use-between
use-between copied to clipboard
Accessing useSharedCounter within Components
To make my code cleaner I'm exporting my Component into their separate file. By doing so I'm having an issue as I can't access useSharedCounter
Should I try to pass Rather than
const useSharedCounter = () => useBetween(useCounter);
const Count = () => {
const { count } = useSharedCounter();
return <p>{count}</p>;
};
const Buttons = () => {
const { inc, dec } = useSharedCounter();
return (
<>
<button onClick={inc}>+</button>
<button onClick={dec}>-</button>
</>
);
};
const App = () => (
<>
<Count />
<Buttons />
<Count />
<Buttons />
</>
);
export default App;
I am trying to do the following But I can't see to retrieve the useSharedCounter={useSharedCounter} function in either Button.js or Count.js Is this possibel to perform such refactoring with this library?
import Button from "./Button.js'
import Count from "./Count.js'
const useSharedCounter = () => useBetween(useCounter);
const App = () => (
<>
<Count useSharedCounter={useSharedCounter} />
<Buttons useSharedCounter={useSharedCounter} />
<Count useSharedCounter={useSharedCounter} />
<Buttons useSharedCounter={useSharedCounter} />
</>
);
export default App;
Any update on this? I would truly appreciate. At this point, unless I can fix this I'm gonna probably gonna use a different library...
Hi @cdric,
You should not pass the useSharedCounter
hook through the properties of the component. This is the advantage of "use-between".
You should create a file "use-shared-counter.js" in which you will export the useSharedCounter
hook.
// ./use-shared-counter.js
import { useCallback, useState } from "react";
import { useBetween } from "use-between";
const useCounter = () => {
const [count, setCount] = useState(0);
const inc = useCallback(() => setCount((c) => c + 1), []);
const dec = useCallback(() => setCount((c) => c - 1), []);
return {
count,
inc,
dec
};
};
export const useSharedCounter = () => useBetween(useCounter);
Next, you just use the useSharedCounter
hook in any component.
// ./Buttons.js
import { useSharedCounter } from "./use-shared-counter";
export const Buttons = () => {
const { inc, dec } = useSharedCounter();
return (
<>
<button onClick={inc}>+</button>
<button onClick={dec}>-</button>
</>
);
};
// ./Count.js
import { useSharedCounter } from "./use-shared-counter";
export const Count = () => {
const { count } = useSharedCounter();
return <p>{count}</p>;
};
Enjoy!
Amazing. Thank you so much for your help. Let me test this out and I will keep you posted if I have further question!
in my code base that I inherited - there's a helper for api.
a bloated example - but gist is it's in a separate file - not in a component - I guess question is beyond these api helpers be put into a component? or continue to pass shared setters / getters via paramaeters? or is there a better way? I have a DataManager class that's a singleton - it's allowed me to get data for android / ios before - the whole redux thing is a quagmire and this mostly solves this headache apart from this edge case.
