Tokamak
Tokamak copied to clipboard
Change the default background color
I only found out how to change the color scheme from dark to light (though it doesn't work), but I would like to change the color itself.
I also tried using a ZStack
like so:
struct ContentView: View {
static let inputId = "file"
var document = JSObject.global.document
var body: some View {
ZStack {
Color.blue
Text("Hello, world!")
}
}
}
but the color is not fullscreen.
I found out what you can use width: 100vw;
, but the .frame()
modifier only accepts a CGFloat
.
A flexible frame should fill the screen:
.frame(minWidth: 0, maxWidth: .infinity, minHeight: 0, maxHeight: .infinity)
It doesn't work, and/because it generates invalid property values:
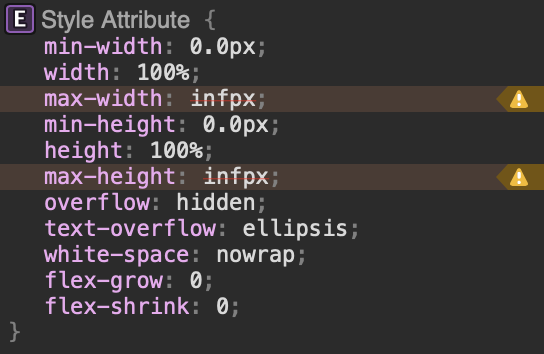
The only way I've found this to work is to set an explicit width and height in a nested VStack:
struct ContentView: View {
var body: some View {
// This ZStack will be for the main background color
ZStack(alignment: .center) {
VStack {
Text("this is a text view")
.foregroundColor(Color.white)
.background(Color.blue)
}
.frame(width: 1200, height: 500)
.background(Color(.yellow))
}
.frame(minWidth: 0, maxWidth: .infinity, minHeight: 0, maxHeight: .infinity)
.background(Color(.green))
}
}
Actually I've found another way, and this doesn't depend on defining the frame size of any subviews.
Similar to the above, except instead of the root view being a ZStack, it's HTML with a div using flexbox's centering:
struct ContentView: View {
var body: some View {
HTML("div", ["style": "display: flex; justify-content: center"]) {
Text("this is a text view")
.foregroundColor(Color.white)
.background(Color.blue)
}
.frame(minWidth: 0, maxWidth: .infinity, minHeight: 0, maxHeight: .infinity)
.background(Color(.green))
}
}
In the version, "0.5.0" this not happened. https://github.com/TokamakUI/tokamak-todo-example @MaxDesiatov @revolter