react-apollo
react-apollo copied to clipboard
Unhandled Rejection (TypeError): Cannot read property 'refetch' of undefined
Hello friends,
Today I faced an issue with GraphQL in my React.js project. What I tried to do is refetch a query once a mutation is completed. My codebase is as below
const getUserTeamQuery = useQuery(getUserTeam(usersFragment))
const data = get(getUserTeamQuery, 'data.getUserTeam')
const [updateUser] = useMutation(updateUserMutation(usersFragment), {
onCompleted: () => {
getUserTeamQuery.refetch()
}
})
const updateTeam = async () => {
updateUser({ variables: { input: { pullTeam } } })
}
Is there any solution to fix the issue?
Hi @proIT324, I just faced the same issue. It was because my component was unmount before the call of refetch
. Maybe you have a side effect caused by your mutation which unmount the component were you call your useQuery
?
I noticed the same thing while building a logout function that calles cache.reset(). Maybe this leads to an invalid state internally.
Was running into the same issue, but noticed that it only appears after a Live Reload. Works just fine after a full reload.
I am experiencing this as well in React Native when trying to refetch when the subscription client reconnects
SubscriptionClient.onReconnected(() => {
refetch()
})
This works fine when the component loads initially. However, if I navigate to the previous screen, then back to the screen with the subscription _this.currentObservable
is an empty object in
_this.obsRefetch = function (variables) {
return _this.currentObservable.query.refetch(variables);
};
thus, query is undefined.
I am experiencing the same problem. When I am loading my screen for the first time, my FlatList pull to refresh is working. After editing my screen.js file and saving it, right after that i will get this failure. I am also using chrome debug with fast refresh enabled.
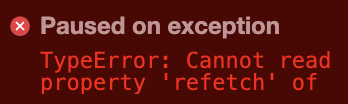

This is my FlatList:
<FlatList
data={data.users}
renderItem={({ item }) => (
<Item
id={item.id}
username={item.username}
email={item.email}
role={item.role}
// eslint-disable-next-line react/prop-types
selected={!!selected.get(item.id)}
onSelect={onSelect}
/>
)}
extraData={data}
keyExtractor={(item) => item.id}
// keyExtractor tells the list to use the ids for the react keys instead of the default key property.
refreshing={networkStatus === 4}
// As long as networkStatus is 4 (true) refetching is in progress. It has to be true, otherwise refetch will not work.
onRefresh={() => refetch()}
/>
I am having the same problem in web React (Create React App and Typescript) with Fast Refresh. If a refetch happens after a fast refreshed change, it just crashes with this error. Would it be possible to fix it? It is probably not a production issue, but it is a huge annoyance when developing an app.
Indeed, it's so annoying. I always have to completely restart the app. Please please is a fix possible?
This also happens with Hermes in React Native, every time we save a file and triggers a refresh, refetching will throw.
I am using @apollo/[email protected]
The annoying thing is, this happens to us on production as well in combination with CodePush. Whenever a new version is updated the app will restart and voila, the whole app crashes because Apollo refetch is unavailable.
I thought it could be a problem because I had declared some anon functions. I fixed that but problem is still occurring.
I currently am working around this like so:
const { data, refetch } = useQuery(...) || {}
EDIT:
This didn't actually work :(
I'm using NextJS. I got the same error after upgrade to next 9.4
, it happened after React refresh implemented by NextJS.
Use link not url in the configuration
`import { ApolloClient } from '@apollo/client';
import { InMemoryCache } from 'apollo-cache-inmemory'; import { HttpLink } from 'apollo-link-http';
const client = new ApolloClient({ cache: new InMemoryCache({ addTypename: true }), link: new HttpLink({ uri: 'http://192.168.1.39:4000' }) });
export default client;`
I stumbled upon the same problem and it looks like the culprit is the !
operator in Apollo's code:
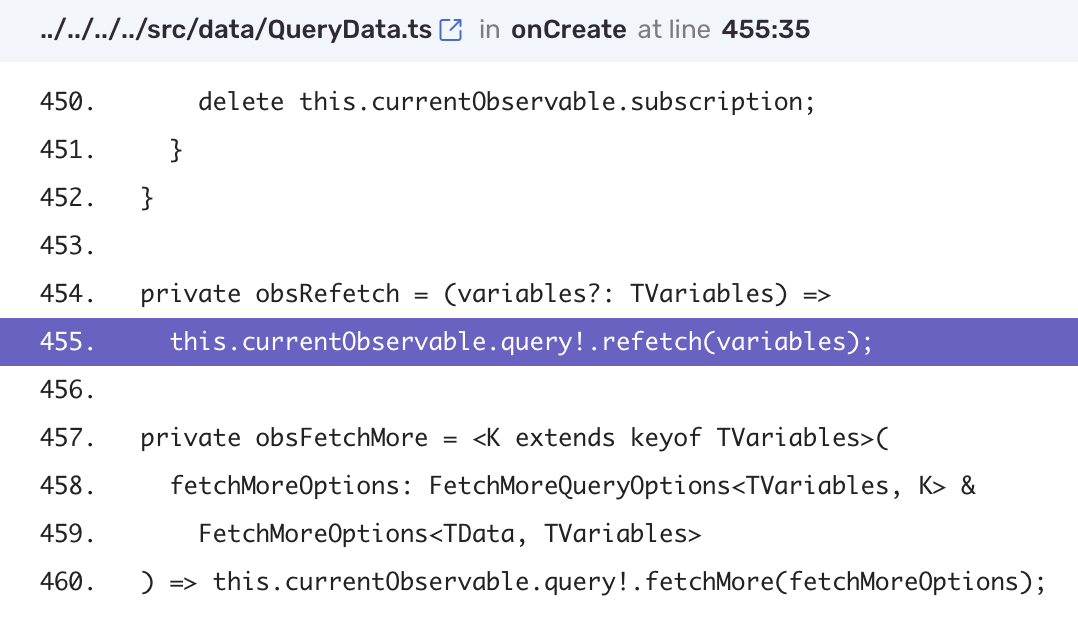
@derekstavis Is it some kind of pre-draft babel for optional chaining (?.
)?
It's typescript, @vicary
^ https://www.typescriptlang.org/docs/handbook/release-notes/typescript-2-0.html#non-null-assertion-operator
A quick fix is to wrap it in setTimout
like so:
const refetch = useCallback(() => { setTimeout(() => _refetch(), 0) }, [_refetch])
@callaars It was meant to be a joke but it pulled off poorly I guess, the use optional chaining would at least make it not crash.
A quick fix is to wrap it in
setTimout
like so:
const refetch = useCallback(() => { setTimeout(() => _refetch(), 0) }, [_refetch])
const {loading, error, data} = useQuery(VIEW_ALL_COMMENTS); const _refetch = useQuery(VIEW_ALL_COMMENTS).refetch; const refetch = useCallback(() => { setTimeout(() => _refetch(), 0) }, [_refetch]);
This code works! Thanks, maxb94!!
Same issue as others, NextJS 9.4 which implements React's native live reload (instead of hot module reload). The only solution is the one proposed by @maxb94
@wooogler a better way to do that is this
const {loading, error, data, refetch: _refetch} = useQuery(VIEW_ALL_COMMENTS);
const refetch = useCallback(() => { setTimeout(() => _refetch(), 0) }, [_refetch]);
FYI: https://github.com/apollographql/apollo-client/issues/5870
@tm1000 Thanks for taking my joke seriously, I guess. I have no idea about the inner workings, it was just a patchy-patch attitude. 😄
Is this issue being looked at or abandoned? or do we wait for v4.0 ?
^
^^