react-super-treeview
react-super-treeview copied to clipboard
How to change icons
How can I change icon of an item ?
I wondered about the same. But the keywordValue property doesnt care what is returned when getting the value from the tree node. I made a typingsfile that says that a tree node can have an icon.
declare module 'react-super-treeview' {
import * as React from "react";
export type TreeNode = {
id: number,
name: string
children?: Array<TreeNode>
isExpanded?: boolean
isChecked?: boolean
isChildrenLoading?: boolean
icon?: JSX.Element
}
export type TreeViewProps = {
data: Array<TreeNode>
onUpdateCb?: (updatedData: Array<TreeNode>) => void
isCheckable?: (node: TreeNode, depth: number) => boolean
isDeletable?: (node: TreeNode, depth: number) => boolean
isExpandable?: (node: TreeNode, depth: number) => boolean
getStyleClassCb?: (node: TreeNode, depth: number) => string
deleteElement?: JSX.Element
depth?: number
keywordChildrenLoading?: string
keywordKey?: string
keywordLabel?: string
loadingElement?: JSX.Element
noChildrenAvailableMessage?: string
onCheckToggleCb?: (nodes: Array<TreeNode>, dept: number) => void
onDeleteCb?: (node: TreeNode, updatedData: Array<TreeNode>, depth: number) => void
onExpandToggleCb?: (node: TreeNode, depth: number) => void
transitionEnterTimeout?: number
transitionExitTimeout?: number
}
declare class SuperTreeView extends React.Component<TreeViewProps> {}
export default SuperTreeView
}
This makes it possible to do this:
import * as React from "react";
import SuperTreeview, { TreeNode } from 'react-super-treeview';
import "react-super-treeview/dist/style.css";
import {useState} from "react";
export default function TestTreeView() {
const [data, setData] = useState<Array<TreeNode>>([
{
id: 1,
name: 'Site 01',
isExpanded: true,
icon: <b>Heisann</b>,
children: [{
id: 2,
name: 'System 01',
isExpanded: true,
icon: <b>Heisann</b>,
children: [
{
id: 3,
name: 'Cage 01'
},
{
id: 4,
name: 'Cage 02'
}
]
}]
}
]);
return (
<SuperTreeview
isCheckable={() => false}
keywordLabel={"icon"}
transitionExitTimeout={0}
data={data}
onUpdateCb={setData}
/>
)
}
which renders this:
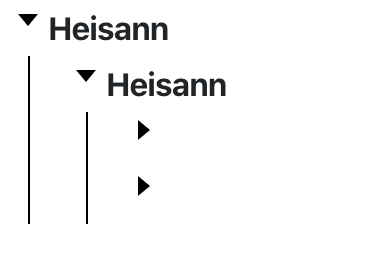
So basically you can just throw in a <FontAwesomeIcon icon={...} />
and you rock :)
long live typeless and dynamic javascript that doesn't care what you throw at it (irony).
if @azizali supports it I can open a pull request for the typingsfile. Whether or not the icon field should be on the TreeNode type or not is not that important for me. I can extend the TreeNode type and add my own fiels. Like type MyTreeNode = TreeNode & { icon?: JSX.Element }
https://github.com/azizali/react-super-treeview/pull/34