality.
✗ Dart plugin not installed; this adds Dart specific functionality.
• Java version OpenJDK Runtime Environment (build 1.8.0_242-release-1644-b3-6222593)
[✓] IntelliJ IDEA Community Edition (version 2020.2.3)
• IntelliJ at /Applications/IntelliJ IDEA CE.app
• Flutter plugin installed
• Dart plugin version 202.8070
[✓] VS Code (version 1.51.0)
• VS Code at /Applications/Visual Studio Code.app/Contents
• Flutter extension version 3.16.0
[✓] Connected device (1 available)
• sdk gphone x86 arm (mobile) • emulator-5554 • android-x86 • Android 11 (API 30) (emulator)
! Doctor found issues in 1 category.
This issue is not fixed still
@HansMuller Does material expect the previously selected item to be highlighted for a DropdownMenu? Also, who is our material contact?
This problem has not yet been resolved
Reproducible on stable 3.3 and master 3.7
M2 specs: https://m2.material.io/components/menus#exposed-dropdown-menu
code sample
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
/// This is the main application widget.
class MyApp extends StatelessWidget {
static const String _title = 'Flutter Code Sample';
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const Center(
child: MyStatefulWidget(),
),
),
);
}
}
/// This is the stateful widget that the main application instantiates.
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
/// This is the private State class that goes with MyStatefulWidget.
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
String dropdownValue = 'One';
@override
Widget build(BuildContext context) {
return DropdownButton<String>(
value: dropdownValue,
icon: const Icon(Icons.arrow_downward),
iconSize: 24,
elevation: 16,
style: const TextStyle(color: Colors.deepPurple),
underline: Container(
height: 2,
color: Colors.deepPurpleAccent,
),
onChanged: (String? newValue) {
setState(() {
dropdownValue = newValue!;
});
},
items: <String>[
'One',
'Two',
'Free',
'Four',
'Five',
'Six',
'Seven',
'Eight',
'Nine',
'Ten',
'Eleven',
'Twelve',
'Thirteen',
'Fourteen',
'Fifteen',
'Sixteen',
'Eighteen',
'Nineteen',
'Twenty',
'Twenty One',
'Twenty Two',
'Twenty Three',
'Twenty Four',
'Twenty Five'
].map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
);
}
}
flutter doctor -v (mac)
[✓] Flutter (Channel master, 3.7.0-13.0.pre.131, on macOS 13.1 22C65 darwin-arm64, locale en-IN)
• Flutter version 3.7.0-13.0.pre.131 on channel master at /Users/mahesh/Development/flutter_master
• Upstream repository https://github.com/flutter/flutter.git
• Framework revision 0196e6050b (2 days ago), 2022-12-31 11:10:23 -0500
• Engine revision 932591ec04
• Dart version 3.0.0 (build 3.0.0-76.0.dev)
• DevTools version 2.20.0
• If those were intentional, you can disregard the above warnings; however it is recommended to use "git" directly to perform update checks
and upgrades.
[✓] Android toolchain - develop for Android devices (Android SDK version 33.0.0-rc4)
• Android SDK at /Users/mahesh/Library/Android/sdk
• Platform android-33, build-tools 33.0.0-rc4
• ANDROID_HOME = /Users/mahesh/Library/Android/sdk
• Java binary at: /Applications/Android Studio.app/Contents/jre/Contents/Home/bin/java
• Java version OpenJDK Runtime Environment (build 11.0.12+0-b1504.28-7817840)
• All Android licenses accepted.
