DiagonalLayout
DiagonalLayout copied to clipboard
How to implement with collapsing toolbar layout
Can't make it work with collapsing toolbar layout. Here is my code. It is showing usual rectangular size.
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:diagonal="http://schemas.android.com/tools"
android:id="@+id/main_content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true">
<android.support.design.widget.AppBarLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:fitsSystemWindows="true"
android:theme="@style/ThemeOverlay.AppCompat.Dark.ActionBar">
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/collapsing_toolbar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:fitsSystemWindows="true"
app:contentScrim="?attr/colorPrimary"
app:expandedTitleMarginEnd="64dp"
app:expandedTitleMarginStart="48dp"
app:layout_scrollFlags="scroll|exitUntilCollapsed">
<com.github.florent37.diagonallayout.DiagonalLayout
android:id="@+id/diagonalLayout"
android:layout_width="match_parent"
android:layout_height="250dp"
diagonal:diagonal_angle="50"
diagonal:diagonal_direction="right"
diagonal:diagonal_position="bottom"
>
<com.flaviofaria.kenburnsview.KenBurnsView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"
android:src="@drawable/spider_man"
/>
</com.github.florent37.diagonallayout.DiagonalLayout>
<android.support.v7.widget.Toolbar
android:id="@+id/toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:layout_scrollFlags="scroll|enterAlways"/>
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
</android.support.design.widget.CoordinatorLayout>
Hi, i faced the same issue. I propose you this solution :
Layout :
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="false"
android:background="@color/md_grey_50">
<android.support.design.widget.AppBarLayout
android:id="@+id/app_bar"
android:layout_width="match_parent"
android:layout_height="@dimen/app_bar_height"
android:fitsSystemWindows="true"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/toolbar_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
android:background="@color/colorPrimary"
app:statusBarScrim="@color/colorPrimaryDark"
app:layout_scrollFlags="scroll|exitUntilCollapsed">
<!--same backgroundColor as your activity parent Layout -->
<View
android:id="@+id/fakeAppBarBackground"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/md_grey_50"/>
<!-- Diagonal Layout with parallax effect and and ParallaxMultiplier set to 1 to keep it at the bottom when we scroll
In this example, I just set a View with background color, but you can set a ImageView and give it an alpha to 0 according to your scroll (SEE JAVA) -->
<com.github.florent37.diagonallayout.DiagonalLayout
android:fitsSystemWindows="true"
android:id="@+id/diagonalLayout"
android:layout_width="match_parent"
android:layout_height="220dp"
app:diagonal_angle="10"
android:elevation="8dp"
android:paddingBottom="16dp"
app:diagonal_direction="left"
app:layout_collapseMode="parallax"
app:layout_collapseParallaxMultiplier="1"
app:diagonal_position="bottom">
<View
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorPrimary"/>
</com.github.florent37.diagonallayout.DiagonalLayout>
<android.support.v7.widget.Toolbar
android:id="@+id/main_toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:layout_collapseMode="pin"
android:elevation="16dp"
app:popupTheme="@style/AppTheme.PopupOverlay" />
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
<android.support.v4.widget.NestedScrollView
app:layout_behavior="@string/appbar_scrolling_view_behavior"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:behavior_overlapTop="128dp">
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
android:layout_width="match_parent"
android:layout_height="180dp"
android:layout_margin="16dp"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/large_text"/>
</LinearLayout>
</android.support.v4.widget.NestedScrollView>
</android.support.design.widget.CoordinatorLayout>
JAVA in your onCreate :
