habitat-lab
habitat-lab copied to clipboard
[Bugfix] [Rearrange] Reset the sensors positons correctly
Motivation and Context
When resetting a rearrange task, the first observation is taken from the origin instead of the position of the robot. this change addresses the issue.
How to reproduce :
Run this code :
import habitat
import habitat.utils.gym_definitions
import gym
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
mode = "rgb_array"
# mode = "human"
# config_file="/Users/vincentpierre/Documents/habitat-lab/configs/tasks/pointnav.yaml"
config_file="/Users/vincentpierre/Documents/habitat-lab/configs/tasks/rearrange/pick.yaml"
#env = gym.make("HabitatPick-v0")
env = gym.make(
"Habitat-v0",
cfg_file_path=config_file,
use_render_mode=False,
)
#call robot.update()
action = np.array([0.0] * 8, dtype=np.float32)
if isinstance(env.action_space, gym.spaces.Discrete):
action = 0
_=env.reset();reset_frame_0=env.render(mode)
_=env.step(action);step_frame_0=env.render(mode)
_=env.reset();reset_frame_1=env.render(mode)
_=env.step(action);step_frame_1=env.render(mode)
_=env.reset();reset_frame_2=env.render(mode)
_=env.step(action);step_frame_2=env.render(mode)
plt.figure()
#subplot(r,c) provide the no. of rows and columns
f, axarr = plt.subplots(3,2)
axarr[0,0].imshow(reset_frame_0);
axarr[0,1].imshow(step_frame_0);
axarr[1,0].imshow(reset_frame_1);
axarr[1,1].imshow(step_frame_1);
axarr[2,0].imshow(reset_frame_2);
axarr[2,1].imshow(step_frame_2);
plt.show()
It will generate the following on main:
And looks like this after the fix :
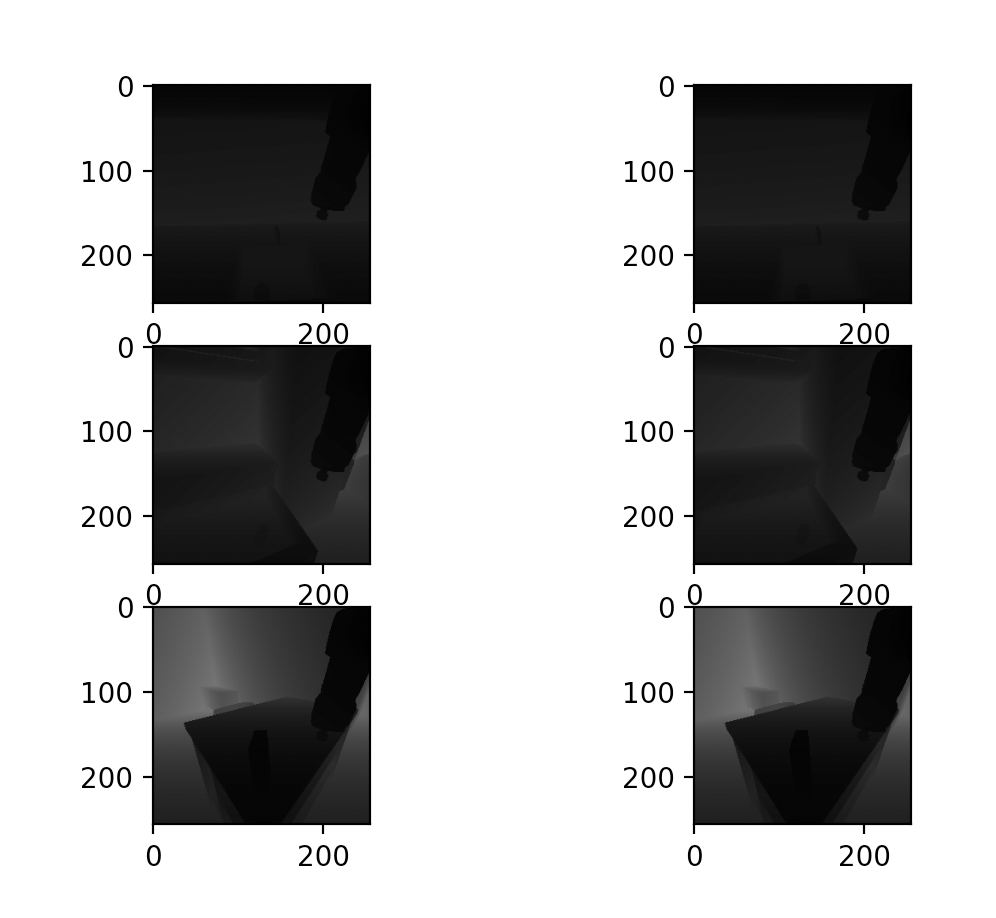
How Has This Been Tested
Types of changes
- Bug fix (non-breaking change which fixes an issue)
Checklist
- [x] My code follows the code style of this project.
- ~[ ] My change requires a change to the documentation.~
- ~[ ] I have updated the documentation accordingly.~
- [x] I have read the CONTRIBUTING document.
- [x] I have completed my CLA (see CONTRIBUTING)
- ~[ ] I have added tests to cover my changes.~
- [x] All new and existing tests passed.
We should face with the same situation for navigation tasks. With Having a separate method _get_first_observations
we need to maintain regular and _get_first_observations
methods now. Need more context and deeper review to validate the idea of the fix.
The fix is actually much simpler I think. Having a seperate call for the first observation is problematic. I think the fix is to just update the robot position here.
The fix is actually much simpler I think. Having a separate call for the first observation is problematic. I think the fix is to just update the robot position here.
I tried and that does not work because reset is overwritten in most rearrange tasks. I would need to update the robot position here as well : https://github.com/facebookresearch/habitat-lab/blob/main/habitat/tasks/rearrange/sub_tasks/pick_task.py#L282 The reason I put it in _get_observations is because it is the only method that seems to be consistently called in all rearrange tasks from the reset method.