[✓] Xcode - develop for iOS and macOS (Xcode 14.0.1)
• Xcode at /Applications/Xcode.app/Contents/Developer
• Build 14A400
• CocoaPods version 1.11.3
[✓] Chrome - develop for the web
• Chrome at /Applications/Google Chrome.app/Contents/MacOS/Google Chrome
[✓] Android Studio (version 2021.2)
• Android Studio at /Applications/Android Studio.app/Contents
• Flutter plugin can be installed from:
🔨 https://plugins.jetbrains.com/plugin/9212-flutter
• Dart plugin can be installed from:
🔨 https://plugins.jetbrains.com/plugin/6351-dart
• Java version OpenJDK Runtime Environment (build 11.0.12+0-b1504.28-7817840)
[✓] IntelliJ IDEA Community Edition (version 2021.2.1)
• IntelliJ at /Applications/IntelliJ IDEA CE.app
• Flutter plugin version 61.2.4
• Dart plugin version 212.5080.8
[✓] VS Code (version 1.74.2)
• VS Code at /Applications/Visual Studio Code.app/Contents
• Flutter extension version 3.56.0
[✓] Connected device (3 available)
• iPhone 12 Pro (mobile) • 026D5789-9E78-4AD5-B1B2-3F8D4E7F65E4 • ios • com.apple.CoreSimulator.SimRuntime.iOS-14-5 (simulator)
• macOS (desktop) • macos • darwin-arm64 • macOS 13.1 22C65 darwin-arm64
• Chrome (web) • chrome • web-javascript • Google Chrome 108.0.5359.124
[✓] HTTP Host Availability
• All required HTTP hosts are available
• No issues found!
[✓] Flutter (Channel stable, 3.3.9, on macOS 12.6 21G115 darwin-arm, locale en-IN)
• Flutter version 3.3.9 on channel stable at /Users/mahesh/Development/flutter
• Upstream repository https://github.com/flutter/flutter.git
• Framework revision b8f7f1f986 (24 hours ago), 2022-11-23 06:43:51 +0900
• Engine revision 8f2221fbef
• Dart version 2.18.5
• DevTools version 2.15.0
[✓] Android toolchain - develop for Android devices (Android SDK version 33.0.0-rc4)
• Android SDK at /Users/mahesh/Library/Android/sdk
• Platform android-33, build-tools 33.0.0-rc4
• ANDROID_HOME = /Users/mahesh/Library/Android/sdk
• Java binary at: /Applications/Android Studio.app/Contents/jre/Contents/Home/bin/java
• Java version OpenJDK Runtime Environment (build 11.0.12+0-b1504.28-7817840)
• All Android licenses accepted.
[✓] Xcode - develop for iOS and macOS (Xcode 14.0.1)
• Xcode at /Applications/Xcode.app/Contents/Developer
• Build 14A400
• CocoaPods version 1.11.3
[✓] Chrome - develop for the web
• Chrome at /Applications/Google Chrome.app/Contents/MacOS/Google Chrome
[✓] Android Studio (version 2021.2)
• Android Studio at /Applications/Android Studio.app/Contents
• Flutter plugin can be installed from:
🔨 https://plugins.jetbrains.com/plugin/9212-flutter
• Dart plugin can be installed from:
🔨 https://plugins.jetbrains.com/plugin/6351-dart
• Java version OpenJDK Runtime Environment (build 11.0.12+0-b1504.28-7817840)
[✓] IntelliJ IDEA Community Edition (version 2021.2.1)
• IntelliJ at /Applications/IntelliJ IDEA CE.app
• Flutter plugin version 61.2.4
• Dart plugin version 212.5080.8
[✓] VS Code (version 1.70.2)
• VS Code at /Applications/Visual Studio Code.app/Contents
• Flutter extension version 3.53.20221101
[✓] Connected device (3 available)
• iPhone 12 Pro (mobile) • 026D5789-9E78-4AD5-B1B2-3F8D4E7F65E4 • ios •
com.apple.CoreSimulator.SimRuntime.iOS-14-5 (simulator)
• macOS (desktop) • macos • darwin-arm64 • macOS 12.6 21G115 darwin-arm
• Chrome (web) • chrome • web-javascript • Google Chrome 107.0.5304.110
[✓] HTTP Host Availability
• All required HTTP hosts are available
• No issues found!
The issue still exists. Has there been any progress?
This issue is missing a priority label. Please set a priority label when adding the triaged-design
label.