cargo
cargo copied to clipboard
:package: A Go dependency injection container that promotes low coupling and inversion of control
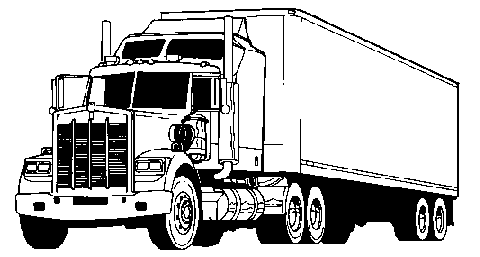
DEPRECATION NOTICE: For a better, production-ready compile-time dependency injection library, use https://github.com/google/wire.
drgomesp/cargo
An efficient and robust Go dependency injection container – by Daniel Ribeiro
Table of Contents
- Introduction
- Installation
- Getting Started
Introduction
cargo is a library that provides a powerful way of handling objects and their dependencies, by using the Container. The container works by implementing the Dependency Injection pattern via constructor injection, resulting in explicit dependencies and the achievement of the Inversion of Control principle.
Installation
$ go get github.com/drgomesp/cargo
Getting Started
Creating/Registering Services
There are two main methods used to define services in the container: container.Register
and container.Set
. The first one assumes you already have a pointer to an object instance
to work with, the second needs a definition.
Suppose you have an object:
type HttpClient struct {}
client := new(HttpClient)
To define that as a service, all you need to do is:
dic := container.NewContainer()
dic.Set("http.client", client)
From now on, whenever you need to work with the http client service, you can simply do:
if s, err := dic.Get("http.client"); err != nil {
panic(err)
}
client := s.(*HttpClient) // the type assertion is required
Or, if you do not need errors handling and panic is fine, you can get the same behavior with short synthax:
client := dic.MustGet("http.client").(*HttpClient)