gscript
gscript copied to clipboard
undefined: while
string S = "abcdefx";
string T = "def";
int myIndex(string S,string T,int pos) {
int i = pos;
int j = 0;
byte[] s = toByteArray(S);
byte[] t = toByteArray(T);
int slen = len(s);
int tlen = len(t);
while(i <= slen && j <= tlen) { //undefined: while
// for(i <= slen && j <= tlen) {
if (s[i] == s[j]) {
i += 1;
j += 1;
}else{
i = i - j + 1;
j = 0;
}
}
if (j == tlen) {
return i - tlen;
} else {
return 0;
}
}
int res = myIndex(S,T,0);
println(res);
感谢反馈,GScript
目前并没有提供 while
关键字,可直接使用 for
循环替代。
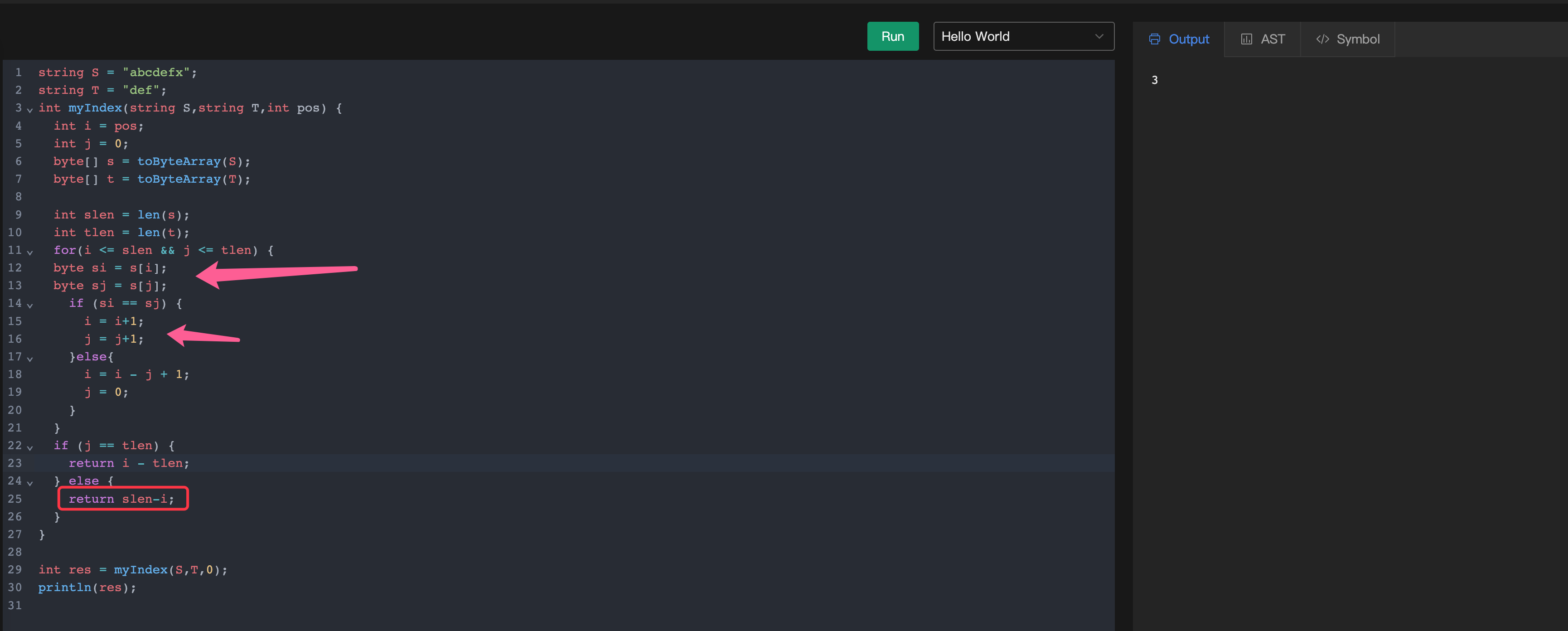
目前暂不支持 +=
语法和数组直接比较 s[i] == s[j]
,后续会逐步完善。
调整后可运行的代码:
string S = "abcdefx";
string T = "def";
int myIndex(string S,string T,int pos) {
int i = pos;
int j = 0;
byte[] s = toByteArray(S);
byte[] t = toByteArray(T);
int slen = len(s);
int tlen = len(t);
for(i <= slen && j <= tlen) {
byte si = s[i];
byte sj = s[j];
if (si == sj) {
i = i+1;
j = j+1;
}else{
i = i - j + 1;
j = 0;
}
}
if (j == tlen) {
return i - tlen;
} else {
return slen-i;
}
}
int res = myIndex(S,T,0);
println(res);
//enhancement
string str = "foo";
char[] ch = str.chars(); //转换为字符数组,可以通过index拿到单个字符
//or
string s = str.get(index);
class Strings {
string get(string str,int index) {
byte[] bs = toByteArray(str);
int idx = index;
if(idx >= len(bs)) {
return nil;
}else {
return toString(bs[idx: idx + 1]);
}
}
}
Strings s = Strings();
string foo = "foo";
string f = s.get(foo,0);
println(f); //expected f = "f";