survey
survey copied to clipboard
AskOne with default set to empty string and required may still be empty
What operating system and terminal are you using? OS: MacOS 10.15.7 Terminal: zsh 5.8 (x86_64-apple-darwin19.6.0)
An example that showcases the bug.
I use survey
lib to populate a struct - it can be either pre-filled with some values already or be empty. Recently updated from v1 to v2 and found an interesting issue. In v1 Default
field used to be string
, but in v2 it is interface{}
and it causes an issue with defining defaults.
When default value is set to an empty string select interface shows correct graphics (the first item is selected by default):
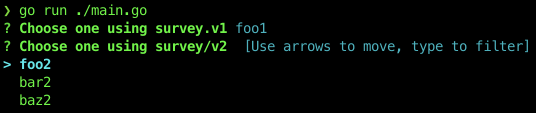
but pressing return (enter) writes an empty string to the response. If I move select down and up then it is set to the correct value. If Default
is not set at all - it works as expected - the first element that is displayed as selected is being written to the response.
package main
import (
"fmt"
survey2 "github.com/AlecAivazis/survey/v2"
"gopkg.in/AlecAivazis/survey.v1"
)
type mySetup struct {
question1 string
question2 string
question3 string
}
func main() {
var initialSetup mySetup
promptOneV1 := &survey.Select{
Message: "Choose one using survey.v1",
Options: []string{"foo1", "bar1", "baz1"},
Default: initialSetup.question1,
}
if err := survey.AskOne(promptOneV1, &initialSetup.question1, survey.Required); err != nil {
panic(err)
}
promptOneV2 := &survey2.Select{
Message: "Choose one using survey/v2",
Options: []string{"foo2", "bar2", "baz2"},
Default: initialSetup.question2,
}
if err := survey2.AskOne(promptOneV2, &initialSetup.question2, survey2.WithValidator(survey2.Required)); err != nil {
panic(err)
}
promptOneV2NoDefault := &survey2.Select{
Message: "Choose one using survey/v2 w/out default",
Options: []string{"foo3", "bar3", "baz3"},
}
if err := survey2.AskOne(promptOneV2NoDefault, &initialSetup.question3, survey2.WithValidator(survey2.Required)); err != nil {
panic(err)
}
fmt.Printf("Your choices: %s; %s; %s\n", initialSetup.question1, initialSetup.question2, initialSetup.question3)
}
What did you expect to see?
❯ go run ./main.go
? Choose one using survey.v1 foo1
? Choose one using survey/v2 foo2
? Choose one using survey/v2 w/out default foo3
Your choices: foo1; foo2; foo3
What did you see instead?
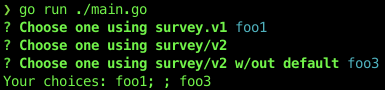