cardano-serialization-lib
cardano-serialization-lib copied to clipboard
Can't import the named export 'memory'.'buffer' (imported as 'wasm') from default-exporting module (only default export is available)
I'm having this issue whenever i try to import the package into one of my components via import * as wasm from '@emurgo/cardano-serialization-lib-browser';
I'm using create-react-app with Typescript:
"@emurgo/cardano-serialization-lib-asmjs": "^10.0.4",
"@emurgo/cardano-serialization-lib-browser": "^10.0.4",
"@types/react": "^17.0.40",
"react": "^17.0.2",
"typescript": "^4.6.2",
"web-vitals": "^2.1.4"
Hello,
you only need @emurgo/cardano-serialization-lib-browser
this is about how you would use it:
const cardanoWasm = null;
export const loadCardanoWasm = async () => {
if (cardanoWasm) {
return cardanoWasm;
}
cardanoWasm = await import('@emurgo/cardano-serialization-lib-browser/cardano_serialization_lib');
return cardanoWasm;
}
here is how to import and use that in other files:
import { loadCardanoWasm } from '../utils/loadCardanoRustModule';
const cardanoWasm = await loadCardanoWasm();
const addr = cardanoWasm.Address.from_bech32(userAddress);
@TiagoX9 pinging because I just realized the issue is 2 days old
@ozgrakkurt thank you! I will give it a try.
import { loadCardanoWasm } from '../utils/loadCardanoRustModule';
it didn't work for me. I had the same error again.
Can't import the named export 'memory'.'buffer' (imported as 'wasm') from default-exporting module (only default export is available)
@moraisandreua if you are using create-react-app you may need to override the webpack.config to make it work. There's this package react-app-rewired. I haven't try to solve this issue yet though
@TiagoX9 this one works just fine. I'm making a fork of it: https://github.com/dendorferpatrick/nami-wallet-examples/
@moraisandreua great! But only for Nami though :(
@TiagoX9 can you try something like this:
class Loader {
async load() {
if (this._wasm) return;
/**
* @private
*/
this._wasm = await import("@emurgo/cardano-serialization-lib-browser/");
}
get Cardano() {
return this._wasm;
}
}
export default new Loader();
I exactly trying this so I landed here, it comes up with the same error.
class Loader {
async load() {
if (this._wasm) return;
/**
* @private
*/
this._wasm = await import(
"@emurgo/cardano-serialization-lib-browser/"
);
}
get Cardano() {
return this._wasm;
}
}
export default new Loader();
with below in my component
import { Loader } from "../Loader";
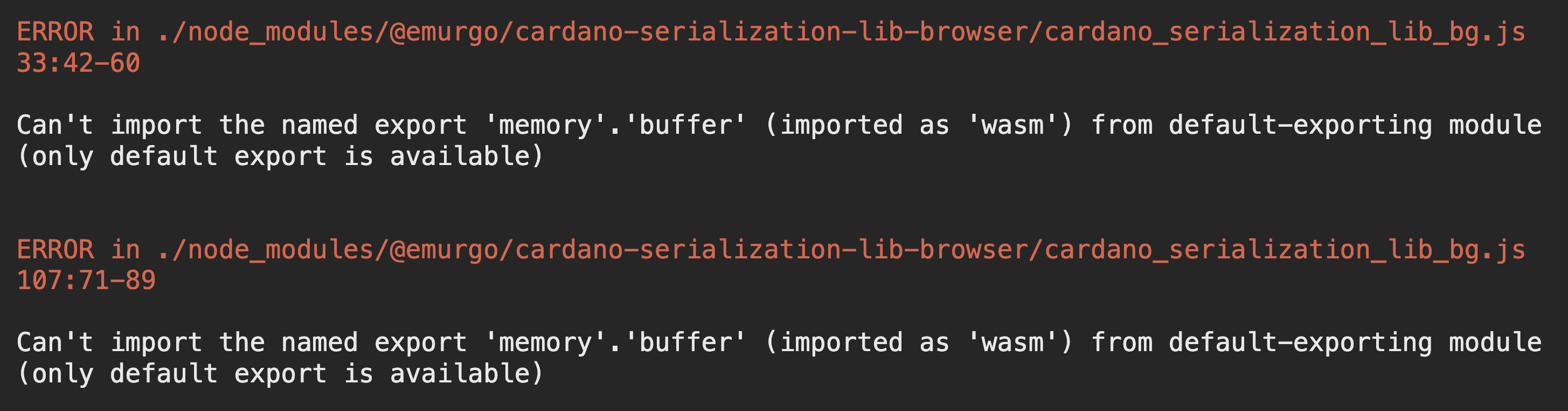
What was the solution?
I'd like to know how you solved it too.
Ive gotten WASM to work with React and Angular a few times... Craco is a great frame to run on, and supports WASM. But editing the Webpack.config is probably the way, I have the same issue and will post if I fix it
Use @emurgo/cardano-serialization-lib-asmjs package. i.e. import * as CardanoWasm from "@emurgo/cardano-serialization-lib-asmjs";
It worked for me.
https://github.com/Emurgo/cardano-serialization-lib/issues/415#issuecomment-1174788657
This worked, for whatever reason, this is the code that I have fiddled with just to get it to actually compile and function properly:
//get wallet address
walletStore.wallet.api.getUsedAddresses().then(async function (response) {
var address = response[0]
console.log(address)
let { Buffer } = require('buffer')
var bech32Address = CardanoWasm.Address.from_bytes(Buffer.from(address,"hex")).to_bech32();
console.log(bech32Address)
setUsedAddress(bech32Address);
dispatch({ type: 'cardanoStore/setLoading', payload: false });
});
This is using some version of the nami-wallet system, and I need to rip out the address from the connected wallet. That's the only reason that I needed this to work, so I can send it along with a web-request to a server -.- So frustrating.
This solution might help https://github.com/Emurgo/cardano-serialization-lib/issues/295#issuecomment-995943141
for those who still want to use CRA with CSL. Webpack configuration:
const webpack = require('webpack');
module.exports = function override(config, env) {
const wasmExtensionRegExp = /\.wasm$/;
config.resolve.extensions.push('.wasm');
config.experiments = {
asyncWebAssembly: true
};
config.resolve.fallback = {
buffer: require.resolve('buffer/'),
stream: false
}
config.module.rules.forEach((rule) => {
(rule.oneOf || []).forEach((oneOf) => {
if (oneOf.type === "asset/resource") {
oneOf.exclude.push(wasmExtensionRegExp);
}
});
});
config.plugins.push(new webpack.ProvidePlugin({
Buffer: ['buffer', 'Buffer'],
}));
config.plugins.push(new webpack.HotModuleReplacementPlugin())
return config;
}
Here an example with mesh:
https://github.com/jmagan/cra-simple-mesh