blog
blog copied to clipboard
C++ Class Aggregation
Reference: Book: Starting Out with C++ from Control Structures to Objects, byTony Gaddis, eighth edition
Concept: Aggregation occurs when a class contains an instance of another class.
Example:
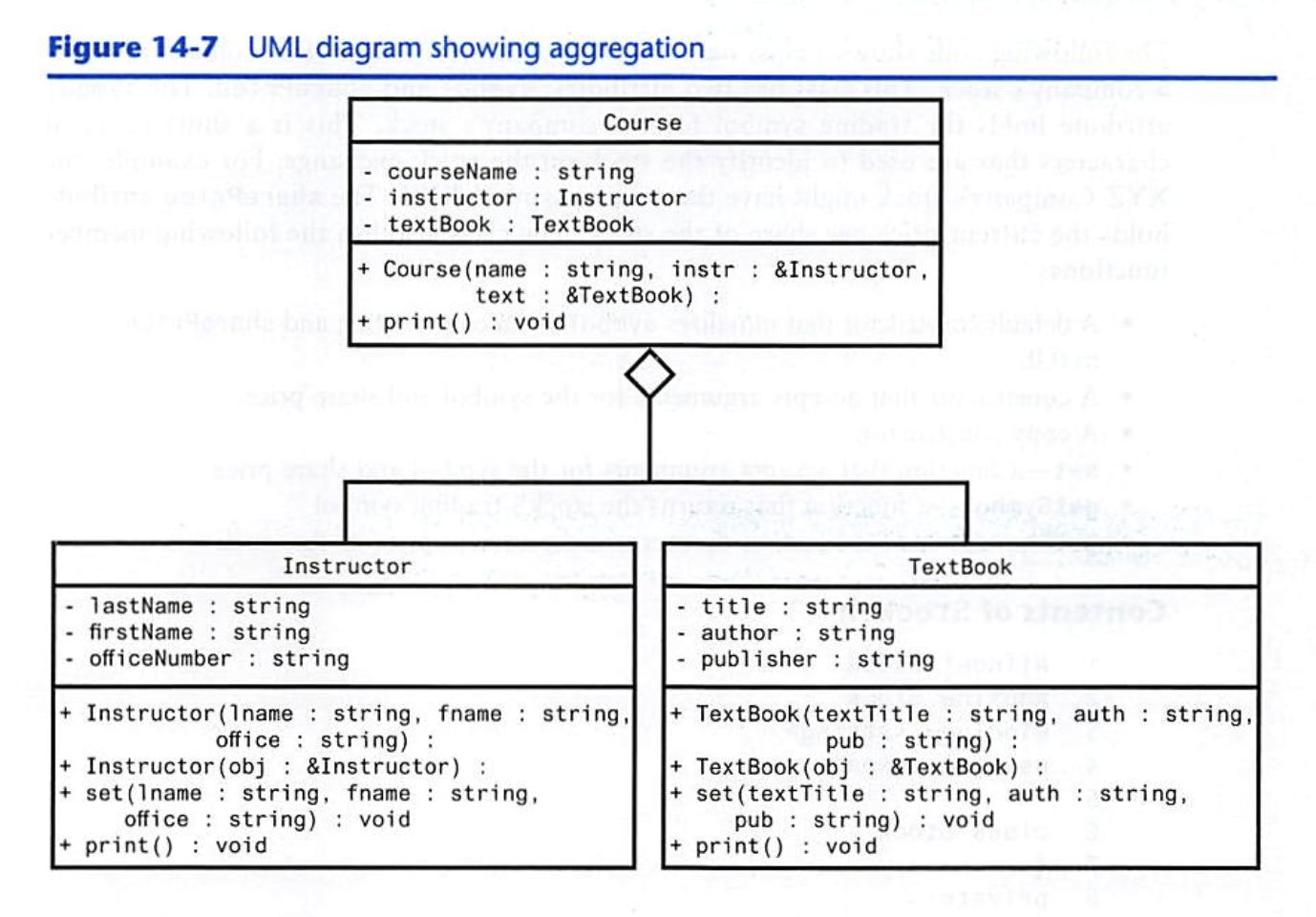
Instructor.h
#ifndef INSTRUCTOR
#define INSTRUCTOR
#include <iostream>
#include <string>
using namespace std;
// Instructor class
class Instructor
{
private:
string lastName; // Last name
string firstName; // First name
string officeNumber; // Office number
public:
// The default constructor stores empty strings
// in the string objects.
Instructor()
{ set("", "", ""); }
// Constructor
Instructor(string lname, string fname, string office)
{ set(lname, fname, office); }
// set function
void set(string lname, string fname, string office)
{ lastName = lname;
firstName = fname;
officeNumber = office; }
// print function
void print() const
{ cout << "Last name: " << lastName << endl;
cout << "First name: " << firstName << endl;
cout << "Office number: " << officeNumber << endl; }
};
#endif
TextBook.h
#ifndef TEXTBOOK
#define TEXTBOOK
#include <iostream>
#include <string>
using namespace std;
// TextBook class
class TextBook
{
private:
string title; // Book title
string author; // Author name
string publisher; // Publisher name
public:
// The default constructor stores empty strings
// in the string objects.
TextBook()
{ set("", "", ""); }
// Constructor
TextBook(string textTitle, string auth, string pub)
{ set(textTitle, auth, pub); }
// set function
void set(string textTitle, string auth, string pub)
{ title = textTitle;
author = auth;
publisher = pub; }
// print function
void print() const
{ cout << "Title: " << title << endl;
cout << "Author: " << author << endl;
cout << "Publisher: " << publisher << endl; }
};
#endif
Course.h
#ifndef COURSE
#define COURSE
#include <iostream>
#include <string>
#include "Instructor.h"
#include "TextBook.h"
using namespace std;
class Course
{
private:
string courseName; // Course name
Instructor instructor; // Instructor
TextBook textbook; // Textbook
public:
// Constructor
Course(string course, string instrLastName,
string instrFirstName, string instrOffice,
string textTitle, string author,
string publisher)
{ // Assign the course name.
courseName = course;
// Assign the instructor.
instructor.set(instrLastName, instrFirstName, instrOffice);
// Assign the textbook.
textbook.set(textTitle, author, publisher); }
// print function
void print() const
{ cout << "Course name: " << courseName << endl << endl;
cout << "Instructor Information:\n";
instructor.print();
cout << "\nTextbook Information:\n";
textbook.print();
cout << endl; }
};
#endif
The Course
class has an Instructor
object and a TextBook
object as member variables. Those objects are used as attributes of the Course
object.
Making an instance of one class an attribute of another class is called object aggregation.
The word aggregate means "a whole that is made of constituent parts."
main.cpp
// This program demonstrates the Course class.
#include "Course.h"
int main()
{
// Create a Course object.
Course myCourse("Intro to Computer Science", // Course name
"Kramer", "Shawn", "RH3010", // Instructor info
"Starting Out with C++", "Gaddis", // Textbook title and author
"Addison-Wesley"); // Textbook publisher
// Display the course info.
myCourse.print();
return 0;
}
Output:
$ g++ main.cpp -o main
$ ./main
Course name: Intro to Computer Science
Instructor Information:
Last name: Kramer
First name: Shawn
Office number: RH3010
Textbook Information:
Title: Starting Out with C++
Author: Gaddis
Publisher: Addison-Wesley