appBarLayout = (AppBarLayout)findViewById(R.id.app_bar);
collapsingToolbarLayout = (CollapsingToolbarLayout) findViewById(R.id.toolbar_layout);
diagonalLayout = (DiagonalLayout) findViewById(R.id.diagonalLayout);
fakeAppBarBackground = findViewById(R.id.fakeAppBarBackground);
appBarLayout.addOnOffsetChangedListener(new AppBarLayout.OnOffsetChangedListener() {
@Override
public void onOffsetChanged(AppBarLayout appBarLayout, int verticalOffset) {
float offsetAlpha = (appBarLayout.getY() / appBarLayout.getTotalScrollRange());
//diagonalLayout.setAlpha( 1 - (offsetAlpha * -1));
fakeAppBarBackground.setAlpha( 1 - (offsetAlpha * -1));
if (Math.abs(verticalOffset)-appBarLayout.getTotalScrollRange() == 0) { //==0
// Collapsed
}
else {
//Expanded
}
}
});
@GauvainSeigneur can you please provide us the final code which you made the changes and is working fine and shiny? I mean that the code which is hiding the toolbar or something I tried using it but it seems like this and no magic happens:
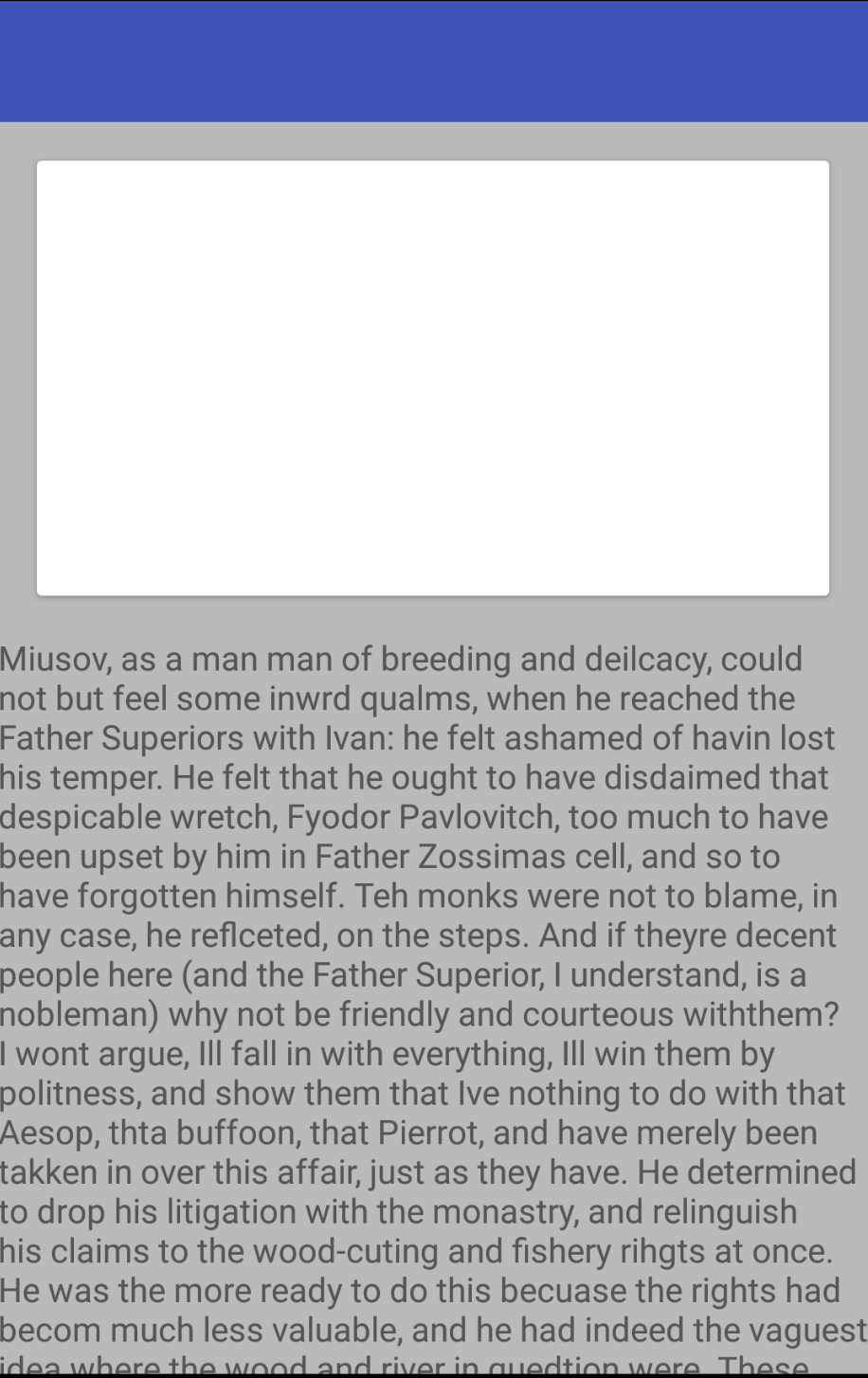
Hi @mori-honest ,
Sorry but all my code is already here... But you can put your code and describe the expected behaviour so we can check it together.
@GauvainSeigneur I'm getting the same result as @mori-honest and I'm using your code, copy-pasted without changes.
Hi, i'm sorry you're right, something wrong with the elevation outlineprovider... so I put it at 0... But try to download lib files and include it directly to your project. I don't know why but it works better.
And , Please try this xml
I will make a little project if you want to this week
<android.support.design.widget.CoordinatorLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="false"
android:background="@color/md_grey_50">
<android.support.design.widget.AppBarLayout
android:id="@+id/app_bar"
android:layout_width="match_parent"
android:layout_height="@dimen/app_bar_height"
android:fitsSystemWindows="true"
android:theme="@style/AppTheme.AppBarOverlay">
<android.support.design.widget.CollapsingToolbarLayout
android:id="@+id/toolbar_layout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:fitsSystemWindows="true"
android:background="@color/colorPrimary"
app:statusBarScrim="@color/colorPrimaryDark"
app:layout_scrollFlags="scroll|exitUntilCollapsed">
<!--same backgroundColor as your activity parent Layout -->
<View
android:id="@+id/fakeAppBarBackground"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/md_grey_50"/>
<!-- Diagonal Layout with parallax effect and and ParallaxMultiplier set to 1 to keep it at the bottom when we scroll
In this example, I just set a View with background color, but you can set a ImageView and give it an alpha to 0 according to your scroll (SEE JAVA) -->
<com.github.florent37.diagonallayout.DiagonalLayout
android:fitsSystemWindows="true"
android:id="@+id/diagonalLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:diagonal_angle="15"
android:elevation="0dp"
android:outlineProvider="none"
app:diagonal_direction="left"
app:layout_collapseMode="parallax"
app:layout_collapseParallaxMultiplier="0.5"
app:diagonal_position="bottom">
<View
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/colorPrimary"/>
</com.github.florent37.diagonallayout.DiagonalLayout>
<android.support.v7.widget.Toolbar
android:id="@+id/main_toolbar"
android:layout_width="match_parent"
android:layout_height="?attr/actionBarSize"
app:layout_collapseMode="pin"
android:elevation="16dp"
app:popupTheme="@style/AppTheme.PopupOverlay" />
</android.support.design.widget.CollapsingToolbarLayout>
</android.support.design.widget.AppBarLayout>
<android.support.v4.widget.NestedScrollView
app:layout_behavior="@string/appbar_scrolling_view_behavior"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<LinearLayout
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/large_text"/>
</LinearLayout>
</android.support.v4.widget.NestedScrollView>
</android.support.design.widget.CoordinatorLayout>
@GauvainSeigneur It works! Thanks for putting your time into this.