export async function reLoginUser(setShowLogin:any,setAuthenticated:any) {
const credentials = { Username: email, Password: pwd };
NocApi.post('/logon',credentials)
.then(response => {
console.log("response:",response);
if (token) {
Promise.all([
setUser(credentials),
])
.then(async (res) => {
console.log('navigation go HomeScreen');
setShowLogin(false); // HERE
setAuthenticated(true);
})
}
}
})
}
In above example I'm passing state variables around to external function - I get it's not needed when used within a component - but here can't see any other way. It's possible I could introduce a DataManager singleton -but maybe an overkilll
UPDATE:
exploring this
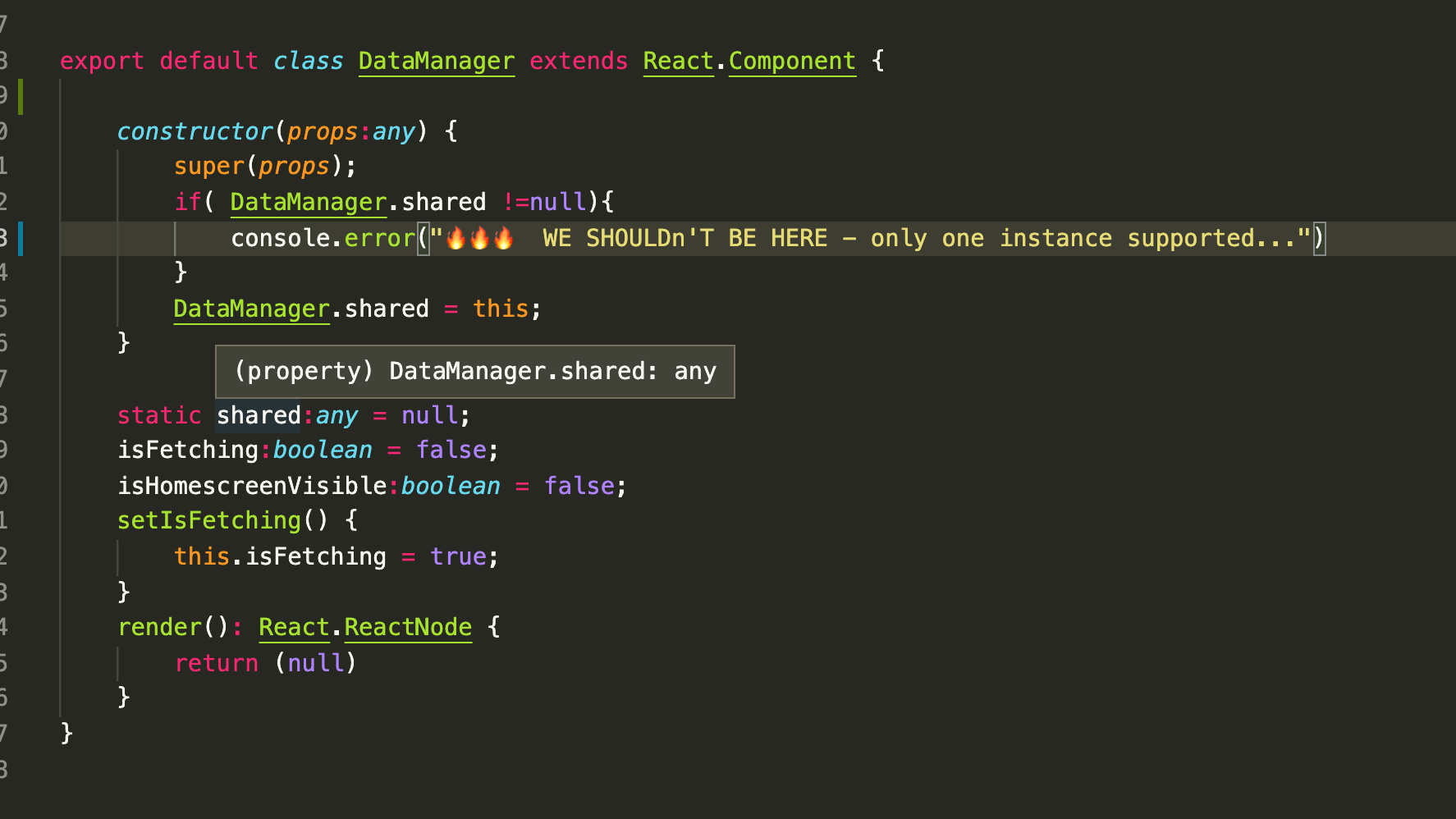
Hi @cdric,
You should not pass the
useSharedCounter
hook through the properties of the component. This is the advantage of "use-between".You should create a file "use-shared-counter.js" in which you will export the
useSharedCounter
hook. ... Enjoy!
Hello,with React 18.0, I has a problem. index.tsx
has changed to those, and the setCount
is not working, count
is always 0. What need I do to adapt 18.0?
Thank you.
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App'
import reportWebVitals from './reportWebVitals'
const root = ReactDOM.createRoot(
document.getElementById('root') as HTMLElement
)
root.render(
<React.StrictMode>
<App/>
</React.StrictMode>
)
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals()