EasyAdminBundle
EasyAdminBundle copied to clipboard
[FEATURE] Column chooser
Sometimes you have too many columns/properties in entity, but some of them required by one user, some other by another one.
Let's assume we have a list of employees with many fields. By default you need to scroll page left and right to see them all.
Just add the following lines to your existing code and see the results.
use EasyCorp\Bundle\EasyAdminBundle\Provider\SessionSelectedColumnStorageProvider;
class EmployeeCrudController extends AbstractCrudController
{
public function __construct(
private SessionSelectedColumnStorageProvider $sessionSelectedColumnStorageProvider
) {}
public function configureCrud(Crud $crud): Crud
{
return $crud
->setEntityLabelInSingular('admin_label.employee.singular')
->setEntityLabelInPlural('admin_label.employee.plural')
->setupColumnChooser($this->sessionSelectedColumnStorageProvider) // <= magic is here
;
}
Now you can see new global action button on index page named "Column chooser".
It allows you choose which columns to hide/show and reorder them as you wish:
Add video:
https://user-images.githubusercontent.com/230304/204554873-ca81ac84-4360-4c0d-b14f-6f60c7a66d58.mov
Selected columns saved in user session:
Another suggested option is to save selected columns in your user object in database.
Your user entity MUST implement interface
EasyCorp\Bundle\EasyAdminBundle\Interfaces\UserParametersStorageInterface
use EasyCorp\Bundle\EasyAdminBundle\Provider\UserSelectedColumnStorageProvider;
class EmployeeCrudController extends AbstractCrudController
{
public function __construct(
private UserSelectedColumnStorageProvider $userSelectedColumnStorageProvider
) {}
public function configureCrud(Crud $crud): Crud
{
return $crud
->setEntityLabelInSingular('admin_label.employee.singular')
->setEntityLabelInPlural('admin_label.employee.plural')
->setupColumnChooser($this->userSelectedColumnStorageProvider) // <= magic is here
;
}
In my case I store parameters in related table. But your implementation may be slightly different.
By default column chooser
use all fields from index
and detail
pages.
Sometimes you want to setup it more precisely.
You may globally setup in your dashboard controller and then make customisation on controller basis.
// DashboadController.php
use EasyCorp\Bundle\EasyAdminBundle\Provider\UserSelectedColumnStorageProvider;
class DashboardController extends AbstractDashboardController
{
public function __construct(
private TranslatorInterface $translator,
private UserSelectedColumnStorageProvider $userSelectedColumnStorageProvider,
) {}
public function configureCrud(): Crud
{
return Crud::new()
->setColumnChooserSelectedColumnStorageProvider($this->userSelectedColumnStorageProvider)
->setPaginatorPageSize(10);
}
}
// EmployeeCrudController.php
class EmployeeCrudController extends AbstractCrudController
{
public function configureCrud(Crud $crud): Crud
{
return $crud
->setEntityLabelInSingular('admin_label.employee.singular')
->setEntityLabelInPlural('admin_label.employee.plural')
->enableColumnChooser() // enable it
->setColumnChooserColumns(
['imageName', 'familyName', 'givenName', 'phone', 'email'], // default
[], // get all avaiable fields from index and detail page or specify only allowed
['deletedAt', 'virtualField'] // or may just exclude some fields
)
;
}
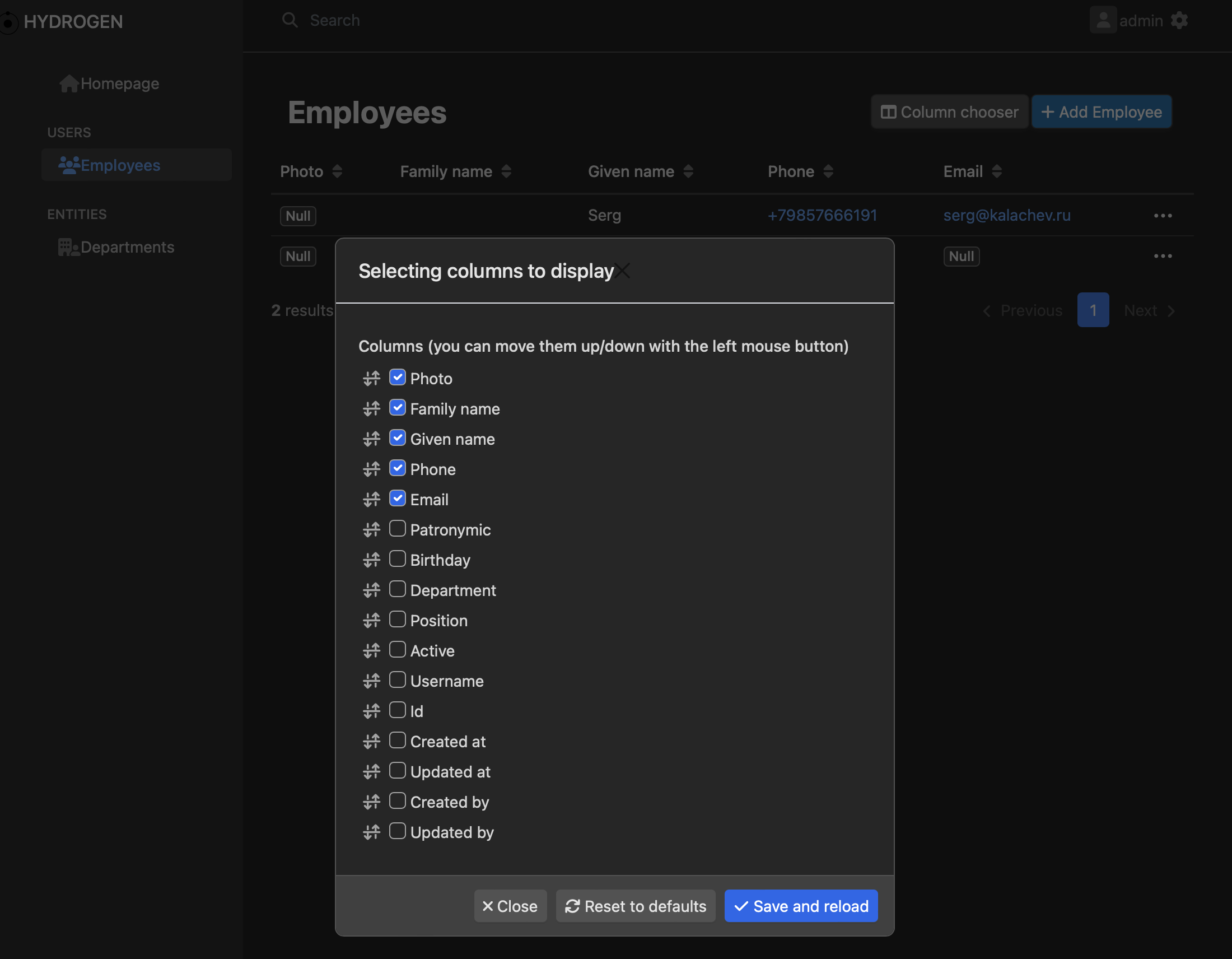